Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial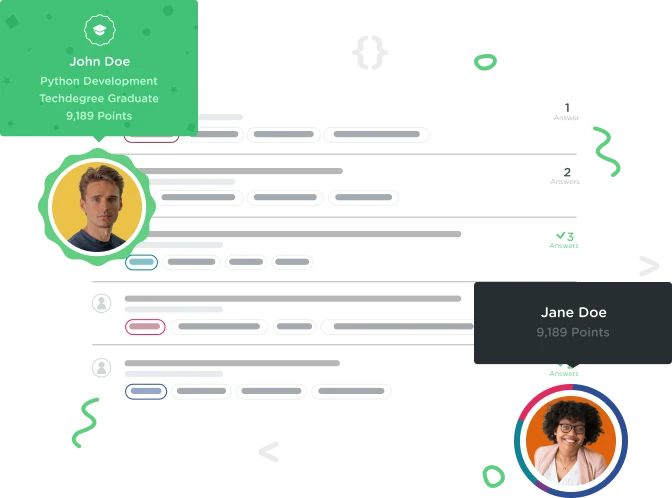
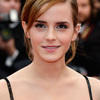
mohamadreza azadi
5,167 PointsError
i dont know exactly what i do and so confused the error say: ./ConferenceRegistrationAssistant.java:26: error: missing return statement } ^ Note: JavaTester.java uses unchecked or unsafe operations. Note: Recompile with -Xlint:unchecked for details.
public class ConferenceRegistrationAssistant {
/**
* Assists in guiding people to the proper line based on their last name.
*
* @param lastName The person's last name
* @return The line number based on the first letter of lastName
*/
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
/*
lineNumber should be set based on the first character of the person's last name
Line 1 - A thru M
Line 2 - N thru Z
*/
if ('A' < 'M') {
return lastName.charAt(0);
}
if ('N' > 'Z') {
return lastName.charAt(1);
}
}
}
public class Example {
public static void main(String[] args) {
/*
IMPORTANT: You can compare characters using <, >. <=, >= and == just like numbers
*/
if ('C' < 'D') {
System.out.println("C comes before D");
}
if ('B' > 'A') {
System.out.println("B comes after A");
}
if ('E' >= 'E') {
System.out.println("E is equal to or comes after E");
}
// This code is here for demonstration purposes only...
ConferenceRegistrationAssistant assistant = new ConferenceRegistrationAssistant();
/*
Remember that there are 2 lines.
Line #1 is for A-M
Line #2 is for N-Z
*/
int lineNumber = 0;
/*
This should set lineNumber to 2 because
The last name is Zimmerman which starts with a Z.
Therefore it is between N-Z
*/
lineNumber = assistant.getLineNumberFor("Zimmerman");
/*
This method call should set lineNumber to 1, because 'A' from "Anderson" is between A-M.
*/
lineNumber = assistant.getLineNumberFor("Anderson");
/*
Likewise Charlie Brown's 'B' is between 'A' and 'M', so lineNumber should be set to 1
*/
lineNumber = assistant.getLineNumberFor("Brown");
}
}
2 Answers

Mike Wagner
23,559 PointsYour return statements are essentially returning the first letter and second letter of the last name if A comes before M (first letter) and if N comes before Z (second letter). The first issue with this is that you are not returning an int
like the function expects, which makes your returns sort of go out the window. The second issue is that you're not actually comparing the character you retrieve to the parameters (and, of course, you're retrieving the wrong letter in the second if statement). What should be happening is you need to get the .charAt(0)
and probably store it as a Character
variable which you can then pass into your conditionals as something like
if ('A' <= returnedChar && returnedChar <= 'M') {
lineNumber = 1; // Since the variable is already created, we could use
// it and leave the return lineNumber at the end of the function,
// or we can simply "return 1;" here and "return 2;" in the other conditional.
}
and we should be good.
If you decide to ditch the lineNumber
variable in your if
s, you'll probably need to have a return
statement outside of your conditionals, probably something like return 0;
since it wants an int
but you don't want to return a 1 or 2 since those are valid data if we meet our conditions in the above checks. Personally, I would just leave the lineNumber
variable in place and have a single return
statement at the end of the function, like the Challenge had set up from the start.
If you need more help, or didn't understand something I said, I'll try to clarify. Good luck! :)

Chris Jones
Java Web Development Techdegree Graduate 23,933 PointsLooks like you're missing a return statement in the getLineNumberFor() method. You have return statements inside the scope of two IF statements, but Java will complain because not all logic branches have a return statement. In our mind, a letter should fall between A-M or N-Z, so it seems like all logic branches are returning a value, but Java just sees that two IF statements with nested return statements - it doesn't know that at least one of those IF statements will always apply to the parameter.
If you turn the second IF statement into an ELSE statement, then that should fix the error.
Let me know if you have any more questions.
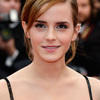
mohamadreza azadi
5,167 Pointsthanks chris jones but it does'nt work i change that code to if ('A' <='M') { return lastName.charAt(0); }
else{ return lastName.charAt(1); } and another error say : Bummer! Hmmm, I entered "Nelson" for the lastName and expected line number 2 to be returned, but instead it was 78

Chris Jones
Java Web Development Techdegree Graduate 23,933 PointsSorry about that. I just noted the first problem I saw. Didn't look through the rest of it. Mike Wagner's answer is much more thorough:). I'm glad you solved it!
mohamadreza azadi
5,167 Pointsmohamadreza azadi
5,167 Pointsunfortunately didn't understand:(
Mike Wagner
23,559 PointsMike Wagner
23,559 Pointsmohamadreza azadi - I'm not sure what you didn't understand, so I'm just going to post a functional interpretation of my answer here for you to look at.
This will check to see that
lastName.charAt(0)
is either between 'A' and 'M' or 'N' and 'Z' and adjust thelineNumber
to the requiredreturn
value.mohamadreza azadi
5,167 Pointsmohamadreza azadi
5,167 Pointsow thanks a lot!
Mike Wagner
23,559 PointsMike Wagner
23,559 Pointsmohamadreza azadi - of course. Glad to help. Make sure you choose an answer as best so that it shows as solved in the Community :)