Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial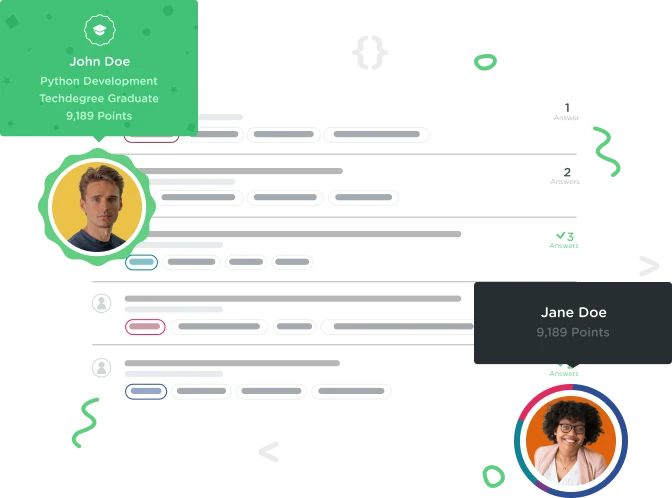

Mo Reese
11,868 PointsError debug, please help
class Account():
def __init__(self,owner,balance):
self.owner = owner
self.balance = balance
def deposit(self,amt):
self.balance = self.balance + amt
print(f"Your deposit of {amt} has been accepted! Your new account balance is: ${self.balance:1.2f}")
def withdraw(self,amt):
if self.balance:
try:
self.balance - amt
if amt > self.balance:
print(f"You have insufficient funds for this request, please choose an amount of {self.balance:1.2f} or less")
else:
print(f"Your request has been accepted. Your new account balance is {self.balance:1.2f - amt}")
else:
print("The account has a zero balance.")
For some reason, that last line "else: print("The account has a zero balance")" is giving me an error of: unexpected unindent. Although I think it is correct, I've still tried to align it differently, but to no avail. What are my tired eyes just not seeing?
2 Answers

Wendy Leung
5,322 PointsYou have a try
block without an except
block. You need both when you're using try/except
for error handling.
Replace the last else
with except
, then indent it so it aligns with try
.
Explanation of try except: https://www.w3schools.com/python/python_try_except.asp

Tlotlo Molokwe
16,618 PointsTry aligning the 2nd else statement with the first if statement, to me they don't look aligned
Mo Reese
11,868 PointsMo Reese
11,868 Points@Wendy Leung, thanks to your comment, I was able to see that I was using "try" incorrectly-thank you also for the link so that I could refresh myself. Ultimately, I ended up rewriting & simplifying, which fixed the issue.