Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial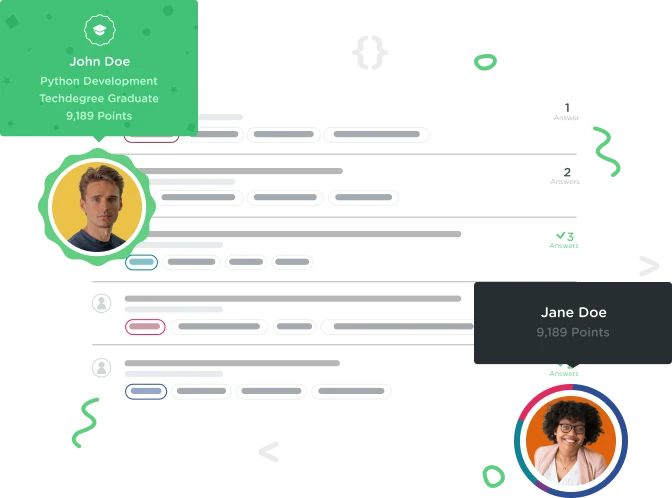

kenikeako
1,785 PointsError getting the image resource for the Icon for the IconImageView in the Dayadapter class.
I am getting this error when trying to set the iconImageView on the DayAdapter class.
java.lang.NullPointerException: Attempt to invoke virtual method 'void android.widget.ImageView.setImageResource(int)' on a null object reference
Day day = mDays[position]; //This is the offending code -------> holder.iconImageView.setImageResource(day.getIconId()); holder.temperatureTextView.setText(day.getmTemperatureMax()+ ""); holder.dayNameLabel.setText(day.getDayofTheWeek());
I day of the week and the temperaturemax display correctly. I am not sure what I have wrong in my code. Here are the getters and setters for the current, Daily, and forcast classes.
package com.celebs.ikeak.practiceweather10242017.weather;
import com.celebs.ikeak.practiceweather10242017.ForeCast; import com.celebs.ikeak.practiceweather10242017.R;
import java.sql.Date; import java.sql.Time; import java.text.SimpleDateFormat;
/** * */ public class current {
private String Icon;
private long time;
private double temperature;
private double humidity;
private double percipChance;
private String Summary;
private String TimeZone;
public String getIcon() {
return Icon;
}
public void setIcon(String icon) {
Icon = icon;
}
public int getIconId () {
return ForeCast.getIconId(Icon);
}
public long getTime() {
return time;
}
public void setTime(long time) {
this.time = time;
}
//We need to set the time with the timezone in mind
public String getFormattedTime() {
//This gets the format the time should be from the timezone
SimpleDateFormat formatter = new SimpleDateFormat("h:mm a");
formatter.setTimeZone(java.util.TimeZone.getTimeZone(TimeZone));
//This gets the time
Date dateTime = new Date((getTime()* 1000));
String timeString = formatter.format(dateTime);
return timeString;
}
public String getTimeZone() {
return TimeZone;
}
public void setTimeZone(String timeZone) {
TimeZone = timeZone;
}
public int getTemperature() {
return (int) Math.round (temperature);
}
public void setTemperature(double temperature) {
this.temperature = temperature;
}
public double getHumidity() {
return humidity;
}
public void setHumidity(double humidity) {
this.humidity = humidity;
}
//percipitation chance
public int getPercipChance() {
double percipitationChance = percipChance * 100;
return (int) Math.round(percipitationChance);
}
public void setPercipChance(double percipChance) {
this.percipChance = percipChance;
}
public String getSummary() {
return Summary;
}
public void setSummary(String summary) {
Summary = summary;
}
}
package com.celebs.ikeak.practiceweather10242017.weather;
import android.os.Parcel; import android.os.Parcelable;
import com.celebs.ikeak.practiceweather10242017.ForeCast;
import java.text.SimpleDateFormat; import java.util.Date; import java.util.TimeZone;
/**
- Created by ikeak on 10/28/2017. */
//Implement an interface public class Day implements Parcelable {
private long mTime;
private String mSummary;
private double mTemperatureMax;
private String mIcon;
private String mTimeZone;
public Day() {
}
public long getmTime() {
return mTime;
}
public void setmTime(long mTime) {
this.mTime = mTime;
}
public String getmSummary() {
return mSummary;
}
public void setmSummary(String mSummary) {
this.mSummary = mSummary;
}
public int getmTemperatureMax() {
return (int) Math.round(mTemperatureMax);
}
public void setmTemperatureMax(double mTemperatureMax) {
this.mTemperatureMax = mTemperatureMax;
}
public String getmTimeZone() {
return mTimeZone;
}
public void setmTimeZone(String mTimeZone) {
this.mTimeZone = mTimeZone;
}
public int getIconId() {
return ForeCast.getIconId(mIcon);
}
public String getmIcon() {
return mIcon;
}
public void setmIcon(String mIcon) {
this.mIcon = mIcon;
}
public String getDayofTheWeek() {
SimpleDateFormat formatter = new SimpleDateFormat("EEEE");
formatter.setTimeZone(TimeZone.getTimeZone(getmTimeZone()));
Date dateTime = new Date(mTime * 1000);
return formatter.format(dateTime);
}
@Override
public int describeContents() {
return 0;
}
//Writetoparcel wraps the data up.
@Override
public void writeToParcel(Parcel dest, int flags) {
dest.writeLong(mTime);
dest.writeString(mSummary);
dest.writeDouble(mTemperatureMax);
dest.writeString(mIcon);
dest.writeString(mTimeZone);
}
//Unwraps the data
private Day(Parcel in) {
mTime = in.readLong();
mSummary = in.readString();
mTemperatureMax = in.readDouble();
mIcon = in.readString();
mTimeZone = in.readString();
}
public static final Creator<Day> CREATOR = new Creator<Day>() {
@Override
public Day createFromParcel(Parcel source) {
return new Day(source);
}
@Override
public Day[] newArray(int size) {
return new Day[size];
}
};
}
package com.celebs.ikeak.practiceweather10242017;
import com.celebs.ikeak.practiceweather10242017.weather.Day; import com.celebs.ikeak.practiceweather10242017.weather.Hour; import com.celebs.ikeak.practiceweather10242017.weather.current;
/**
-
Created by ikeak on 10/28/2017. */ public class ForeCast {
private current mCurrent; private Hour[] mHourlyForecast; private Day[] mDailyForecast;
public current getmCurrent() { return mCurrent; }
public void setmCurrent(current mCurrent) { this.mCurrent = mCurrent; }
public Hour[] getmHourlyForecast() { return mHourlyForecast; }
public void setmHourlyForecast(Hour[] mHourlyForecast) { this.mHourlyForecast = mHourlyForecast; }
public Day[] getmDailyForecast() { return mDailyForecast; }
public void setmDailyForecast(Day[] mDailyForecast) { this.mDailyForecast = mDailyForecast; }
public static int getIconId(String iconString) {
int iconId = R.drawable.clear_day; if(iconString.equals("clear-day")) { iconId = R.drawable.clear_day; } else if(iconString.equals("clear-night")) { iconId = R.drawable.clear_night; } else if (iconString.equals("rain")) { iconId = R.drawable.rain; } else if (iconString.equals("snow")) { iconId = R.drawable.snow; } else if (iconString.equals("sleet")) { iconId = R.drawable.sleet; } else if (iconString.equals("wind")) { iconId = R.drawable.wind; } else if (iconString.equals("fog")) { iconId = R.drawable.fog; } else if (iconString.equals("cloudy")) { iconId = R.drawable.cloudy; } else if (iconString.equals("partly-cloudy-day")) { iconId = R.drawable.partly_cloudy; } else if (iconString.equals("partly-cloudy-night")) { iconId = R.drawable.cloudy_night; } return iconId;
}
}
1 Answer

Seth Kroger
56,413 PointsThe message java.lang.NullPointerException: Attempt to invoke virtual method 'void android.widget.ImageView.setImageResource(int)' on a null object reference
is saying that the View is null, indicating the problem is in the Adapter when you set the View in the holder (or the XML for the list items).