Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial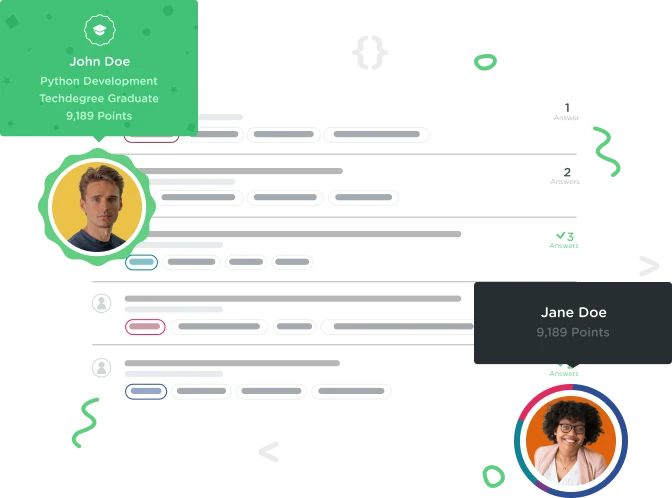

jc laurent
6,351 Pointserror handling
"Make sure you're calling the parse method!" I thought I had....
enum ParserError: ErrorType {
case EmptyDictionary(String)
case InvalidKey(String)
}
struct Parser {
var data: [String : String?]?
func parse() throws {
if let someDict = data {
if let someData = someDict["someKey"] {
} else { throw ParserError.InvalidKey("No response") }
} else { throw ParserError.EmptyDictionary("Unknown")
}
}
}
let data: [String : String?]? = ["someKey": nil]
let parser = Parser(data: data)
do {
let result = try parse(data, parser)
} catch ParserError.EmptyDictionary(let key) {
} catch ParserError.InvalidKey(let dat) {
}
11 Answers

Moritz Lang
25,909 PointsHi,
why do you add an associated type to the parser error enum? I think that's much more than the challenge asks for. Also please make sure to always add a generic catch block, so that you app knows what to do if none of the specific catch blocks get called. In my solution I use a guard let statement
, but feel free to go with your if let statement
instead, if you preferr.
enum ParserError: ErrorType {
case EmptyDictionary
case InvalidKey
}
struct Parser {
var data: [String : String?]?
func parse() throws {
guard let data = self.data else { throw ParserError.EmptyDictionary }
guard data.keys.contains("someKey") else { throw ParserError.InvalidKey }
}
}
let data: [String : String?]? = ["someKey": nil]
let parser = Parser(data: data)
do {
try parser.parse()
} catch ParserError.EmptyDictionary {
print("The dictionary is empty.")
} catch ParserError.InvalidKey {
print("Invalid key.")
} catch {
print("Fuck.")
}

jc laurent
6,351 Pointswell the fact is I'm following the course and the examples given that's why I got stuck! thks Moritz

Moritz Lang
25,909 PointsMaybe you have to slown down and really try to understand the "why". I think it's a longer learning path if you just try to pass all challenges as fast as you can and 3 days later don't know how to solve it anymore.

jc laurent
6,351 Pointssure

jc laurent
6,351 Pointsany method to propose perhaps?

Moritz Lang
25,909 PointsYou mean any help on how to learn iOS development?

jc laurent
6,351 Pointsabsolutely

Moritz Lang
25,909 PointsWell, that depends. :) For me blogging helps a lot. And if I'm not 100% sure about what's taught in a video I simply watch it again.

jc laurent
6,351 PointsWhich blogs?

jc laurent
6,351 PointsThks Moritz
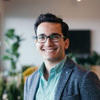
Baran Ozkan
8,818 PointsMy Xcode compiler is forcing me to unwrap data.keys.contains("someKey") using a '!' or '?' although I'm already using guard statement for unwrapping. Does this have to do with version changes or what's going on? Thanks in advance!
Full code below.
'''
enum ParserError: Error { case emptyDictionary case invalidKey }
struct Parser { var data: [String : String?]?
func parse() throws {
guard let data1 = data else {
throw ParserError.emptyDictionary
}
guard data.keys.contains("someKey") else {
throw ParserError.invalidKey
}
}
}
let data: [String : String?]? = ["someKey": nil] let parser = Parser(data: data)
do { try parser.parse() } catch ParserError.emptyDictionary { print("Empty Dictionary") } catch ParserError.invalidKey { print("Invalid Key") }
'''