Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial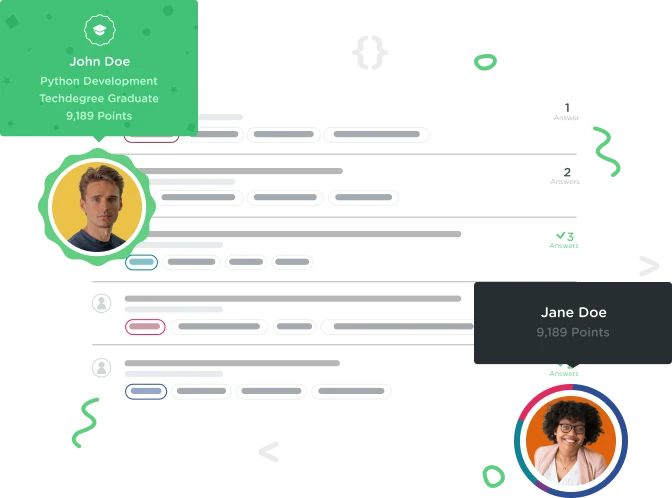

Michael Stolp-Smith
4,855 PointsError: com.teamtreehouse.Treet cannot be cast to java.lang.Comparable
I am getting an unfamiliar error with this. I've checked for syntax errors multiple times and I'm not sure what's going on. (I am using "this." instead of the "m" prefix, but that should not be an issue here as far as I can tell, and hasn't been up to this point.)
The error:
Exception in thread "main" java.lang.ClassCastException: com.teamtreehouse.Treet cannot be cast to java.lang.Comparable
at java.util.ComparableTimSort.countRunAndMakeAscending(ComparableTimSort.java:320)
at java.util.ComparableTimSort.sort(ComparableTimSort.java:188)
at java.util.Arrays.sort(Arrays.java:1246)
at Example.main(Example.java:30)
the code:
package com.teamtreehouse;
import java.util.Date;
public class Treet implements Comparable{
private final String author;
private final String description;
private final Date creationDate;
public Treet(String author, String description, Date creationDate){
this.author = author;
this.description = description;
this.creationDate = creationDate
}
@Override
public String toString(){
return String.format ("Treet: \"%s\" by %s on %s",
this.description,
this.author,
this.creationDate);
}
@Override
public int compareTo(Object obj){
Treet other = (Treet) obj;
if (equals(other)){
return 0;
}
int dateCmp = this.creationDate.compareTo(other.this.creationDate);
if (dateCmp == 0){
return this.description.compareTo(other.this.description);
}
return dateCmp;
}
public String getAuthor(){
return this.author;
}
public Date getCreationDate(){
return this.creationDate;
}
public String getDescription(){
return this.description;
}
public String[] getWords(){
return this.description.toLowerCase().split("[^\\w@#']");
}
}
import com.teamtreehouse.Treet;
import java.util.Arrays;
import java.util.Date;
public class Example{
public static void main(String[] args){
Treet treet = new Treet(
"Mike",
"This is a cool post, man. @treehouse "+
"is teaching me a lot",
new Date(1488402048000L)
);
Treet secondTreet = new Treet(
"journeytocode",
"@treehouse makes learning Java sooooo fun! #treet",
new Date(1421878767000L)
);
System.out.printf("This is a new Treet: %s %n", treet);
System.out.println("The words are:");
for (String word: treet.getWords()){
System.out.println(word);
}
Treet[] treets = {treet, secondTreet};
Arrays.sort(treets);
for (Treet exampleTreet : treets){
System.out.println(exampleTreet);
}
}
}
2 Answers
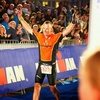
Steve Hunter
57,712 PointsHi Michael,
There's some code in there that implies you want to substitute a prefix 'm' with a this.
. That's not how that works.
The 'm' prefix is merely a naming convention - some don't use it anymore but it is still valid code - substituting an 'm' prefix for a this.
is a totally different thing.
I understand why you don't want to prefix with an 'm'. That's fine. But just use the resulting non-m variable name as you would the with-m variable name. this
is used for something different, usually/often within a constructor to differentiate, but hey ...
I'm on my tablet tonight as the work machines are doing their thing in the office. But I'll look again in the morning. However, in your compareTo
there are too many chained dot-notations involving this
. Indeed, other.this.creationDate
is impossible. You're comparing this.creationDate
, i.e. a member variable of this instance to a member variable of the same (obviously) name of another instance of the same class but using other.this.creationDate
. A member variable can't belong to other
and this
at the same time. You're comparing the values of two instances of the same class's member variables. You can't have other.this
.
You have two class instances/objects. One is other
and the one you're 'in' is this
. Both have a member variable called creationDate
you are comparing them with compareTo()
. One side of the comparison is this.creationDate
the other side is other.creationDate
. Never the twain shall meet. They are wholly distinct objects.
I hope that helps.
Steve.

Michael Stolp-Smith
4,855 PointsThat was definitely helpful. This wasn't something I had considered as an issue, but it makes sense! Thanks for the help. removing the "this." prefix solved the error.
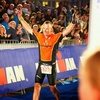
Steve Hunter
57,712 PointsExcellent - I'm glad you got it fixed and that my post made sense to you!!