Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial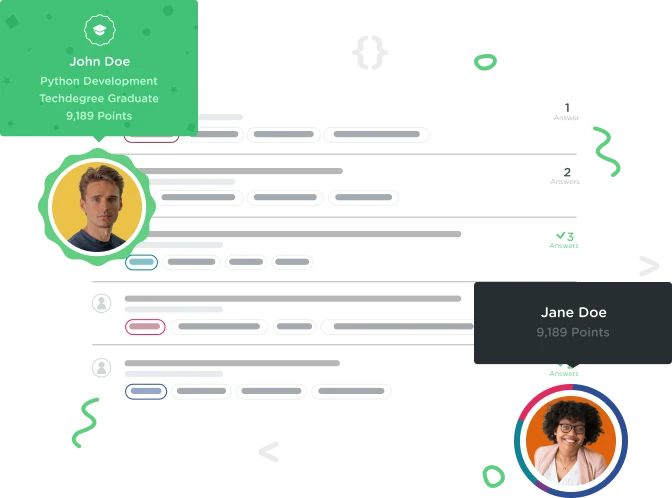

Collin Cannavo
2,494 PointsError Handling: I'm not getting errors in xCode and the preview isn't showing any issues, but it's still not completing
In the editor, you have a struct named Parser whose job is to deconstruct information from the web. The incoming data can be nil, keys may not exist, and values might be nil as well. In this task, complete the body of the parse function. If the data is nil, throw the EmptyDictionary error. If the key "someKey" doesn't exist throw the InvalidKey error. Hint: To get a list of keys in a dictionary you can use the keys property which returns an array. Use the contains method on it to check if the value exists in the array
enum ParserError: Error {
case EmptyDictionary
case InvalidKey
}
struct Parser {
var data: [String : String?]?
func parse() throws {
guard let parsedData = data else {
throw ParserError.EmptyDictionary
}
guard parsedData.keys.contains("someKey") else {
throw ParserError.InvalidKey
}
}
}
let data: [String : String?]? = ["someKey": nil]
do {
let parser = try Parser(data: data).parse()
} catch let error {
print(error)
}
3 Answers
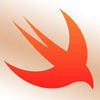
Jeff McDivitt
23,970 PointsHi Collin -
This is how I completed this challenge. You are on the right track you just needed to go a little farther
enum ParserError: Error {
case emptyDictionary
case invalidKey
}
struct Parser {
var data: [String : String?]?
func parse() throws {
guard let data = data else {
throw ParserError.emptyDictionary
}
guard data["somekey"] != nil else {
throw ParserError.invalidKey
}
}
}
let data: [String : String?]? = ["someKey": nil]
let parser = Parser(data: data)
do {
let parser = try Parser(data: data)
try parser.parse()
} catch ParserError.emptyDictionary(let description) {
print(description)
}
catch ParserError.invalidKey(let description) {
print(description)
}
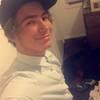
Jordan Ward
2,395 PointsHey Jeff I dont mean to be rude, and I'm sure your much more advanced than me but doesn't it say "For this task, just use a generic catch block rather than pattern matching on specific errors". in that case shouldn't you just create a do catch block like..
do { let parser = try Parser(data: data) try parser.parse() } catch let error { print(error) }

Collin Cannavo
2,494 PointsThat's the problem. It's not giving me an error. It says "Your code could not be compiled. Please click on "Preview" to view the compiler errors.", but when I click on the Preview button it's blank; it doesn't show any errors.
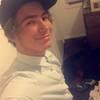
Jordan Ward
2,395 PointsOh never mind thats part 3 lol
Jeff McDivitt
23,970 PointsJeff McDivitt
23,970 PointsWhat is the exact error that you are receiving when you attempt to run?