Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial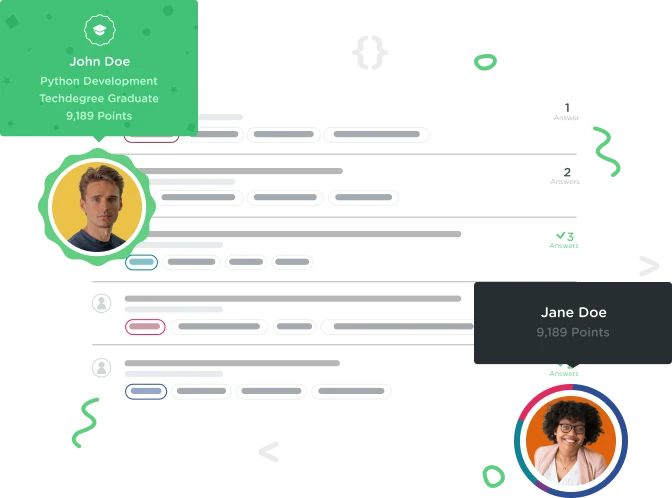
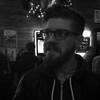
Forrest Olson
16,661 PointsError: "events.js:141 throw er; // Unhandled 'error' event"
This code worked perfectly when I ran it in Workspaces. When I ran "node app.js chalkers" on Workspaces it returned the error message just fine. However, I copied the code, pasted it into Atom, and ran it on my local machine and I got this error: "events.js:141 throw er; // Unhandled 'error' event"
Here's my code:
"use strict"
const https = require('https');
const printMessage = (username, badges, points) => {
console.log(`${username} has ${badges} badges and ${points} points in Javascript!`);
}
function getProfile(username) {
try {
const request = https.get(`teamtreehouse.com/${username}.json`, response => {
// variable to hold string of data
let body = "";
// when we receive data
response.on('data', data => {
//turn that shit into a string
body += data.toString();
});
// after the data's been received:
response.on('end', () => {
// parse the data that has been housed in the body variable
const json = JSON.parse(body);
printMessage(username, json.badges.length, json.points.JavaScript);
});
});
} catch (error) {
console.error(error.message);
}
}
const users = process.argv.slice(2);
users.forEach(getProfile);
Why am I receiving this "throw er" error on my local machine instead of the error.message in the catch statement?
1 Answer

Robert Stewart
11,921 PointsI just tried it with node 11.11 and it correctly throws the error "Invalid URL: teamtreehouse.com/chalkers.json" so my question is what version of node are you using on your local machine? I would recommend reinstalling/updating your node.js installation.
You can tell which version you have by running: node --version
As a side note, events.js:141 tells you that it isn't in your code but somewhere further inside of node.js which I'm guessing means that you're trying to use functionality that isn't supported by the version of node that is installed on your machine.
Forrest Olson
16,661 PointsForrest Olson
16,661 PointsYep, that was it!
Appreciate the note about the "events.js:141" part of the error message, I was wondering where that file was. Thank you!