Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial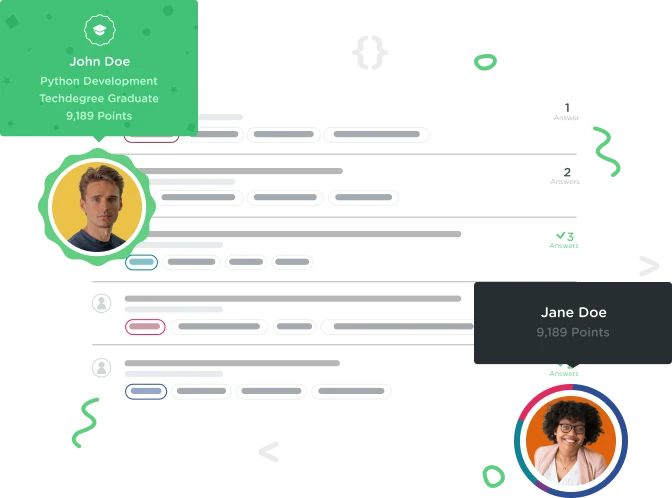

Larry Singleton
18,946 PointsEntity Frameworks Basics
Question:
In Teacher.cs add the following to the Teacher class:
A using directive for the System.Collections.Generic namespace
A navigation collection property named Courses of type ICollection<Course>
A default constructor that initializes the Courses property to an instance of List<Course>
***
courses.cs
namespace Treehouse.CodeChallenges
{
public class Course
{
public int Id { get; set; }
public string Title { get; set; }
public string Description { get; set; }
public int Length { get; set; }
public int TeacherId { get; set; } // foreign key property
public Teacher Teacher { get; set; } // navigation property
}
}
teacher.cs
using System.Collections.Generic; // 1st objective
namespace Treehouse.CodeChallenges
{
public class Teacher
{
public Courses() //3rd objective???
{
Courses = new List<Course>();
}
public int Id { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public ICollection<Courses> Courses { get; set; } // 2nd objective
}
}
error
Teacher.cs(6,23): error CS1520: Class, struct, or interface method must have a return type
Compilation failed: 1 error(s), 0 warnings
What am I missing?
namespace Treehouse.CodeChallenges
{
public class Course
{
public int Id { get; set; }
public string Title { get; set; }
public string Description { get; set; }
public int Length { get; set; }
public int TeacherId { get; set; } // foreign key property
public Teacher Teacher { get; set; } // navigation property
}
}
using System.Collections.Generic; // 1st objective
namespace Treehouse.CodeChallenges
{
public class Teacher
{
public Courses() //3rd objective???
{
Courses = new List<Course>();
}
public int Id { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public ICollection<Courses> Courses { get; set; } // 2nd objective
}
}
4 Answers
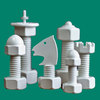
Steven Parker
231,268 PointsI see two issues:
- Courses should be "type ICollection<Course>" (singular) but you wrote "ICollection<Courses>" (plural)
- A constructor must have the same name as its class, but you named yours "Courses" instead of "Teacher"

Larry Singleton
18,946 PointsIt worked, thanks so much!

Mihai Leonardo Cosmin
3,828 Pointsusing System.Collections.Generic; //Step 1 namespace Treehouse.CodeChallenges { public class Teacher { public Teacher() { Courses = new List<Course>(); // Step 3 }
public int Id { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public ICollection<Course> Courses{get;set;} // Step 2
}
}

Mihai Leonardo Cosmin
3,828 Pointsusing System.Collections.Generic; //Step 1 namespace Treehouse.CodeChallenges { public class Teacher { public Teacher() { Courses = new List<Course>(); // Step 3 }
public int Id { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public ICollection<Course> Courses{get;set;} // Step 2
}
}
This is Correct
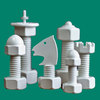
Steven Parker
231,268 PointsDid you mean to post it two times? (You can delete the extra one).
Larry Singleton
18,946 PointsLarry Singleton
18,946 PointsI don't have a clue, guidance please...