Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial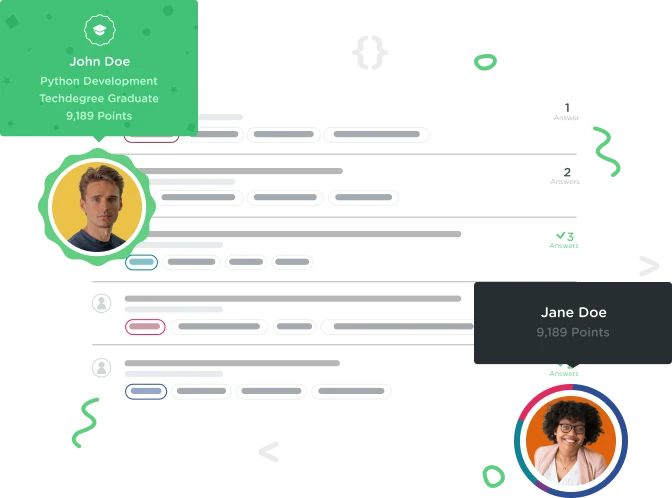

Ryan Forrester
Courses Plus Student 4,052 PointsError getting user JSON
Everything works fine on the home page. No errors in terminal either. When I enter a username "chalkers" in the url I do not get any data. There are no visible errors so I console.logged the variable username
right after the line:
var studentProfile = new Profile(username);
console.log("This is the username",username);
When I goto the URL/chalkers my console.log outputs to terminal two username calls:
This is the username chalkers
This is the username favicon.ico
Any reason why this call is happening twice? Below is my router.js
var Profile = require("./profile.js");
// Handle HTTP route GET / and POST / i.e. Home
function home(request, response){
// if url == "/" && GET
if(request.url == "/"){
// show search
response.writeHead(200, {'Content-Type': 'text/plain'});
response.write("Header\n");
response.write("Search\n");
response.end("Footer\n");
}
}
// response.end('Hello World\n');
// IF url == "/" && POST
// redirect to /:username
// Handle HTTP rout GET /:username i.e. /chalkers
function user(request, response){
// if url == "/...."
var username = request.url.replace("/", "");
if(username.length > 0){
response.writeHead(200, {'Content-Type': 'text/plain'});
response.write("Header\n");
// Get JSON from Treehouse
var studentProfile = new Profile(username);
console.log("This is the username",username);
// on "end"
studentProfile.on("end", function(profileJSON){
// show PROFILE
// Store the values which we need
var values = {
avatarUrl: profileJSON.gravatar_url,
username: profileJSON.profile_name,
badges: profileJSON.badges.length,
javascriptPoints: profileJSON.JavaScript
}
// Simple response
response.write(values.username + " has " + values.badges + " badges.\n");
response.end("Footer\n");
});
// on "error"
studentProfile.on("error", function(errorObj){
// show error
response.end('Footer\n');
});
response.end("Footer\n");
}
}
module.exports.home = home;
module.exports.user = user;
3 Answers

Chris Anderson
2,879 PointsSorry - me again! Cracked it with the proper solution. The quick and dirty method did give an error in the Chrome console later but didn't stop the server but this is the way to do it properly (I think) to end the favicon request and allow you to get on with coding. Code snippet here:
//3. Handle http route for GET /:username i.e. chrisanderson8
function user(request, response) {
//if the URL == "/...." (anything)
var username = request.url.replace("/", "");
//This IF statement is the fix
if (username === 'favicon.ico') {
response.writeHead(200, {'Content-Type': 'image/x-icon'} );
response.end();
console.log('favicon requested'); //checking to see if this IF statement was called
return;
}
//Now the favicon request has been dealt with, we can carry on as normal with the same code. No need to check the URL
if(username.length > 0) {
response.writeHead(200, {'content_type': 'text/plain'});
response.write("Header\n");
//get json from Treehouse
var studentProfile = new Profile(username);
console.log(username); //checking the username in the console
//on "end"
studentProfile.on("end", function(profileJSON){
//show profile
Thanks!
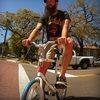
george birnbaum
5,652 PointsSeeing the same issue. Just did a console log of the username, and it's pulling the favicon as a username...

Chris Anderson
2,879 PointsHey,
I'm getting the same problem but have found a quick fix and (hopefully) a proper solution too (but yet to get working).
First the quick fix:
Add in an extra condition to your 'If' statement in the router.js so that it does not process a URL ending in favicon.ico. It does mean the call is still made but the code doesn't process it, so you don't get the error and can carry on with the tutorial. Code snippet below.
if(username.length > 0 && username !== 'favicon.ico' ) {
response.writeHead(200, {'content_type': 'text/plain'});
response.write("Header\n");
//get json from Treehouse
var studentProfile = new Profile(username);
console.log(username);
//on "end"
studentProfile.on("end", function(profileJSON){
//show profile
For the longer fix:
It turns out (from a bit of Googling) that this is a common problem (if problem is the correct word here) within Node where this additional call is made to get the favicon. So some people have tried to solve it using the below. Source here. I've modified the code from the source and displayed it below to use the naming conventions within our App. However, being a complete novice I couldn't implement this in a way which solved the problem, so I'm using the quick and dirty method above for now. I thought I'd include this in case you could use it.
if (request.url === 'favicon.ico') {
response.writeHead(200, {'Content-Type': 'image/x-icon'} );
response.end();
console.log('favicon requested');
return;
}
Good luck!
Patrick O'Dacre
15,471 PointsPatrick O'Dacre
15,471 PointsThanks, Chris!
Great work. I learned a lot from your solution and explanation.
Cheers