Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial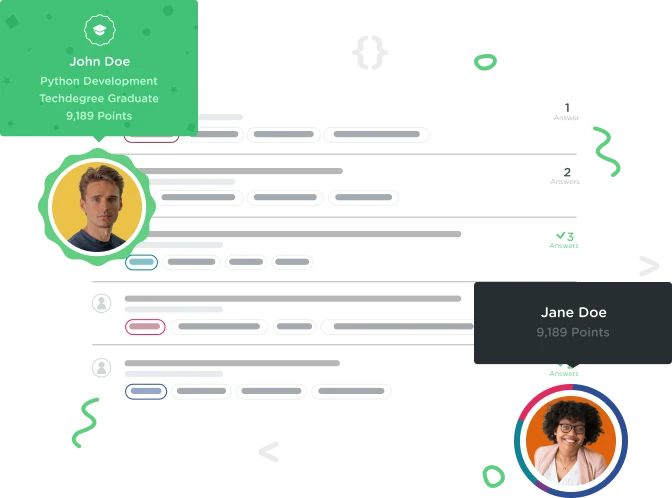
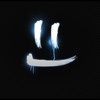
Grigorij Schleifer
10,365 Pointsequals (Object anObject) question ?
Hey folks,
I have a question regarding the use of String variables and their methods like equals().
In the video we created a String variable "noun" and stored a value from the console input inside of it. I know that the input from the console/Scanner etc. is always a String. And String is an Object, because it is a nonprimitive type right? So if we use the dot operator we can access the methods like equals() etc.
My first question is:
- Are this methods from the String class, or are they from the Obect class?
And my second question is about the equals method. The argument inside the equals method must be an Object. But we pass a String "dork" as a censored word into noun.equals("dork") .
- Can we pass everething except primitive types inside a method that expects an Object?
Thanks
Grigorij
3 Answers
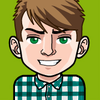
Harry James
14,780 PointsHey Grigorij!
Interesting questions! The String data type is actually a class itself and you're right, it's a non-primitive data type.
Now, when we call methods, they are generally off the class itself, in this case the String class. However, the equals()
method slightly different and is an example of a method overridden from its parent, in this case Object (String actually extends Object).
Now, when we override a method, we must make sure that the syntax is exactly the same as specified in the parent (There are some exclusions which I will discuss below). So what do I mean by this exactly? Well, in the Object class, the method syntax for the equals()
method is this:
public boolean equals(Object obj)
The String class must make sure its method looks exactly the same with some exclusions. The only things that may differ is the access modifier may be higher than originally defined (If in the parent it was protected, it could be set as public in the overriding class. It couldn't be private, however) and the name of any parameters can differ. So, that means you must always return the same type, always have the same name for the method, including case and always have the same number of parameters, with the same types in the same order.
Finally for your last question, yes we can pass in anything as an object except primitive types. Everything is a child of the Object class. Earlier up, I mentioned how String explicitly extends Object. It wouldn't actually make a difference if it didn't and in fact all classes can override Object's equals() method. Why? Because everything class is a child of the Object class.
Hopefully this should answer your questions but before I leave you with this, you may wonder how I know that String is overriding equals(), and not just using its own equals() method. This is where the documentation is your friend and from this link, we can see that the String equals()
specifically overrides the Object equals()
:
Awesome! Hopefully this should answer everything here for you but if there's still something bugging you, give me a shout and I'd love to answer anything else you're unsure on :)
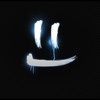
Grigorij Schleifer
10,365 PointsHarry,
you are awesome !
I definitely need more time to understand it. But I keep on reading, watching and coding .... I promise :)
Thank you so much for your great explanation
Grigorij
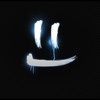
Grigorij Schleifer
10,365 PointsHi Harry,
I have more questions:)
How do you format your comments in a such advanced way. I mean pasting pictures and adding lines between text??? Can I learn this somewhere?
For example if I want add a link, I paste a link inside text. It looks horrible and not readable at all. But I want to do it more readable. For example here is a "link". And if the user clicks the word link, he is redirected to a page I want to show. Sorry for my bad english.
Thank you
Grigorij
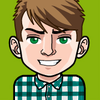
Harry James
14,780 PointsHey again Gigorij!
You sure can! Treehouse provides a Markdown Cheatsheet for you to use whenever you're posting something, it's just underneath the input field:
I'll also explain the pictures one for you as a lot of students get confused with how this works.

Here, the alt text is Alternate text. This is the text that is displayed when the image can't be shown. For example, those who use accessibility options may have the alternate text read out to them. Note that this text is optional.
You'll also notice on the Markdown Cheatsheet it allows you to set a title - this isn't actually used anywhere on the post so you can just ignore it. I believe it was previously used as a caption but not 100% sure on that.
There's three other formatting types you can use that I know aren't referenced on the Cheatsheet, so I'll also provide these here:
Dividers
Dividers like the ones shown on this post can be added with 3 dashes, ---
.
Titles
Titles are added by writing one or more dashes underneath a piece of text, like this:
Title
-----
Code text
With 3 backticks, we can open a code block. You can also surround text in one or two backticks so that it looks like this
. Here's how it looks in the editor:
``code formatted text``
(or)
`code formatted text`
Hope this helps and if you have any problems with using any of these, let me know and I'll edit one of your posts to use them. This way, you can also look at your own post by editing it and see how I went about formatting in that way :)
Also, I came across your forum reply over here and just wanted to give you a shoutout for that answer (I also gave you a +1!). Great to hear that you're helping out in the Community - we need people like you who love assisting others ;)
Grigorij Schleifer
10,365 PointsGrigorij Schleifer
10,365 PointsHey Harry,
very cool explanation!
But why we need to compare a String to an object? If I want to find equal words in a text from a console and I use stringName.equals("some word") is "some word" an object??? In my understaning we compare two String variables.
To be honest I don´t understand why we need to override the equals() method from Object class.
Grigorij
Harry James
14,780 PointsHarry James
14,780 PointsHey Grigorij!
Sorry I must've confused you a bit there. We don't need to override the equals() method - if we wanted to we could, however.
And yes, "some word" is an object, it is a String. In fact, we can even call methods off
"some word"
by putting a period.
after the declaration.I believe it's Java Data Structures where you'll learn about why this accepts any object. I'll talk about it briefly here though so you get an understanding.
You'll soon learn about the Comparable Interface in Java. This allows us to compare two objects of the same type to see if they are really equal. So normally what would happen is we'd have two "different" Strings:
Now these look the same don't they? But Java would actually see these as being different (They're two different instances of a String). How does Java know that them two variables are the same? To it, they are different. The Comparable interface allows us to compare our objects between one another.
So, the String class uses the Comparable interface to compare two String objects. This way, it knows if they are really the same by its definition of equal.
We can do the same with our classes, we tell Java what our version of two equal objects is. Don't worry if this doesn't quite make sense yet though, when you go through it in the course and utilize its powers yourself, you'll start understanding what I'm on about here. I'm keeping it basic here and it's actually a lot more powerful than it sounds :)
As always, if you need me to explain this concept in detail or a bit further then leave me a comment and I'll be sure to do so :)