Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial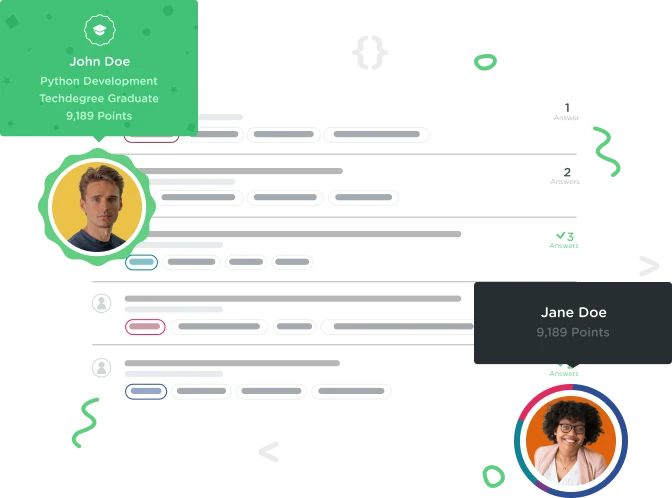

Larry Singleton
18,946 PointsEntity Framework
Question Link: https://teamtreehouse.com/library/entity-framework-basics/crud-operations/deleting-an-entity
Question:
In Repository.cs update the DeleteCourse method to delete a course from the database.
Instantiate an instance of the Context class within a using statement
Create a "stub" course entity and set its Id property value to the passed in id parameter value
Retrieve the course's entry using the context's Entry method and set its state to "Deleted"
Call the context's SaveChanges method to persist the changes to the database
Provided Code:
using System.Collections.Generic;
using System.Data.Entity;
using System.Linq;
namespace Treehouse.CodeChallenges
{
public static class Repository
{
public static List<Course> GetCourses()
{
using (var context = new Context())
{
return context.Courses
.OrderBy(c => c.Teacher.LastName)
.ThenBy(c => c.Teacher.FirstName)
.ToList();
}
}
public static List<Course> GetCoursesByTeacher(string lastName)
{
using (var context = new Context())
{
return context.Courses
.Where(c => c.Teacher.LastName == lastName)
.ToList();
}
}
public static Course GetCourse(int id)
{
using (var context = new Context())
{
return context.Courses
.Include(c => c.Teacher)
.SingleOrDefault(c => c.Id == id);
}
}
public static void AddCourse(Course course)
{
using (var context = new Context())
{
context.Courses.Add(course);
context.SaveChanges();
}
}
public static void UpdateCourse(Course course)
{
using (var context = new Context())
{
context.Courses.Attach(course);
context.Entry(course).State = EntityState.Modified;
context.SaveChanges();
}
}
public static void DeleteCourse(int id)
{
// TODO
}
}
}
My Last Attempt:
using System.Collections.Generic;
using System.Data.Entity;
using System.Linq;
namespace Treehouse.CodeChallenges
{
public static class Repository
{
public static List<Course> GetCourses()
{
using (var context = new Context())
{
return context.Courses
.OrderBy(c => c.Teacher.LastName)
.ThenBy(c => c.Teacher.FirstName)
.ToList();
}
}
public static List<Course> GetCoursesByTeacher(string lastName)
{
using (var context = new Context())
{
return context.Courses
.Where(c => c.Teacher.LastName == lastName)
.ToList();
}
}
public static Course GetCourse(int id)
{
using (var context = new Context())
{
return context.Courses
.Include(c => c.Teacher)
.SingleOrDefault(c => c.Id == id);
}
}
public static void AddCourse(Course course)
{
using (var context = new Context())
{
context.Courses.Add(course);
context.SaveChanges();
}
}
public static void UpdateCourse(Course course)
{
using (var context = new Context())
{
context.Courses.Attach(course);
context.Entry(course).State = EntityState.Modified;
context.SaveChanges();
}
}
public static void DeleteCourse(int id)
{
using (Context context = GetContext())
var stub = new stub() { Id = stubId };
context.Entry(stub).State = EntityState.Deleted;
context.SaveChanges();
}
}
}
Error Provided:
Repository.cs(62,54): error CS1023: An embedded statement may not be a declaration or labeled statement
Compilation failed: 1 error(s), 0 warnings
Not sure what I'm doing wrong...
using System.Collections.Generic;
using System.Data.Entity;
using System.Linq;
namespace Treehouse.CodeChallenges
{
public static class Repository
{
public static List<Course> GetCourses()
{
using (var context = new Context())
{
return context.Courses
.OrderBy(c => c.Teacher.LastName)
.ThenBy(c => c.Teacher.FirstName)
.ToList();
}
}
public static List<Course> GetCoursesByTeacher(string lastName)
{
using (var context = new Context())
{
return context.Courses
.Where(c => c.Teacher.LastName == lastName)
.ToList();
}
}
public static Course GetCourse(int id)
{
using (var context = new Context())
{
return context.Courses
.Include(c => c.Teacher)
.SingleOrDefault(c => c.Id == id);
}
}
public static void AddCourse(Course course)
{
using (var context = new Context())
{
context.Courses.Add(course);
context.SaveChanges();
}
}
public static void UpdateCourse(Course course)
{
using (var context = new Context())
{
context.Courses.Attach(course);
context.Entry(course).State = EntityState.Modified;
context.SaveChanges();
}
}
public static void DeleteCourse(int id)
{
using (Context context = GetContext())
var stub = new stub() { Id = stubId };
context.Entry(stub).State = EntityState.Deleted;
context.SaveChanges();
}
}
}
4 Answers
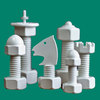
Steven Parker
231,268 PointsI spot a few issues:
- no GetContext method has been defined
- take a look at the other methods for examples of the using statement syntax
- you forgot to enclose the code block after the using in braces
- there's no "stub" type, your stub needs to be a
new Course()
. - the passed-in argument is just "id" (not "stubId")
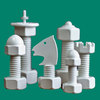
Steven Parker
231,268 PointsYou got off track a bit here:
var course = context.Courses.Where(c => c.Id == id);
All you needed in your original was to change the object type from "Stub" to "Course", and use the passed-in argument:
var stub = new Course() { Id = id };
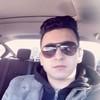
Oualid Jniyah
21,607 Pointschange stub to course!
public static void DeleteCourse(int id) { using (var context = new Context() ) { var course = new Course() { Id = id }; context.Entry(course).State = EntityState.Deleted; context.SaveChanges(); } }

Larry Singleton
18,946 PointsI now have this:
using System.Collections.Generic;
using System.Data.Entity;
using System.Linq;
namespace Treehouse.CodeChallenges
{
public static class Repository
{
public static List<Course> GetCourses()
{
using (var context = new Context())
{
return context.Courses
.OrderBy(c => c.Teacher.LastName)
.ThenBy(c => c.Teacher.FirstName)
.ToList();
}
}
public static List<Course> GetCoursesByTeacher(string lastName)
{
using (var context = new Context())
{
return context.Courses
.Where(c => c.Teacher.LastName == lastName)
.ToList();
}
}
public static Course GetCourse(int id)
{
using (var context = new Context())
{
return context.Courses
.Include(c => c.Teacher)
.SingleOrDefault(c => c.Id == id);
}
}
public static void AddCourse(Course course)
{
using (var context = new Context())
{
context.Courses.Add(course);
context.SaveChanges();
}
}
public static void UpdateCourse(Course course)
{
using (var context = new Context())
{
context.Courses.Attach(course);
context.Entry(course).State = EntityState.Modified;
context.SaveChanges();
}
}
public static void DeleteCourse(int id)
{
using (var context = new Context() ){
var course = context.Courses.Where(c => c.Id == id);
context.Entry(course).State = EntityState.Deleted;
context.SaveChanges();
}
}
}
}
am now getting this:
Bummer! Did you create a stub course entity using the passed in course 'id' parameter value and then pass it into the context 'Entry' method call?
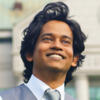
Tanmoy Chowdhury
12,622 Pointsusing System.Collections.Generic;
using System.Data.Entity;
using System.Linq;
namespace Treehouse.CodeChallenges
{
public static class Repository
{
public static List<Course> GetCourses()
{
using (var context = new Context())
{
return context.Courses
.OrderBy(c => c.Teacher.LastName)
.ThenBy(c => c.Teacher.FirstName)
.ToList();
}
}
public static List<Course> GetCoursesByTeacher(string lastName)
{
using (var context = new Context())
{
return context.Courses
.Where(c => c.Teacher.LastName == lastName)
.ToList();
}
}
public static Course GetCourse(int id)
{
using (var context = new Context())
{
return context.Courses
.Include(c => c.Teacher)
.SingleOrDefault(c => c.Id == id);
}
}
public static void AddCourse(Course course)
{
using (var context = new Context())
{
context.Courses.Add(course);
context.SaveChanges();
}
}
public static void UpdateCourse(Course course)
{
using (var context = new Context())
{
context.Courses.Attach(course);
context.Entry(course).State = EntityState.Modified;
context.SaveChanges();
}
}
public static void DeleteCourse(int id)
{
using (var context = new Context())
{
var stub = new Course() { Id = id };
context.Entry(stub).State = EntityState.Deleted;
//var course = context.Courses.Where(c => c.Id == id);
//context.Entry(course).State = EntityState.Deleted;
context.SaveChanges();
}
}
}
}