Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial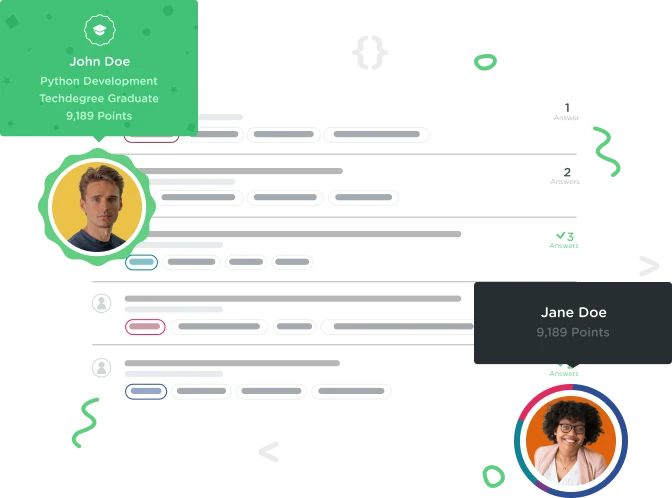
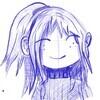
Jack Stone
9,838 Pointserror CS0117
Getting the following error when typing trying to run code.
Game.cs(13,19): error CS0117: System.Console' does not contain a definition for
Writeline'
/usr/lib/mono/4.5/mscorlib.dll (Location of the symbol related to previous error)
Map.cs(17,22): error CS1061: Type TreehouseDefence.Point' does not contain a definition for
x' and no extension method x' of type
TreehouseDefence.Point' could be found. Are you missing an assembly reference?
Code is as follows: (Game.cs)
using System;
namespace TreehouseDefence
{
class Game
{
public static void Main()
{
Map map = new Map(8, 5);
Point point = new Point (4, 2);
bool isOnMap = map.OnMap (point);
Console.Writeline(isOnMap);
point = new Point (8, 5);
isOnMap = map.OnMap (point);
Console.WriteLine (isOnMap);
}
}
}
(map.cs)
namespace TreehouseDefence
{
class Map
{
public readonly int Width;
public readonly int Height;
public Map(int width, int height)
{
Width = width;
Height = height;
}
public bool OnMap(Point point)
{
return point.x >= 0 && point.X < width &&
point.Y >=0 && point.Y < Height;
}
}
}
3 Answers
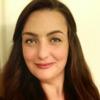
Jennifer Nordell
Treehouse TeacherHi there! It's correct. There is no Writeline
in the System
class. There is, however, a WriteLine
in that class. Remember, capitalization matters here.
Hope this helps!
edited for additional information
If you're still receiving an error about Point
, we will need to see your code in Point.cs
.
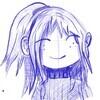
Jack Stone
9,838 PointsStill having the same error with the x point
namespace TreehouseDefence
{
class Point {
public readonly int X;
public readonly int Y;
public Point (int x, int y)
{
X = x;
Y = y;
}
}
}
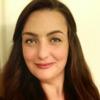
Jennifer Nordell
Treehouse TeacherHi again! Again, capitalization is your enemy here.
You've written:
return point.x >= 0 && point.X < width &&
point.Y >=0 && point.Y < Height;
But both X and Y should be capitalized and both Width
and Height
should be capitalized:
return point.X >= 0 && point.X < Width &&
point.Y >=0 && point.Y < Height;
You simply forgot to capitalize the first X
and Width
.
Hope this helps!
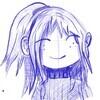
Jack Stone
9,838 PointsYou're a star.
Thank you very much.