Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial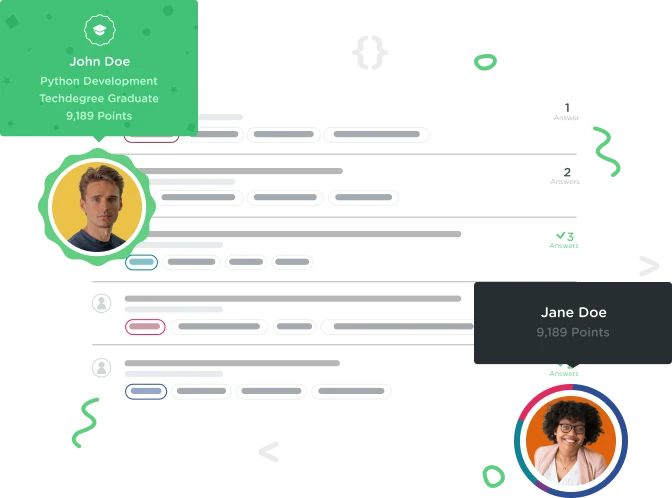

lukej
34,222 PointsError Creating the pickLunchSpot Function
Hi, I can not recreate the pickLunchSpot function, that Craig demonstrated in JShell. "Illegal start of expression error keeps coming up. Can someone help me out?
import java.util.Random;
public class RandomlyPickLunchSpot {
public static void main(String[] args) {
// method takes varargs: an indefinite number of parameters of type String
// and turns them into an array
// randomly pick one item of the array
public static String pickLunchSpot(String... spots) {
System.out.printf("Randomly picking %d lunch spots. %n",
spots.length);
Random random = new Random();
return spots[random.nextInt(spots.length)];
}
String lunchSpot = pickLunchSpot("Que Sabrosa", "Las Primas", "Life of Pie");
System.out.printf("%s %n", lunchSpot);
}
}
The following error comes up:
RandomlyPickLunchSpot.java:10: error: illegal start of expression
public static String pickLunchSpot(String... spots) {
^
RandomlyPickLunchSpot.java:17: error: <identifier> expected
System.out.printf("%s %n", lunchSpot);
^
RandomlyPickLunchSpot.java:17: error: illegal start of type
System.out.printf("%s %n", lunchSpot);
^
RandomlyPickLunchSpot.java:20: error: class, interface, or enum expected
}
^
4 errors
1 Answer
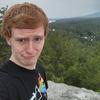
Brendon Butler
4,254 PointsThe problem is that you can't encapsulate one java method within another. Your pickLunchSpot method is inside your main method. Instead, you need to close your main method, and put the pickLunchSpot method outside of the braces. Like this:
import java.util.Random;
public class RandomlyPickLunchSpot {
public static void main(String[] args) {
String lunchSpot = pickLunchSpot("Que Sabrosa", "Las Primas", "Life of Pie");
System.out.printf("%s %n", lunchSpot);
} /* close main method here */
/* start pickLunchSpot method here (EDIT: removed default comments for visual clarity) */
public static String pickLunchSpot(String... spots) {
System.out.printf("Randomly picking %d lunch spots. %n",
spots.length);
Random random = new Random();
return spots[random.nextInt(spots.length)];
}
}