Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial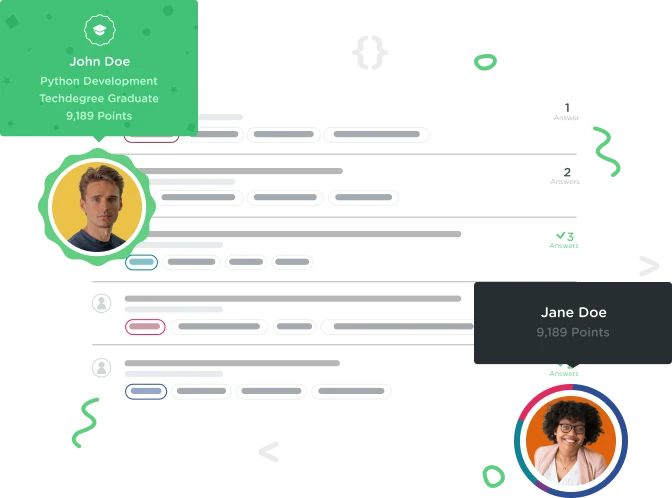

Thomas Williams
9,447 PointsError: @BindView not applicable to local variable or cannot resolve 'R2'......
My gradle Module: app code:
apply plugin: 'com.android.application'
apply plugin: 'com.neenbedankt.android-apt'
android {
compileSdkVersion 24
buildToolsVersion "24.0.3"
defaultConfig {
applicationId "grouppay.com.stormy"
minSdkVersion 15
targetSdkVersion 24
versionCode 1
versionName "1.0"
testInstrumentationRunner "android.support.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
}
}
}
dependencies {
compile 'com.jakewharton:butterknife:8.4.0'
apt 'com.jakewharton:butterknife-compiler:8.4.0'
compile fileTree(dir: 'libs', include: ['*.jar'])
androidTestCompile('com.android.support.test.espresso:espresso-core:2.2.2', {
exclude group: 'com.android.support', module: 'support-annotations'
})
compile 'com.android.support:appcompat-v7:24.2.1'
testCompile 'junit:junit:4.12'
compile 'com.squareup.okhttp3:okhttp:3.4.1'
}
My gradle Project: Stormy code:
buildscript {
repositories {
jcenter()
mavenCentral()
}
dependencies {
classpath 'com.android.tools.build:gradle:2.2.1'
classpath 'com.neenbedankt.gradle.plugins:android-apt:1.8'
// NOTE: Do not place your application dependencies here; they belong
// in the individual module build.gradle files
}
}
allprojects {
repositories {
jcenter()
}
}
task clean(type: Delete) {
delete rootProject.buildDir
}
My MainActivity code:
@BindView(R.id.timeLabel) TextView mTimeLabel;
@BindView(R2.id.humdityLabel) TextView mHumidityValue;
For @BindView(R.id.timeLabel) TextView mTimeLabel; i get @BindView not applicable to local variable error.
As per the the instructions on the butternife readMe file...
Now make sure you use R2 instead of R inside all Butter Knife annotations.
class ExampleActivity extends Activity { @BindView(R2.id.user) EditText username; @BindView(R2.id.pass) EditText password; ... }
I tried '@BindView(R2.id.humdityLabel) TextView mHumidityValue;' but got cannot resolve R2.
Any help/solutions/advice before i give up on butterknife will be truly appreciated.
3 Answers

Seth Kroger
56,413 PointsAnnotations can be defined to be used only in certain contexts. For instance @Override only makes sense with methods, so it's definition says it's used to only annotate methods. The same goes for custom annotations. Unless the annotation is generally applicable, it's best to define what it can be used for. Java Annotations goes over this if you're interested.
BindView.java:
@Retention(CLASS) @Target(FIELD)
public @interface BindView {
/** View ID to which the field will be bound. */
@IdRes int value();
}
@BindView (and @InjectView as well) is defined to be used on member fields only. When you say "i get @BindView not applicable to local variable error." it's quite literal. You should pull them out of the method (onCreate() I'm guessing?) and make them private member variables.
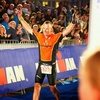
Steve Hunter
57,712 PointsHi Thomas,
I did this project some time ago so there will have been updates to both Android Studio and ButterKnife. I'm using Butterknife 6.1.0.
In my module's build.gradle, I have:
dependencies {
.
.
compile 'com.jakewharton:butterknife:6.1.0'
.
.
}
In my MainActivity
I am injecting the view with:
@InjectView(R.id.timeLabel) TextView mTimeLabel;
@InjectView(R.id.humidityValue) TextView mHumidityValue;
protected void onCreate(Bundle savedInstanceState){
.
.
ButterKnife.inject(this);
.
}
So, that uses @InjectView
rather than @BindView
.
As I say, this may be due to the earlier project using earlier versions of ButterKnife - that version is likely to still work; maybe try backdating the version number to get the code working, then try the updates?
I hope that helps; it probably doesn't!
Steve.

Thomas Williams
9,447 PointsI cant believe thats what it was, what a muppet, think I was focusing too much on the gradle code because i'm not so familiar with it. Pulling the code out of onCreate() and placing them as member variables solved my problem. Many thanks.