Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial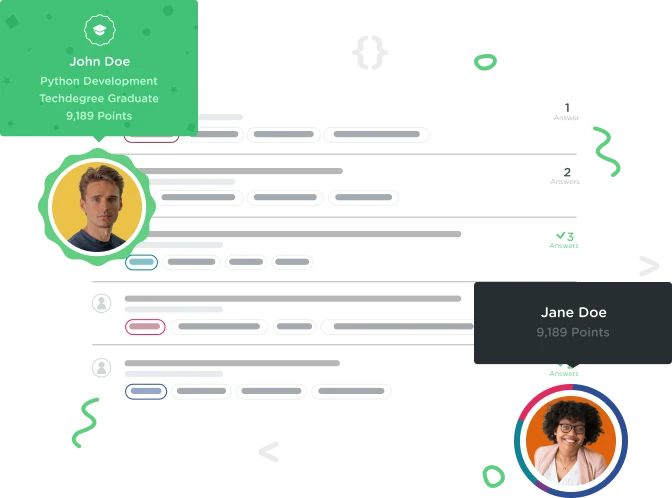

Thomas Newton
Courses Plus Student 2,442 PointsEnsure towers can't be placed on paths?
Part way through, the instructor simply assumes I would already know how to code this. However, I cannot for the life of me recall the method to do so. I am sure it involves either the Map Location or Path Location methods, but some guidance would be appreciated.
UPDATE: It seems I need to clarify - I would appreciate some reference code that would help me solve this issue. Verbal feedback is useful, but I learn much better by visual example than by text. Thanks for your time.
5 Answers

Gavin Ralston
28,770 PointsThis was mentioned around 1:00 in the video as an exercise for you to complete on your own if you want. MapLocation has code you can use for validation, and it's very similar to what you could implement in the Tower class to confirm if it's designated point is on the path
class MapLocation : Point
{
public MapLocation(int x, int y, Map map) : base(x, y)
{
// here's your validation code...
if (!map.OnMap(this))
{
throw new OutOfBoundsException(x + "," + y + " is outside the boundaries of the map.");
}
}
}
So the constructor for the MapLocation will throw an exception if the point isn't on the Map, which has the OnMap() method.
public bool OnMap(Point point)
{
return point.X >= 0 && point.X < Width &&
point.Y >= 0 && point.Y < Height;
}
So I guess one way to approach it would be to write something very similar in the Path class. Something like:
public bool OnPath(Point point) {
for (int i = 0; i < _path.Length; i++) {
if (i.X = point.X && i.Y == point.Y) return true;
}
return false;
}
...and use that to check for a valid starting position in the Tower constructor, like
if (!path.onPath(towerPosition))
{
// place tower
}
else
{
// do whatever you do when you don't get a valid start position
}
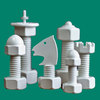
Steven Parker
231,275 PointsThe basic idea would be to loop through the path locations, checking to see if any of them match the location where the tower is being placed. If a conflict is detected, you could return null or throw an exception, depending on how you want to handle that situation in the rest of the code.
As mentioned in the video, there's an example of this kind of validation shown in the creation of the MapLocation class.

Thomas Newton
Courses Plus Student 2,442 PointsOkay, but where was that even mentioned? I just looked through all the videos and couldn't find any mention of it. I'm even looking at my MapLocation class and the MapLocation.cs file, and I'm not seeing what I'm supposed to do. So I have no reference or guides for this, and I don't feel like I can continue the course unless I have this figured out.
I honestly think it's pretty ballsy of the tutors to simply assume we'd remember these details, especially since I can never remember any of the methods to do this. Where would I even put this loop? The tower class? The path class? I just feel like I've hit a brick wall...

hankamamut
3,177 PointsWill anybody be nice enough and post a solution that compiles? Thanks.

ALEX M
2,233 PointsI'm not sure if this is the most optimal solution, but the issue seems to be that if we compare path[i] to a point the result always returns false. I resolved this with the code below where I'm comparing X to X and Y to Y. I used a bool variable x to debug and track when the "if" reads as "true" and I'm passing in a point to compare against, this way you can check whether any point is onPath.
public bool onPath(Point point)
{ bool x = false;
for (int i = 0; i < _path.Length; i++)
{
if (_path[i].X == point.X && _path[i].Y == point.Y) { x = true; break; }
else { x = false; }
}
return x;
}

Sergey Ivanov
Courses Plus Student 1,053 PointsI think this method should be in Path class.
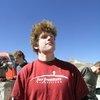
Geoffrey Neal
30,298 PointsSteven, I had the same basic idea but it isn't working.
public class Tower
{
private readonly MapLocation _location;
private Path _path;
public Tower(MapLocation location, Path path)
{
_location = location;
_path = path;
}
public bool IsOnPath()
{
for (int i = 0; i < _path.Length; i++)
{
if (_path[i] == _location) return true;
}
return false;
}
}
The problem seems to be that _path is a Path object and no longer an Array of MapLocations, so I can't reference each MapLocation in _path with [i] (this is my best guess as to the problem). I gives the error message "Cannot apply indexing with [] to an expression of type 'Path' ". Any ideas how to overcome this?
Gavin I think you're trying to do something similar to me but I don't understand how you can have i.X and i.Y when i is of type int? Surely i will just be the number of times the loop has occurred? I am using it as the index for each item in _path (but I am obviously still having problems).