Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial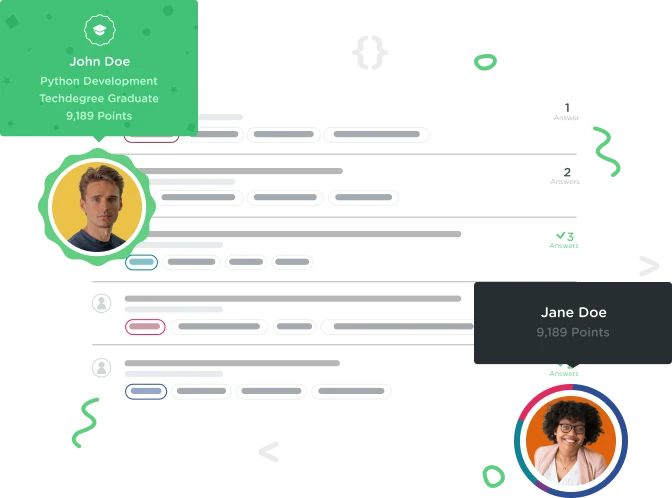
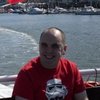
Sean Flanagan
33,235 PointsError: cannot implicitly convert type "double" to "int"
Hi. I got this error message after running my program;
Program.cs(35,39): error CS0266: Cannot implicitly convert type `double' to `int'. An explicit conversion exists (are you missing a cast?)
Here's my program:
using System;
namespace Treehouse.PracticeSession
{
class Program
{
public static void Main()
{
// TODO Declare a boolean variable named "keepGoing"
// and assign it a value of "true". Use this variable to
// know when to exit the while loop.
bool keepGoing = true;
// TODO Define a while loop.
// Keep looping as long as the variable "keepGoing" has a value of "true".
while (keepGoing)
{
// TODO Prompt the user with the text "Enter a number:"
Console.Write("Enter a number or type \"quit\" to exit: ");
// and assign their value to an "entry" string variable.
string entry = System.Console.ReadLine();
// TODO If the user entered the text "quit"...
if (entry == "quit")
// TODO Exit the program.
{
keepGoing = false;
}
// TODO Else the user didn't enter the text "quit"...
else
{
// TODO Parse the user's entry to an integer
int number = int.Parse(entry);
// TODO Square the user's provided number
// (i.e. multiply the number by itself).
int square = Math.Pow(number, 2); // This line caused the error.
// TODO Output the result.
// Example: "The square of 2 is 4." or
// "2 multiplied by itself is equal to 4."
Console.WriteLine("The square of " + number + " is " + square + ".");
}
}
// TODO Output the text "Goodbye!" after exiting the loop.
keepGoing = false;
Console.WriteLine("Goodbye!");
}
}
}
I then changed the type for square
to the more general var
and the program worked perfectly.
What is it about int
that triggers this error?
2 Answers
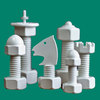
Steven Parker
231,533 PointsThe "Math.pow" function returns a double, so assigning it to an "int" variable causes the error because it cannot be implicitly converted.
But declaring your variable using "var" causes the type to be matched to type being assigned, so this causes the type of the variable "square" to be "double".
Note that "var" is not a type, but a request to the compiler to determine the type for you. That's why you cannot declare something as "var" unless it is being initialized at the same time.
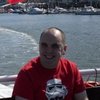
Sean Flanagan
33,235 PointsThank you Steven.