Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial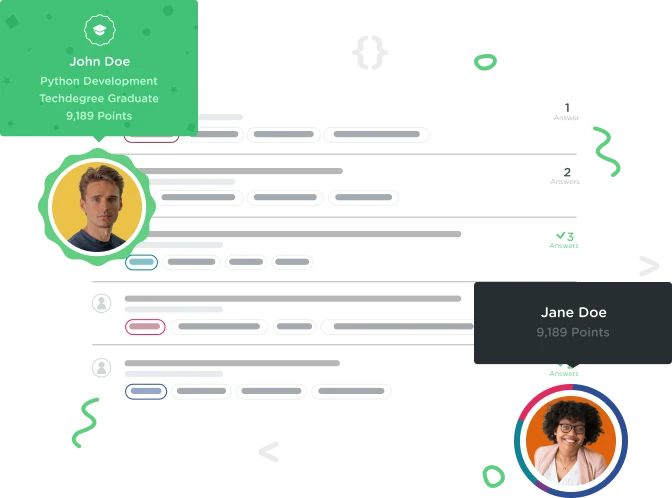

Zimri Leijen
11,835 PointsError handling for invalid JSON submitted
When I POST invalid JSON I don't get the usual error messages, neither 400 nor 500, how do you catch invalid JSON, and why doesn't the try...catch block catch this?
It does get a status message of 400 so it seems some of the middleware throws another error.
3 Answers

Simon Coates
8,377 PointsIn the event this is useful in the future, a 400 might be enough for whatever is consuming the API. I'm no expert, but adding global error handler middleware could catch the error (and any others). It might also be possible to write your own json parser middleware if you needed to respond specifically to malformed json.

Trevor Maltbie
Full Stack JavaScript Techdegree Graduate 17,021 PointsGood question....
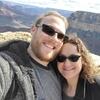
James Crosslin
Full Stack JavaScript Techdegree Graduate 16,882 PointsI liked this question, so I coded out a possible way to handle some of these errors along with more description to the client!
const { response } = require("express")
const express = require("express")
const records = require("./records")
const app = express()
app.use(express.json())
//CRUD - Create Read Update Delete
//Send a Get request to /quotes to Read a list of quotes\
app.get("/quotes", async (req, res, next) => {
try {
const quotes = await records.getQuotes()
res.json(quotes)
} catch (err) {
err.status = 400
return next(err)
}
})
//Send a Get request to /quotes/:id toRead a quote
app.get("/quotes/:id", async (req, res, next) => {
try {
const quote = await records.getQuote(+req.params.id)
if (!quote) throw new Error("Quote not found")
res.json(quote)
} catch (err) {
err.status = 404
return next(err)
}
})
//Send a Post request to /quotes to Create a quote
app.post("/quotes", async (req, res, next) => {
try {
const { author, quote } = req.body
if (author && quote) {
const newQuote = await records.createQuote({ quote, author })
res.status(201).json(newQuote)
} else {
const missing = author ? "Quote" : "Author"
throw new Error(
`${missing} not included: must include both an author and and a quote`
)
}
} catch (err) {
err.status = 400
return next(err)
}
})
//Send a Put request to /quotes/:id to Update a quote
//Send a Delete request to /quotes/:id to Delete a quote
//Send a Get request to /quotes/random to Read a random quote
app.use((req, res, next) => {
const err = new Error("Not Found")
err.status = 404
next(err)
})
app.use((err, req, res, next) => {
res.status(err.status || 500).json({ message: err.message })
})
let port = process.env.PORT ?? 3000
app.listen(port, () => console.log(`Quote API listening on port ${port}!`))