Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial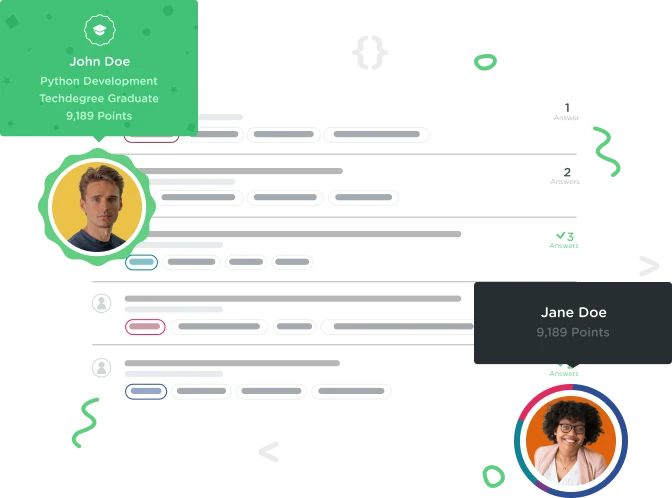

Luka Nixon
2,303 PointsError after clicking "Start Adventure" button
So my Story apps title page works, it allows the user to input their name, but when the click the button to progress to the next page it gives the message "Unfortunately, Signals From Mars has stopped. I'll post he LogCat and some of the primary files of the code.
LogCat:05-11 11:30:46.618: D/dalvikvm(1177): GC_FOR_ALLOC freed 62K, 5% free 2818K/2960K, paused 62ms, total 63ms 05-11 11:30:46.648: I/dalvikvm-heap(1177): Grow heap (frag case) to 4.113MB for 1350016-byte allocation 05-11 11:30:46.678: D/dalvikvm(1177): GC_FOR_ALLOC freed <1K, 4% free 4137K/4280K, paused 28ms, total 28ms 05-11 11:30:46.938: D/(1177): HostConnection::get() New Host Connection established 0xb71e6940, tid 1177 05-11 11:30:47.058: W/EGL_emulation(1177): eglSurfaceAttrib not implemented 05-11 11:30:47.068: D/OpenGLRenderer(1177): Enabling debug mode 0 05-11 11:30:56.558: D/AndroidRuntime(1177): Shutting down VM 05-11 11:30:56.558: W/dalvikvm(1177): threadid=1: thread exiting with uncaught exception (group=0xb3ae5ba8) 05-11 11:30:56.578: E/AndroidRuntime(1177): FATAL EXCEPTION: main 05-11 11:30:56.578: E/AndroidRuntime(1177): Process: com.lukan.signalsfrommars, PID: 1177 05-11 11:30:56.578: E/AndroidRuntime(1177): android.content.ActivityNotFoundException: Unable to find explicit activity class {com.lukan.signalsfrommars/com.lukan.signalsfrommars.StoryActivity}; have you declared this activity in your AndroidManifest.xml? 05-11 11:30:56.578: E/AndroidRuntime(1177): at android.app.Instrumentation.checkStartActivityResult(Instrumentation.java:1628) 05-11 11:30:56.578: E/AndroidRuntime(1177): at android.app.Instrumentation.execStartActivity(Instrumentation.java:1424) 05-11 11:30:56.578: E/AndroidRuntime(1177): at android.app.Activity.startActivityForResult(Activity.java:3424) 05-11 11:30:56.578: E/AndroidRuntime(1177): at android.app.Activity.startActivityForResult(Activity.java:3385) 05-11 11:30:56.578: E/AndroidRuntime(1177): at android.support.v4.app.FragmentActivity.startActivityForResult(FragmentActivity.java:839) 05-11 11:30:56.578: E/AndroidRuntime(1177): at android.app.Activity.startActivity(Activity.java:3627) 05-11 11:30:56.578: E/AndroidRuntime(1177): at android.app.Activity.startActivity(Activity.java:3595) 05-11 11:30:56.578: E/AndroidRuntime(1177): at com.lukan.signalsfrommars.MainActivity.startStory(MainActivity.java:46) 05-11 11:30:56.578: E/AndroidRuntime(1177): at com.lukan.signalsfrommars.MainActivity.access$1(MainActivity.java:43) 05-11 11:30:56.578: E/AndroidRuntime(1177): at com.lukan.signalsfrommars.MainActivity$1.onClick(MainActivity.java:37) 05-11 11:30:56.578: E/AndroidRuntime(1177): at android.view.View.performClick(View.java:4438) 05-11 11:30:56.578: E/AndroidRuntime(1177): at android.view.View$PerformClick.run(View.java:18422) 05-11 11:30:56.578: E/AndroidRuntime(1177): at android.os.Handler.handleCallback(Handler.java:733) 05-11 11:30:56.578: E/AndroidRuntime(1177): at android.os.Handler.dispatchMessage(Handler.java:95) 05-11 11:30:56.578: E/AndroidRuntime(1177): at android.os.Looper.loop(Looper.java:136) 05-11 11:30:56.578: E/AndroidRuntime(1177): at android.app.ActivityThread.main(ActivityThread.java:5017) 05-11 11:30:56.578: E/AndroidRuntime(1177): at java.lang.reflect.Method.invokeNative(Native Method) 05-11 11:30:56.578: E/AndroidRuntime(1177): at java.lang.reflect.Method.invoke(Method.java:515) 05-11 11:30:56.578: E/AndroidRuntime(1177): at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:779) 05-11 11:30:56.578: E/AndroidRuntime(1177): at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:595) 05-11 11:30:56.578: E/AndroidRuntime(1177): at dalvik.system.NativeStart.main(Native Method) 05-11 11:30:58.598: I/Process(1177): Sending signal. PID: 1177 SIG: 9
Client code: package com.lukan.signalsfrommars;
import android.support.v7.app.ActionBarActivity; import android.support.v7.app.ActionBar; import android.support.v4.app.Fragment; import android.text.Editable; import android.content.Intent; import android.os.Bundle; import android.view.LayoutInflater; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.view.ViewGroup; import android.os.Build; import android.widget.Button; import android.widget.EditText; import android.widget.Toast;
public class MainActivity extends ActionBarActivity {
private EditText mNameField;
private Button mStartButton;
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.fragment_main);
mNameField = (EditText) findViewById(R.id.nameEditText);
mStartButton = (Button) findViewById(R.id.startButton);
mStartButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
String name = mNameField.getText().toString();
startStory(name);
}
});
}
private void startStory(String name){ Intent intent = new Intent(this, StoryActivity.class); intent.putExtra(getString(R.string.key_name), name); startActivity(intent); }
}
Code for the second page:
package com.lukan.signalsfrommars;
import android.app.Activity; import android.content.Intent; import android.os.Bundle; import android.util.Log;
public class StoryActivity extends Activity{
public static final String TAG = StoryActivity.class.getSimpleName();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_story);
Intent intent= getIntent();
String name = intent.getStringExtra(getString(R.string.key_name));
if (name == null){
name = "Friend";
}
Log.d(TAG, name);
}
}
2 Answers

Brennan Agranoff
14,041 PointsTry harder

Brennan Agranoff
14,041 PointsGood question