Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial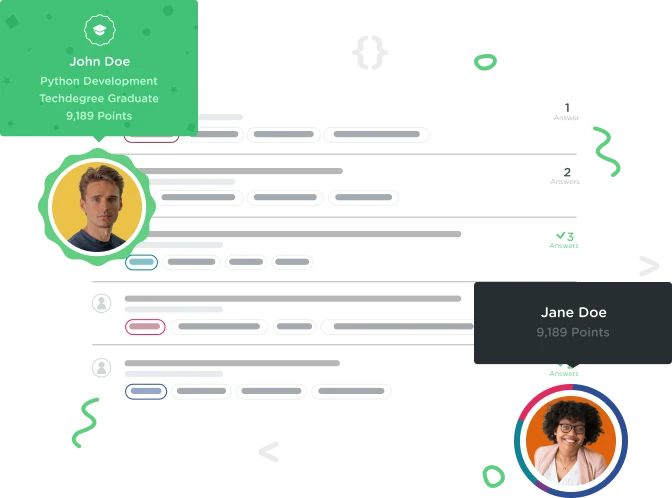
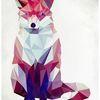
Nick Gaudio
Python Web Development Techdegree Student 2,133 PointsError Handling on reverse number game
So I decided to take ol' Ken Love's advice and write the reverse version of the number game where I know the number and the computer is guessing. I also wrote this originally before watching the video on how Ken did it. I'm sure it might not be as pretty but it works! Below is my code:
Import Random module/library
import random
def game():
#Guesses for the computer
guesses_left = 5
#Set the range for the computer to guess
lower_bound = 1
upper_bound = 10
while guesses_left:
ran_num = random.randint(lower_bound,upper_bound)
#Make the computer Guess
print("The computer's guess is {}.".format(ran_num))
my_response = input("Is this the correct number? (y) or is it 'Higher' or 'Lower'?")
if my_response.lower() == "higher":
guesses_left -= 1
print("You have {} guesses left, computer.".format(guesses_left))
lower_bound = ran_num + 1
print("")
elif my_response.lower() == "lower":
guesses_left -= 1
print("You have {} guesses left, computer.".format(guesses_left))
upper_bound = ran_num - 1
print("")
elif my_response.lower() == "y":
print("That's the correct number!")
break
else:
print("Man over Machine.")
game()
Everything works just fine. I just want to know how I can error handle for when I decide to be a jerk to the computer and set the upper bound (through my responses of higher and lower) below the lower bound on the random.randint() function.
e.g. lower_bound = 5 upper_bound = 4 random.randint(lower_bound, upper_bound) 5 4 This throws an error since 4 is lower than 5.
This obviously wouldn't happen if I play the game fairly and don't change my number but I'm just curious.
2 Answers
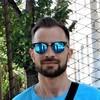
Oszkár Fehér
Treehouse Project ReviewerHi Nick Interesting idea.One approach would be a method which handles this. The second something like this:
if lower_bound > upper_bound:
ran_num = random.randint(upper_bound, lower_bound)
else:
ran_num = random.randint(upper_bound, lower_bound)
The method would be something similar:
def generator():
if lower_bound > upper_bound:
return random.randint(upper_bound, lower_bound)
else:
return random.randint(upper_bound, lower_bound)
and when you call:
ran_num = generator()
But this is just my idea. Keep up the good work!
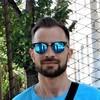
Oszkár Fehér
Treehouse Project ReviewerHi Nick I apologize because i did a mistake but is fixable
def generator():
if lower_bound > upper_bound:
return random.randint(upper_bound, lower_bound)
else:
return random.randint(lower_bound, upper_bound)
and also with the if statement i forgot to change the order of the variables but i think you got the idea. Happy coding