Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial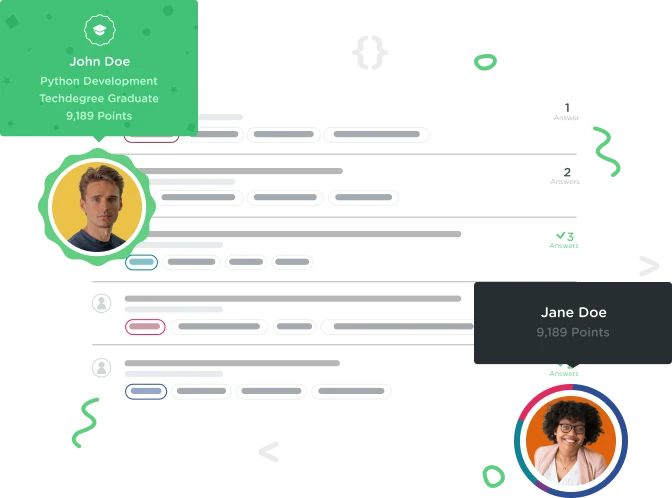

s t
2,699 Pointsenum method objective
My code passed in the Treehouse compiler but has errors in xcode:
// Example of UIBarButtonItem instance // let someButton = UIBarButtonItem(title: "A Title", style: .plain, target: nil, action: nil)
enum BarButton { case done(title: String) case edit(title: String)
func button() -> UIBarButtonItem { switch self { case .done(let title): return UIBarButtonItem(title: "title", style: .done, target: nil, action: nil) case .edit(let title): return UIBarButtonItem(title: "title", style: .plain, target: nil, action: nil) } } } let done = BarButton.done(title: "Save") let edit = BarButton.edit(title: "Save")
The error message I am getting is " Type UIBarButtonItemStyle has no member 'done'" and " Type UIBarButtonItemStyle has no member 'plain' "
Does anyone know why?
4 Answers
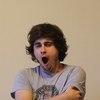
Arman Arutyunov
21,900 Points// Example of UIBarButtonItem instance
// let someButton = UIBarButtonItem(title: "A Title", style: .plain, target: nil, action: nil)
enum BarButton {
case done(title: String)
case edit(title: String)
func button() -> UIBarButtonItem {
switch self {
case .done(let title):
return UIBarButtonItem(title: title, style: .done, target: nil, action: nil)
case .edit(let title):
return UIBarButtonItem(title: title, style: .plain, target: nil, action: nil)
}
}
}
let done = BarButton.done(title: "Save")
let button = done.button()
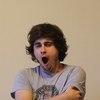
Arman Arutyunov
21,900 PointsI've put your code to the playground and there are actually no errors. Just a couple of warnings saying you declared variables that were never used. Compiler is talking about this
let title
inside
case .done(let title):
and
case .edit(let title):
Let's deal with it by actually using those constants inside the case return so you don't hardcode the value of a button title but put there anything declared in the process of instantiating.
The full snippet:
let someButton = UIBarButtonItem(title: "A Title", style: .plain, target: nil, action: nil)
enum BarButton {
case done(title: String)
case edit(title: String)
func button() -> UIBarButtonItem {
switch self {
case .done(let title):
return UIBarButtonItem(title: title, style: .done, target: nil, action: nil)
case .edit(let title):
return UIBarButtonItem(title: title, style: .plain, target: nil, action: nil)
}
}
}
let done = BarButton.done(title: "Save")
let edit = BarButton.edit(title: "Save")
Feel free to ask if there are any more questions

s t
2,699 PointsThanks but actually I was mistaken, I still did not pass the objective with just this code. What am I missing?
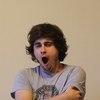
Arman Arutyunov
21,900 PointsAnd hey, I'm trying to help you. Minusing my first answer wasn't really necessary
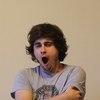
Arman Arutyunov
21,900 PointsThat's really odd. But try calling .done and .plain through UIBarButtonItemStyle like this
case .done(let title):
return UIBarButtonItem(title: title, style: UIBarButtonItemStyle.done, target: nil, action: nil)
case .edit(let title):
return UIBarButtonItem(title: title, style: UIBarButtonItemStyle.plain, target: nil, action: nil)