Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial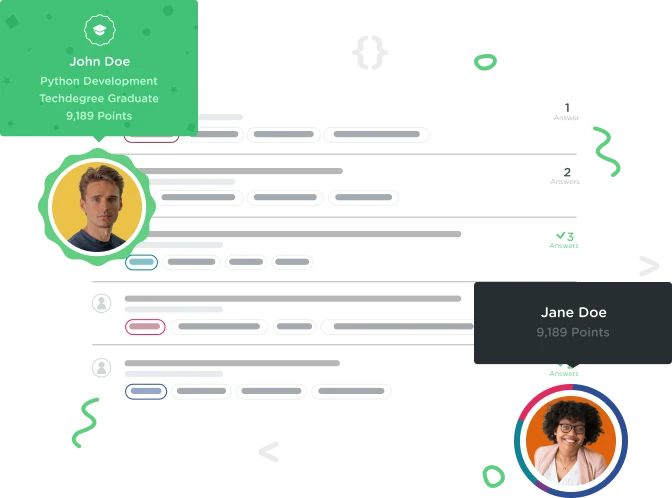
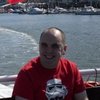
Sean Flanagan
33,235 Points"End of file" error
Hi. I'm not sure what I've done but I get an error message when trying to run my program:
using System; //Using directive: avoids repeating "System".
namespace Treehouse.FitnessFrog
{
class Program
{
static void Main()
{
run runningTotal = 0.0;
while (true) {
// Prompt the user for minutes exercised
Console.Write("Enter how many minutes you exercised or type \"quit\" to exit: "); //Print text to screen, keeps cursor at end of line after colon.
// Write: method name.
// Console: name of class that contains method.
// System: namespace that contains class.
// Namespace.ClassName.MethodName(parameterType parameterName);
var entry = Console.ReadLine(); //ReadLine reads one line at a time from the console.
if (entry.ToLower() == "quit")
{
break;
}
try
{
var minutes = double.Parse(entry);
if (minutes <= 0)
{
Console.WriteLine(minutes + " is not an acceptable value.");
continue;
}
else if (minutes <= 10)
{
Console.WriteLine("Better than nothing!");
}
else if (minutes <= 30)
{
Console.WriteLine("Way to go!");
}
else if (minutes <= 60)
{
Console.WriteLine("You must be a ninja in training!");
}
else
{
Console.WriteLine("Okay, now you're just showing off!");
}
runningTotal += minutes;
}
catch(FormatException)
{
Console.WriteLine("That is not valid input.");
continue;
}
// Add minutes exercised to total.
// Display total minutes exercised on-screen.
//WriteLine writes one line at a time to the console.
Console.WriteLine("You've entered " + runningTotal + " minutes."); //Plus operator concatenates strings.
// Repeat until user quits.
Console.WriteLine("Goodbye");
}
}
}
Error:
Program.cs(68,246): error CS1525: Unexpected symbol `end-of-file'
Compilation failed: 1 error(s), 0 warnings
Here's a snapshot I've taken: https://w.trhou.se/seepakgqxl
Thanks in advance for any help.
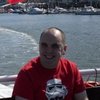
Sean Flanagan
33,235 PointsHi Nipi and thanks for your input. I can see I'm one } short and that it's the while (true)
one but I don't know where it's supposed to go. It's just that in the video, Jeremy cut and pasted a large chunk of code and that confused me. I was trying to work out which code he pasted and where. I think it would help if he posted the code in the Teacher's Notes.
2 Answers
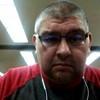
micram1001
33,833 PointsYour bracket should line up. If statement { }, while statement {}, etc.
using System; //Using directive: avoids repeating "System".
namespace Treehouse.FitnessFrog
{
class Program
{
static void Main()
{
var runningTotal = 0.0;
while (true) {
// Prompt the user for minutes exercised
Console.Write("Enter how many minutes you exercised or type \"quit\" to exit: ");
//Print text to screen, keeps cursor at end of line after colon.
// Write: method name.
// Console: name of class that contains method.
// System: namespace that contains class.
// Namespace.ClassName.MethodName(parameterType parameterName);
var entry = Console.ReadLine(); //ReadLine reads one line at a time from the console.
if (entry.ToLower() == "quit")
{
break;
}
try
{
var minutes = double.Parse(entry);
if (minutes <= 0)
{
Console.WriteLine(minutes + " is not an acceptable value.");
continue;
}
else if (minutes <= 10)
{
Console.WriteLine("Better than nothing!");
}
else if (minutes <= 30)
{
Console.WriteLine("Way to go!");
}
else if (minutes <= 60)
{
Console.WriteLine("You must be a ninja in training!");
}
else
{
Console.WriteLine("Okay, now you're just showing off!");
}
runningTotal += minutes;
}
catch(FormatException)
{
Console.WriteLine("That is not valid input.");
continue;
}
// Add minutes exercised to total.
// Display total minutes exercised on-screen.
//WriteLine writes one line at a time to the console.
Console.WriteLine("You've entered " + runningTotal + " minutes.");
//Plus operator concatenates strings.
// Repeat until user quits.
Console.WriteLine("Goodbye");
}
}
}
}
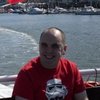
Sean Flanagan
33,235 PointsHi Michael. Thanks for your help. I added a closing brace under Console.WriteLine("Goodbye");
.
I can show you my Program as it is now:
using System; //Using directive: avoids repeating "System".
namespace Treehouse.FitnessFrog
{
class Program
{
static void Main()
{
run runningTotal = 0.0;
while (true)
{
// Prompt the user for minutes exercised
Console.Write("Enter how many minutes you exercised or type \"quit\" to exit: "); //Print text to screen, keeps cursor at end of line after colon.
// Write: method name.
// Console: name of class that contains method.
// System: namespace that contains class.
// Namespace.ClassName.MethodName(parameterType parameterName);
var entry = Console.ReadLine(); //ReadLine reads one line at a time from the console.
if (entry.ToLower() == "quit")
{
break;
}
try
{
var minutes = double.Parse(entry);
if (minutes <= 0)
{
Console.WriteLine(minutes + " is not an acceptable value.");
continue;
}
else if (minutes <= 10)
{
Console.WriteLine("Better than nothing!");
}
else if (minutes <= 30)
{
Console.WriteLine("Way to go!");
}
else if (minutes <= 60)
{
Console.WriteLine("You must be a ninja in training!");
}
else
{
Console.WriteLine("Okay, now you're just showing off!");
}
runningTotal += minutes;
}
catch(FormatException)
{
Console.WriteLine("That is not valid input.");
continue;
}
// Add minutes exercised to total.
// Display total minutes exercised on-screen.
//WriteLine writes one line at a time to the console.
Console.WriteLine("You've entered " + runningTotal + " minutes."); //Plus operator concatenates strings.
// Repeat until user quits.
Console.WriteLine("Goodbye");
}
}
}
}
I typed in mcs Program.cs
in the console and I got:
Program.cs(9,9): error CS0246: The type or namespace name `run' could not be found. Are you m
issing an assembly reference?
Program.cs(50,11): error CS0841: A local variable `runningTotal' cannot be used before it is
declared
Program.cs(64,49): error CS0841: A local variable `runningTotal' cannot be used before it is
declared
I then typed in mono Program.exe
and yet the program worked fine. I'm a bit miffed about the error messages that arose from my mcs Program.cs
command.
Thanks. :)
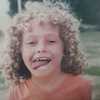
Hugo da Silva Ribas
7,666 PointsHello there,
Maybe i don't know much about C# syntax but,
Why were you declaring a variable with 'run' instead of 'var'?
The program doesn't seems to understand. The error explicitly says so.
Cya
Nipi Tiri
2,988 PointsNipi Tiri
2,988 PointsMaybe you miss one ending bracket? //Edit, i see one ending bracket missing, if you start reading outside then, while loop hasnt ending bracket.