Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial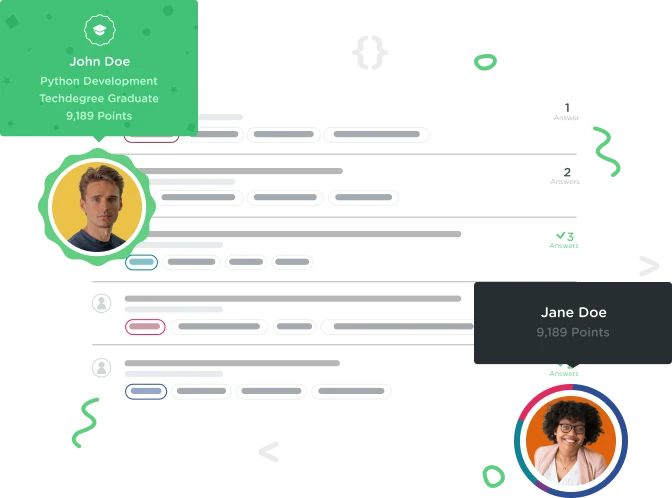

Ziad Alian
1,520 Pointserror
Any idea why i'm getting this error
./PezDispenser.java:32: error: cannot find symbol
int newAmount = mPezCount + PezAmount;
^
symbol: variable PezAmount
location: class PezDispenser
1 error
public class PezDispenser{ public static final int MAX_PEZ = 12; private String mCharacterName; private int mPezCount; public PezDispenser(String characterName) { mCharacterName = characterName; mPezCount = 0; }
public boolean dispense() { boolean wasDispensed = false; if (!isEmpty()){ mPezCount--; wasDispensed = true; } return wasDispensed; }
public boolean isEmpty(){ return mPezCount == 0; }
public void load() {
load(MAX_PEZ);
}
public void load(int pezAmount){ //^First p should be lowercase. int newAmount = mPezCount + pezAmount; if (newAmount > MAX_PEZ) { throw new IllegalArgumentException("Too many PEZ!!");
}
mPezCount = newAmount;
}
public String getCharacterName(){ return mCharacterName;
}
}
2 Answers
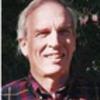
jcorum
71,830 PointsZiad, when I put your code into my IDE it compiles and runs fine. So let's try this. Delete your two files. Then copy this code and paste it into your IDE and save it as ExampleZiad.java:
public class ExampleZiad {
public static void main(String[] args) {
// Your amazing code goes here...
System.out.println("We are making a new Pez Dispenser.");
PezDispenserZiad dispenser = new PezDispenserZiad ("Yoda");
System.out.printf("The dispnenser chracter is %s\n",
dispenser.getCharacterName());
if (dispenser.isEmpty()) {
System.out.println("It's curently empty");
}
System.out.println("loading...");
dispenser.load();
if (!dispenser.isEmpty()) {
System.out.println("It's not longer empty");
}
while (dispenser.dispense()) {
System.out.println("Chomp!");
}
if (dispenser.isEmpty()) {
System.out.println("Ate all the PEZ");
}
dispenser.load(4);
dispenser.load(2);
while (dispenser.dispense()) {
System.out.println("Chomp!");
}
dispenser.load(400);
System.out.println("This will never happen");
}
}
Then copy this code and paste it in to a new file which you will save as PezDispenserZiad.java:
public class PezDispenserZiad {
public static final int MAX_PEZ = 12;
private String mCharacterName;
private int mPezCount;
public PezDispenserZiad(String characterName) {
mCharacterName = characterName;
mPezCount = 0;
}
public boolean dispense() {
boolean wasDispensed = false;
if (!isEmpty()){
mPezCount--;
wasDispensed = true;
}
return wasDispensed;
}
public boolean isEmpty(){
return mPezCount == 0;
}
public void load() {
load(MAX_PEZ);
}
public void load(int pezAmount){
//^First p should be lowercase.
int newAmount = mPezCount + pezAmount;
if (newAmount > MAX_PEZ) {
throw new IllegalArgumentException("Too many PEZ!!");
}
mPezCount = newAmount;
}
public String getCharacterName(){
return mCharacterName;
}
}
When you run ExampleZiad you should see the output:
We are making a new Pez Dispenser.
The dispnenser chracter is Yoda
It's curently empty
loading...
It's not longer empty
Chomp!
Chomp!
Chomp!
Chomp!
Chomp!
Chomp!
Chomp!
Chomp!
Chomp!
Chomp!
Chomp!
Chomp!
Ate all the PEZ
Chomp!
Chomp!
Chomp!
Chomp!
Chomp!
Chomp!
Exception in thread "main" java.lang.IllegalArgumentException: Too many PEZ!!
at PezDispenserZiad.load(PezDispenserZiad.java:33)
at ExampleZiad.main(ExampleZiad.java:32)
BTW: The exception at the end is deliberate.
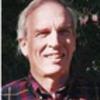
jcorum
71,830 PointsWhen I put your code in my IDE and fix the lines it compiles fine:
public class PezDispenser2{
public static final int MAX_PEZ = 12;
private String mCharacterName;
private int mPezCount;
public PezDispenser2(String characterName) {
mCharacterName = characterName;
mPezCount = 0;
}
public boolean dispense() {
boolean wasDispensed = false;
if (!isEmpty()){
mPezCount--;
wasDispensed = true;
}
return wasDispensed;
}
public boolean isEmpty(){
return mPezCount == 0;
}
public void load() {
load(MAX_PEZ);
}
public void load(int pezAmount){
//^First p should be lowercase.
int newAmount = mPezCount + pezAmount;
if (newAmount > MAX_PEZ) {
throw new IllegalArgumentException("Too many PEZ!!");
}
mPezCount = newAmount;
}
public String getCharacterName(){
return mCharacterName;
}
}
Although it hard to tell when the code isn't set off as code, it looks like the problem is that one of your lines of code ended up in a comment:
public void load(int pezAmount){ //^First p should be lowercase. int newAmount = mPezCount + pezAmount;

Ziad Alian
1,520 PointsLook i copied your code and pasted it in my ide
public class PezDispenser{
public static final int MAX_PEZ = 12; private String mCharacterName; private int mPezCount;
public PezDispenser(String characterName) { mCharacterName = characterName; mPezCount = 0; }
public boolean dispense() { boolean wasDispensed = false; if (!isEmpty()){ mPezCount--; wasDispensed = true; } return wasDispensed; }
public boolean isEmpty(){ return mPezCount == 0; }
public void load() { load(MAX_PEZ); }
public void load(int pezAmount){ //^First p should be lowercase. int newAmount = mPezCount + pezAmount; if (newAmount > MAX_PEZ) { throw new IllegalArgumentException("Too many PEZ!!"); } mPezCount = newAmount; }
public String getCharacterName(){ return mCharacterName;
} }
Example.java
public class Example {
public static void main(String[] args) {
// Your amazing code goes here...
System.out.println("We are making a new Pez Dispenser.");
PezDispenser dispenser = new PezDispenser ("Yoda");
System.out.printf("The dispnenser chracter is %s\n",
dispenser.getCharacterName());
if (dispenser.isEmpty()) {
System.out.println("It's curently empty");
}
System.out.println("loading...");
dispenser.load();
if (!dispenser.isEmpty()) {
System.out.println("It's not longer empty");
}
while (dispenser.dispense()) {
System.out.println("Chomp!");
}
if (dispenser.isEmpty()) {
System.out.println("Ate all the PEZ");
}
dispenser.load(4);
dispenser.load(2);
while (dispenser.dispense()) {
System.out.println("Chomp!");
}
dispenser.load(400);
System.out.println("This will never happen");
}
}
Now see the error that you will get i really struggling with this error for over than 5 days now and i can't get over it