Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial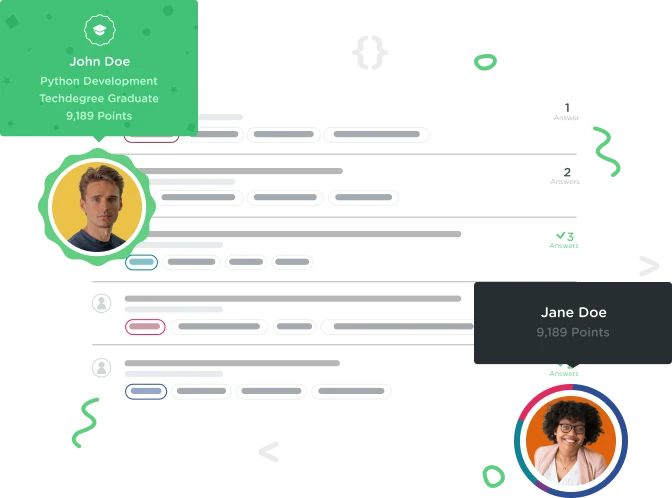

William J. Terrell
17,403 PointsEncountering several errors...
So, my code is as follows:
<?php
class Product
{
public $name = 'default name';
public $price = 0;
public $desc = 'default description';
function __construct($name, $price, $desc) {
$this->name = $name;
$this->price = $price;
$this->desc = $desc;
}
public function getInfo() {
return "Product name: " . $this->name;
}
}
$p = new Product();
$shirt = new Product("Space Juice T-Shirt", 20, "Awesome Grey T-Shirt");
$soda = new Product("Space Juice Soda", 2, "Grape Flavor Soda");
echo $soda->getInfo();
?>
and it seems to print out the correct information to the browser, but I get all these Warnings and Notices too:
Warning: Missing argument 1 for Product::__construct(), called in C:\xampp\htdocs\treehouse\Object Oriented PHP\index.php on line 27 and defined in C:\xampp\htdocs\treehouse\Object Oriented PHP\index.php on line 10
Warning: Missing argument 2 for Product::__construct(), called in C:\xampp\htdocs\treehouse\Object Oriented PHP\index.php on line 27 and defined in C:\xampp\htdocs\treehouse\Object Oriented PHP\index.php on line 10
Warning: Missing argument 3 for Product::__construct(), called in C:\xampp\htdocs\treehouse\Object Oriented PHP\index.php on line 27 and defined in C:\xampp\htdocs\treehouse\Object Oriented PHP\index.php on line 10
Notice: Undefined variable: name in C:\xampp\htdocs\treehouse\Object Oriented PHP\index.php on line 12
Notice: Undefined variable: price in C:\xampp\htdocs\treehouse\Object Oriented PHP\index.php on line 13
Notice: Undefined variable: desc in C:\xampp\htdocs\treehouse\Object Oriented PHP\index.php on line 14
Product name: Space Juice Soda
Any idea what is going on?
Thanks!
3 Answers
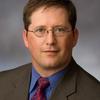
Ted Sumner
Courses Plus Student 17,967 PointsYour issue is that you have $p = new Product();
with no arguments. If you comment out that line your errors go away.
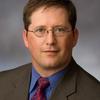
Ted Sumner
Courses Plus Student 17,967 PointsAs another note, you can change your getInfo() function to this:
<?php
return "Product name: $this->name";
Variables inside double quotes are resolved prior to the echo. Variables inside single quotes are not resolved, so the variable name would display.

William J. Terrell
17,403 PointsThanks!
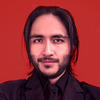
Carlos Rojas "Yoru No Tori"
6,530 PointsI was wondering the same issue, thanks
William J. Terrell
17,403 PointsWilliam J. Terrell
17,403 PointsAlways just a tiny little something, isn't it? :)
Thanks!
Ted Sumner
Courses Plus Student 17,967 PointsTed Sumner
Courses Plus Student 17,967 PointsThey did a poor job with this in the video. If you watch carefully, after he previews the code without errors the offending line is gone from his workspace but he never says to delete it.