Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial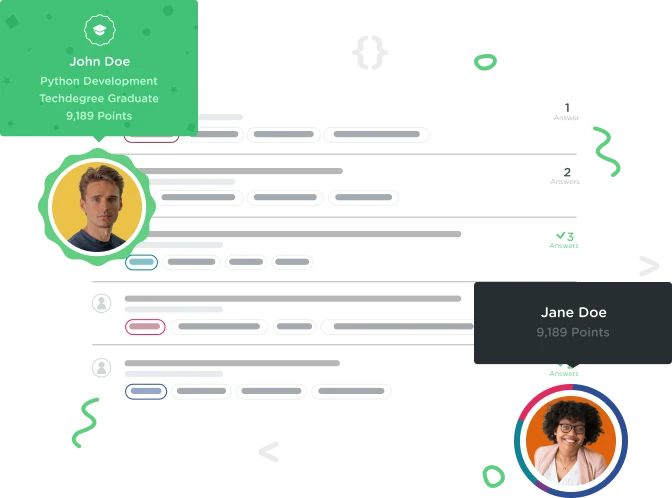
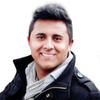
Camilo Lucero
27,692 PointsError: Arrow functions in methods not defined
Declaring a function as a method works fine when using the traditional function declaration, but is not defined when using an arrow function. Why?
const Add = {
one: addOne,
two: addTwo
}
function addOne(x) {
console.log(x + 1);
}
const addTwo = x => console.log(x + 2);
Add.one(5);
// => 6
Add.two(5);
// => addTwo is not defined
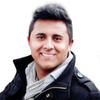
Camilo Lucero
27,692 PointsUnderstood, thank you Joseph!
1 Answer
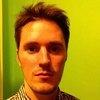
Brendan Whiting
Front End Web Development Techdegree Graduate 84,736 PointsThe issue isn't actually that it's an arrow function. It's because you're assigning it to a const variable lower down after your code to assign addTwo
to Add.two
. There's something called hoisting in JavaScript. And the behavior is slightly different for variable hoisting than for function hoisting.
In this example I've changed const addTwo
to var addTwo
.
const Add = {
one: addOne,
two: addTwo
}
function addOne(x) {
console.log(x + 1);
}
var addTwo = x => console.log(x + 2);
Add.two(5);
There's still an error, but now it's "TypeError: Add.two is not a function" instead of "ReferenceError: addTwo is not defined". Variables declared with var
get hoisted, but those with const
do not. So, now there is a variable called addTwo
hoisted up, but not the function itself is being hoisted up (see the article). Assigning a regular function to the variable produces the same error, even though it's not an arrow function:
var addTwo = function(x) {
console.log(x + 2);
}
But if you move the whole thing - arrow function or not, var or const - to the top of the file, it works, because it doesn't run into that hoisting issue:
const addTwo = x => console.log(x + 2);
const Add = {
one: addOne,
two: addTwo
}
function addOne(x) {
console.log(x + 1);
}
Add.two(5);
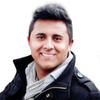
Camilo Lucero
27,692 PointsVery clear! Thanks a lot, Brendan.
Joseph Perez
25,122 PointsJoseph Perez
25,122 PointsHi Camilo!
The difference between your addOne function and addTwo function is that one is a function declaration and one is a function expression. Function declarations are actually hoisted, which is why the method call to Add.one works. The hoisting is what allows your function to be used even though it was defined after the code actually using it.
The addTwo variable is being assigned a function expression which is not hoisted and cannot be used before it's defined.
Try moving this piece of code to the very top of the file before the Add variable. That should fix the error you were encountering. :D
const addTwo = x => console.log(x + 2);