Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial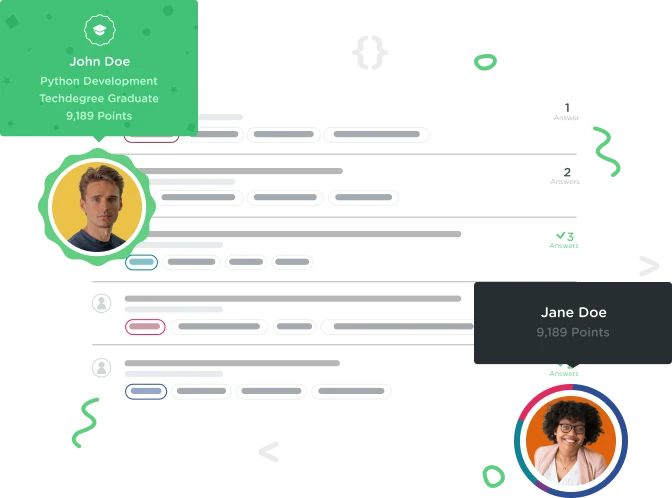

Eduardo Calvachi
6,049 PointsError cannot convert value of type 'SuperEnemy' to expected argument of type 'LaserTower'
I am getting an error: Cannot convert value of type 'SuperEnemy' to expected argument of type 'LaserTower' when I try to pass in superEnemy to the fireAtEnemy method of LaserTower as described on the video. Here are my classes and subclasses, maybe I missed something:
class Enemy {
var life: Int = 2
var position: Point
init(x: Int, y: Int){
self.position = Point(x: x, y: y)
}
func dicreaseHealth(factor: Int){
life -= factor
}
}
class Tower {
let position: Point // tower doesn't move
var range: Int = 1
var strength: Int = 1
init(x: Int, y: Int ){
self.position = Point(x: x, y: y)
}
func fireAtEnemy(enemy: Enemy){
if inRange(self.position, range: self.range, target: enemy.position) {
while enemy.life > 0 {
enemy.dicreaseHealth(self.strength)
print("Enemy has been hit!")
}
} else {
print("Enemy out of range!")
}
}
func inRange(position: Point, range: Int, target: Point) -> Bool{
let availablePositions = position.surroundingPoints(withRange: range)
for point in availablePositions {
if point.x == target.x && point.y == target.y {
return true
}
}
return false
}
}
class SuperEnemy: Enemy {
let isSuper: Bool = true
override init(x: Int, y: Int) {
super.init(x: x, y: y)
self.life = 10
}
}
class LaserTower: Tower {
override init(x: Int, y: Int) {
super.init(x: x, y: y)
self.range = 100
self.strength = 100
}
override func fireAtEnemy(enemy: Enemy) {
while enemy.life > 0 {
enemy.dicreaseHealth(self.strength)
print("Enemy has been Vanquished!")
}
}
}
Class Instantiation:
let laserTower = LaserTower(x: 0, y: 0)
let superEnemy = SuperEnemy(x: 20, y: 20)
LaserTower.fireAtEnemy(superEnemy)
//Error: cannot convert value of type 'SuperEnemy' to expected argument of type 'LaserTower'
1 Answer
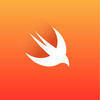
Steven Deutsch
21,046 PointsHey Eduardo Calvachi,
You need to change the capital "L" in LaserTower to a lowercase "l". You need to call the fireAtEnemy method on the instance of LaserTower that you assigned to your laserTower constant.
let laserTower = LaserTower(x: 0, y: 0)
let superEnemy = SuperEnemy(x: 20, y: 20)
LaserTower.fireAtEnemy(superEnemy)
//Error: cannot convert value of type 'SuperEnemy' to expected argument of type 'LaserTower'
// It should be
laserTower.fireAtEnemy(superEnemy)
Good Luck!
Eduardo Calvachi
6,049 PointsEduardo Calvachi
6,049 PointsYou're right Steven I was calling on the object rather than the instance. Thanks for finding the mistake!