Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial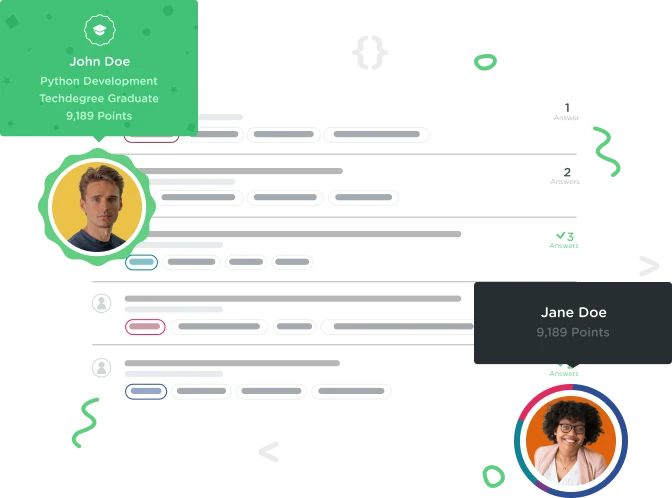

Prem Agrawal
117 Pointserror: Enumerations.playground:48:20: error: 'init(colorLiteralRed:green:blue:alpha:)' is unavailable: This initializer
enum ColorComponent{ case rgb(Float,Float,Float,Float); func color()->UIColor{ switch self{ case .rgb(let red, let green, let blue, let alpha): return UIColor(colorLiteralRed:red/255.0,green:green/255.0,blue:blue/255.0,alpha: alpha) } } } ColorComponent.rgb(61,120,128,1.0).color() Whats wrong with this Code?
10 Answers
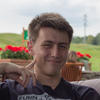
Jonatan Witoszek
15,384 PointsIt seems to me that colorLiteralRed had been depreaced in the newer versions of Swift and is used to store the new color literals.
You can use different UIColor methods instead:
UIColor(displayP3Red:, green:, blue:, alpha: )
or
UIColor(red: , green: , blue: , alpha: )
Just remember to also change the type from Float to CGFloat just like in the hsb example.
Also good to know there is now a simpler, more visual way to store color values in Swift as i mentioned before. You can read more about it here: https://medium.com/@gurdeep060289/color-image-new-literals-in-the-cocoa-town-7ef4f2710194
Reference: https://stackoverflow.com/questions/45361704/initcolorliteralred-green-blue-alpha-deprecated-in-swift-4, https://stackoverflow.com/questions/46344226/error-uicolorcolorliteralred

Alexei Parphyonov
34,128 PointsI have worked it out this way. It works, and the result of RGBA is the same as in the video.
enum ColorComponent {
// rgb - red, green, blue
// hsb - hue, saturation, brightness
case rgb(red: Float, green: Float, blue: Float, alpha: Float)
case hsb(hue: CGFloat, saturation: CGFloat, brightness: CGFloat, alpha: CGFloat)
// In Swift each enum member can store associated values of other types along with this member value
func color() -> UIColor {
switch self {
case .rgb(let red, let green , let blue, let alpha):
return UIColor(red: CGFloat(red/255), green: CGFloat(green/255.0), blue: CGFloat(blue/255.0), alpha: CGFloat(alpha))
case .hsb(let hue, let saturation, let brightness, let alpha):
return UIColor(hue: hue/360.0, saturation: saturation/100.0, brightness: brightness/100.0, alpha: alpha)
}
}
}
let rgbMember = ColorComponent.rgb(red: 61.0, green: 120.0, blue: 198.0, alpha: 1.0).color()
let hsbMember = ColorComponent.hsb(hue: 26.09, saturation: 68.05, brightness: 66.27, alpha: 1.0).color()
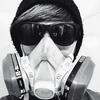
Gavin Hobbs
5,205 PointsI checked out the link Jonatan Witoszek provided, the one with the medium article, and I found a way!
Here's my code!
import UIKit
enum ColorComponent {
case rgb(Float, Float, Float, Float)
case hsb(CGFloat, CGFloat, CGFloat, CGFloat)
func color() -> UIColor {
switch self {
case .rgb(let red, let green, let blue, let alpha):
return #colorLiteral (red: red/255.0, green: green/255.0, blue: blue/255.0, alpha: alpha)
case .hsb(let hue, let saturation, let brightness, let alpha):
return UIColor(hue: hue/36.0, saturation: saturation/100.0, brightness: brightness/100.0, alpha: alpha)
}
}
}
ColorComponent.rgb(61.0, 120.0, 198.0, 1.0).color()
... And YES! It works!!!
This is what the Medium author, Gurdeep Singh, said...
extension UIColor { @nonobjc required public convenience init(colorLiteralRed red: Float, green: Float, blue: Float, alpha: Float) } // USAGE : #colorLiteral(red: 1.0, green: 1.0, blue: 1.0, alpha: 1.0)

Kirill Druzhynin
Python Web Development Techdegree Student 4,874 PointsSwift 4 DevDocs knows nothing about literalColor. I think they dropped this thing. You should you displayP3 instead of colorLiteral and CGFloat instead of Float. Here is the code that works great for me:
enum ColorComponent {
case rgb(red: CGFloat, green: CGFloat, blue: CGFloat, alpha: CGFloat)
case hsb(hue: CGFloat, saturation: CGFloat, brightness: CGFloat, alpha: CGFloat)
func color() -> UIColor {
switch self {
case .rgb(let red, let blue, let green, let alpha):
return UIColor(displayP3Red: red/255.0, green: green/255.0, blue: blue/255.0, alpha: alpha)
case .hsb(let hue, let saturation, let brightness, let alpha):
return UIColor(hue: hue/360.0, saturation: saturation/100.0, brightness: brightness/100.0, alpha: alpha)
}
}
}
ColorComponent.rgb(red: 61.0, green: 120.0, blue: 198.0, alpha: 1.0).color()
ColorComponent.hsb(hue: 61.0, saturation: 120.0, brightness: 198.0, alpha: 1.0).color()

Linus Karlsson
7,402 PointsGreat comment, much obliged!
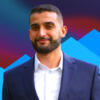
Ahmad Ibrahim
Full Stack JavaScript Techdegree Graduate 26,074 PointsThis worked for me Thanks!
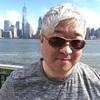
Raymond Choy
iOS Development with Swift Techdegree Graduate 11,187 PointsKirill Druzhynin! As of July 22, 2019 Swapping out Pasan's outdate wrong syntax "colorLiteralRed" and replacing it with your correct updated syntax "displayP3Red" and "CGFloat" instead of "Float" fixed all the error messages. Thank you!!!!!!!! This was driving me crazy. I thought they would have a ding sound when something they were teaching us changed in the latest version of Swift. smh

apbaca06
4,637 Pointsstill having trouble with it.... can somebody help?
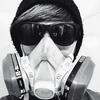
Gavin Hobbs
5,205 Pointsapbaca06 I'm stil having trouble too! Here's my code...
enum ColorComponent {
case rgb(CGFloat, CGFloat, CGFloat, CGFloat)
case hsb(CGFloat, CGFloat, CGFloat, CGFloat)
func color() -> UIColor {
switch self {
case .rgb(let red, let green, let blue, let alpha):
return UIColor(red: red/255.0, green: green/255.0, blue: blue/255.0, alpha: alpha))
case .hsb(let hue, let saturation, let brightness, let alpha):
return UIColor(hue: hue/36.0, saturation: saturation/100.0, brightness: brightness/100.0, alpha: alpha)
}
}
}
ColorComponent.rgb(61.0, 120.0, 198.0, 1.0).color()
I've also tried the displayP3
method too as Jonatan Witoszek mentioned and I still get the same error as I'm getting with the code above.
Here's my errors...
Playground execution failed:
error: EnumerationsOptional.playground:63:95: error: consecutive statements on a line must be separated by ';'
return UIColor(red: red/255.0, green: green/255.0, blue: blue/255.0, alpha: alpha))
^
;
error: EnumerationsOptional.playground:63:95: error: expected expression
return UIColor(red: red/255.0, green: green/255.0, blue: blue/255.0, alpha: alpha))
^

Nico Schmidt
12,781 Pointsimport UIKit
enum ColorComponent{
case rgb(Float,Float,Float,Float) // <- Here was a semicolon too much
func color()->UIColor{
switch self{
case .rgb(let red, let green, let blue, let alpha):
return UIColor(colorLiteralRed:red/255.0,green:green/255.0,blue:blue/255.0,alpha: alpha)
}
}
}
ColorComponent.rgb(61,120,128,1.0).color()

Prem Agrawal
117 PointsSemiColons are used to separate out different lines of code from each other although i copy pasted your code but the same error popped up i think its because of the UIColor(colorLiteralRed) as it may not exist in the newer version of xcode........and how do u make our codes background black here..???
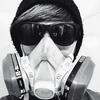
Gavin Hobbs
5,205 PointsPrem Agrawal, you can make your code black if you check out the Markdown Cheetsheet...
Markdown Cheatsheet Markdown is a short-hand syntax for easily converting text to HTML. Below are some popular examples of Markdown formatting. For more examples reference Markdown Basics for a more detailed overview.
Code Wrap your code with 3 backticks (```) on the line before and after. If you specify the language after the first set of backticks, that'll help us with syntax highlighting.
```html
<p>This is code!</p>
```
Additionally to make things bold add two asterisks to the front and the end of what you want bold and just one asterisk for italics

Nico Schmidt
12,781 PointsIt does work for me on the newest version. Did you import UIKit?

Prem Agrawal
117 PointsYes i have imported UIKit but still it does not work for me i am having Xcode version 9.0 beta 5

Prem Agrawal
117 Pointsimport UIKit
enum ColorComponent{ case rgb(CGFloat,CGFloat,CGFloat,CGFloat) // <- Here was a semicolon too much func color()->UIColor{ switch self{ case .rgb(let red, let green, let blue, let alpha): return UIColor(red: red,green: green,blue: blue,alpha: alpha) } } } ColorComponent.rgb(61,120,128,1.0).color()
Now this code does worked for me as i was expecting the problem was with colorLiteralRed which was nowhere present in Developers Documentation of my Xcode so i went there and checked for UIColor and now its working , If anyone had same problem this is the code you are looking for.
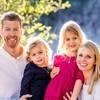
codyl
4,704 PointsSo in other words, this video has been wrong for about a year and a half, and they've not updated it.
Come on Pasan Premaratne I only get so much time to do these lessons. When stuff like this happens it takes me longer to trouble shoot to figure out why it's not working for me than I spend on the lessons.

josh kinney
1,576 PointsAgree this needs to be addressed. I pay for these lessons and I sure hope they would keep them updated
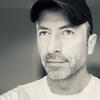
bytepunch
iOS Development with Swift Techdegree Student 5,114 PointsXCode 10.3 (Swift 5) compiler told me this:
return UIColor(_colorLiteralRed: red/255.0, green: green/255.0, blue: blue/255.0, alpha: alpha)
Needs just an underscore.