Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial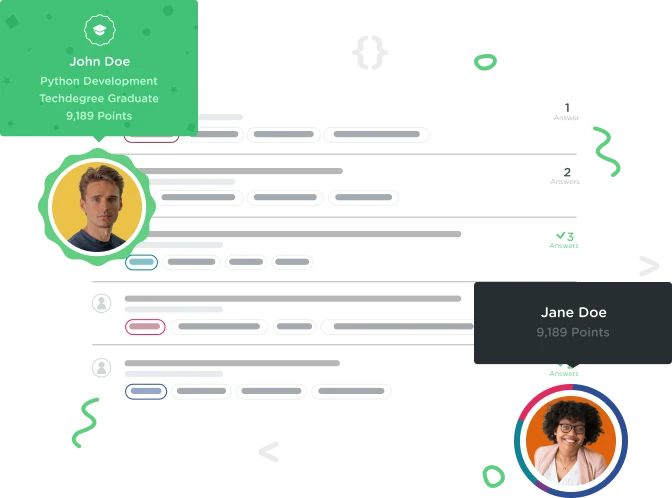
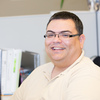
Anthony Romero
6,364 PointsError doesn't make sense: "Bummer! `odds()` didn't return the right output. Got [1, 3, 5, 7], expected kaoa"
I thought the code challenge was asking for odd number output. I'm not sure if this is a bug.
def first_4(orig_list):
new_list = []
for item in range(len(orig_list)):
new_list.append(item)
return new_list[1:5]
def odds(orig_list):
new_list = []
for item in range(len(orig_list)):
new_list.append(item)
return new_list[1::2]
orig_list = list(range(1,12))
print (first_4(orig_list))
print (odds(orig_list))
2 Answers
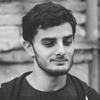
Nicolas Sandller
5,643 PointsHey Anthony,
This worked for me:
def first_4(iterable):
return iterable[0:4]
def odds(iterable):
return iterable[1::2]
The reason being, you need to slice odd numbers, and slicing starts from the indicated index number. So if you start fro 1 and jump in 2's the function will always return odd indexes.
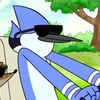
Zachary Fine
10,110 PointsI dont understand this line: " for item in range(len(orig_list)):"
I believe it is asking you to return the odd 'letters' within the dict. Lets assume list is [1,2,3,4,5], then len(list) = 5 and range(5) = [0,1,2,3,4].
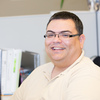
Anthony Romero
6,364 PointsI filled a list with a range of numbers from 1 to 12 in the main program. This list is called orig_list. I call the function with the following line: print (first_4(orig_list)) to pass the list to the first function first_4(). That was the first code challenge and I passed that portion.
The second challenge seemed to be a similar task except I thought it was asking for odd numbered items within the same list. I believe we were supposed to build upon what was coded before. We have not covered dictionaries yet, but your response led to to using string instead of numbers.
I redid the challenge and passed "Oklahoma" into the function. It picked out every other letter within the word. Oklahoma was never mentioned in the challenge but it worked with "kaoa" error response and I completed the challenge.
Anthony Romero
6,364 PointsAnthony Romero
6,364 PointsHere is the requested task for the odds function: "Make a function named odds that accepts an iterable as an argument and returns the items with an odd index in the iterable."