Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial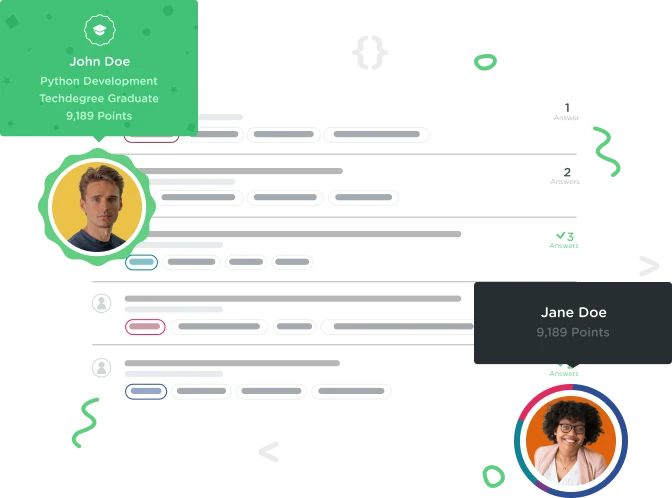

Jack Baer
5,049 PointsError Handling in Swift 3 Handling Errors Challenge Trouble
Hello! I've been stuck on this challenge for a while, and would like some help. The error I'm getting is that it is not being unwrapped. I would appreciate any help. Thanks!
enum ParserError: Error {
case emptyDictionary
case invalidKey
}
struct Parser {
var data: [String : String?]?
func parse() throws {
guard let tempVar = data else { throw ParserError.emptyDictionary }
print(" ")
guard let tempVarTwo = data["someKey"] else { throw ParserError.invalidKey }
print(" ")
}
}
let data: [String : String?]? = ["someKey": nil]
let parser = Parser(data: data)
3 Answers
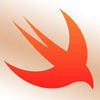
Jeff McDivitt
23,970 PointsNo need to create the temp variables. Eliminate that and you will be fine
enum ParserError: Error {
case emptyDictionary
case invalidKey
}
struct Parser {
var data: [String : String?]?
func parse() throws {
guard let data = data else {
throw ParserError.emptyDictionary
}
guard data["somekey"] != nil else {
throw ParserError.invalidKey
}
}
}
let data: [String : String?]? = ["someKey": nil]
let parser = Parser(data: data)

Jack Baer
5,049 PointsOh, alright. Thanks!

Adesh Balyan
2,217 PointsYou can do this with temp as well. Another way of doing the same thing with for statement which later in future you can use with switch also to match with multiple patterns.
enum ParserError: Error { case emptyDictionary case invalidKey }
struct Parser { var data: [String : String?]?
func parse() throws {
guard let someData = data else {
throw ParserError.emptyDictionary
}
for keys in someData.keys {
if keys != "someKey" {
throw ParserError.invalidKey
}
}
} }
let data: [String : String?]? = ["someKey": nil] let parser = Parser(data: data)
do {
try parser.parse()
} catch ParserError.emptyDictionary {
print(ParserError.emptyDictionary)
} catch ParserError.invalidKey {
print(ParserError.invalidKey)
}
Jenny Dogan
4,595 PointsJenny Dogan
4,595 PointsWhat is the logic behind guard let data = data? are we assigning the data value to itself? And for the second guard statement we're just unwrapping the optional and check to see if it's nil or not without assigning to a constant? Compiler said not to create a constant that won't be used so we're basically just running a method but not assigning the results to a constant? Thanks!
Jeff McDivitt
23,970 PointsJeff McDivitt
23,970 PointsYou are assigning a variable data to the data optional dictionary. In the second part if the the data is not equal nil it parses the data but if it is nil then is throws a Parser Error. For the third question you are correct. Let me know if that helps!