Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial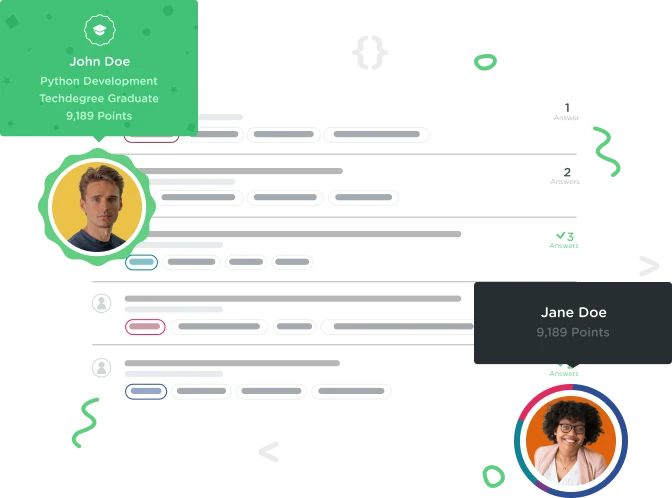

Trevor Hunsberger
1,509 PointsError: Expected 11 words, but received 12.
I'm trying to use the split() method to return an array of Strings from a String, but I'm getting the following error: "Expected 11 words, but received 12." So it would seem that the method is working correctly but I've got no idea where this extra word in the array is coming from. Any ideas on what the problem is?
package com.example;
import java.util.Date;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
public String[] getWords() {
return mBody.split("[\\s+]");
}
}

K Cleveland
21,839 PointsIt would help to trouble shoot this if I knew the string I was trying to split, but it's not given.
This is actually good thinking on your part. You're wondering -- what is it that they're checking? When you make programs, a lot of times, you won't know what the user is passing in or checking. Which is why we have to make sure that our programs do what they say they will do regardless of if we know what's being passed in.
I suggest looking at this part of your code in particular:
"[\\s+]"
Is this the proper syntax for what you want? Since we know that you're not passing that challenge, something is off. Look up what "+" does. You're very close. Good luck! :)

Trevor Hunsberger
1,509 PointsThe "+" tells the method to look for "one or more" of the "\s" or whitespace characters. The error above was in having the "+" sign inside the square brackets with the CharSequence.
1 Answer

K Cleveland
21,839 Pointsreturn mBody.split("[\\s+]");
Double check your syntax here. If you check out the video again, you should be able to spot it. Good luck!

Trevor Hunsberger
1,509 PointsAh, I had the plus sign inside the square brackets, instead of outside of it. Thanks Keaunna Cleveland!
Note: I also got it to pass like this:
return mBody.split("\\s+");
which confused me even further for moment, but from what I can tell this is how you might pass a single character argument?
Trevor Hunsberger
1,509 PointsTrevor Hunsberger
1,509 PointsIt would help to trouble shoot this if I knew the string I was trying to split, but it's not given. I tried adding a main and System.out.println() the getWords method, but I can't call the reference variable of the BlogPost object because it is also not given.