Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial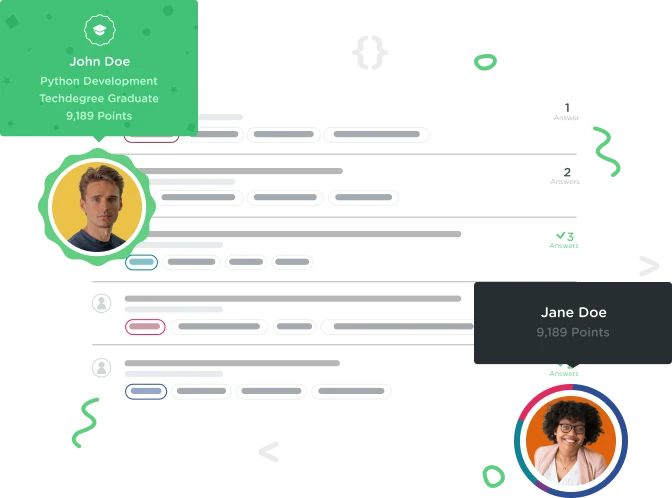

martin2s
1,937 Pointserror calling a method with two input arguments in an enum
Hi, I'd really appreciate some help or pointers, as i've been hitting my head against a wall trying to solve this error. It's no doubt something basic, but i cannot for the life of me figure out the problem.
I managed to solve the challenge that was set without any problems (ie i created the init method without any problems and the test was passed). However, i then decided to play around a bit in order to see how i would call the method in practice. See attached code.
So i created two instances of type Coin, and then called the isGreater method in order to compare coin1 and coin2. Unfortunately, i get an error: Playground execution failed: MyPlayground enums.playground:24:25: error: missing argument label 'newValue:' in call result = coin1.isGreater(coin1,coin2)
Surely coin2 is the newValue property ?
I think i'm misunderstanding something basic eg maybe you cannot make reference to two separate instances in a single method ?
Any help would be appreciated.
thanks, martin.
enum Coin: Int {
case Penny = 1, Nickel = 5, Dime = 10, Quarter = 25
func isGreater(currentValue: Coin, newValue: Coin) -> Bool {
return currentValue.rawValue > newValue.rawValue
}
init () {
self = Coin.Quarter
}
}
var coin1 = Coin()
var coin2 = Coin()
var result: Bool
result = coin1.isGreater(coin1,coin2)
2 Answers

Donald White
5,743 PointsThis is a bit tricky. Since you're using Coin as the parameter, and it contains an init method, it's being viewed as a method rather than a normal value. All Init methods require the parameter name. Swift provides an automatic external name for every parameter in an initializer if you don’t provide an external name yourself. It's confusing since the first parameter isn't being required as an external parameter would be normally, even though both parameters are identical. Swift allows for the first label to be omitted, and even if you put it in, you'll see a warning: "extraneous argument label 'currentValue' in call".
By adding a few more examples you'll see that you can achieve the value/style you want.
enum Coin: Int {
case Penny = 1, Nickel = 5, Dime = 10, Quarter = 25
// original Function
func isGreater(currentValue: Coin, newValue: Coin) -> Bool {
return currentValue.rawValue > newValue.rawValue
}
// Function using instance value
func isGreaterThan(newValue: Coin) -> Bool {
return self.rawValue > newValue.rawValue
}
init () {
self = Coin.Quarter
}
}
var coin1 = Coin()
var coin2 = Coin()
var result: Bool
// Throws error in compiler
result = coin1.isGreater(coin1, coin2)
// No error in compiler - result = false
result = coin1.isGreater(coin1, newValue: coin2)
coin2 = Coin.Penny
// result = true
result = coin1.isGreaterThan(coin2)
// Example function using primitives
func isGreat(value1: Int, value2: Int) -> Bool {
return value1 > value2;
}
var result2 : Bool
// result2 = true
result2 = isGreat(4, 3)
Hope that helps!

zacharyjefferies
6,758 PointsHey Martin,
When I copy and pasted your code into a playground of my own, the error popped up, just like you said, and suggested that you insert 'newValue:' into your code like this: result = coin1.isGreater(coin1, newValue: coin2) To be honest, I have no idea why this is necessary, it's probably something worth looking into. But when I put that in, everything worked fine. It returned false because coin1 and coin2 have the same value, and when I put in greater than or equal to instead of just greater than, it returned true.
Hope I helped, Zach

martin2s
1,937 PointsHi zach - thanks for getting back to me, that's really kind. Cheers, Martin.

zacharyjefferies
6,758 PointsNo problem, sorry I couldn't explain the problem in more detail.
martin2s
1,937 Pointsmartin2s
1,937 PointsHi Donald, Thanks for taking the time to put together your response, that's a really good answer.
I didn’t realise at all that my parameter was being interpreted as a method - and I can see how that would cause some confusion at runtime (....even if I don't fully understand the ramifications, at this stage in my learning).
The examples you gave were really helpful - I've discarded the first variant altogether - tbh, I don't really understand it well enough. (ie the No error in compiler version).
However, the "Function using instance value" makes a lot of sense, and I can see how this would work. Similarly the standalone function using primitives is also easy to understand.
Thanks again - really appreciated.
Martin.