Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial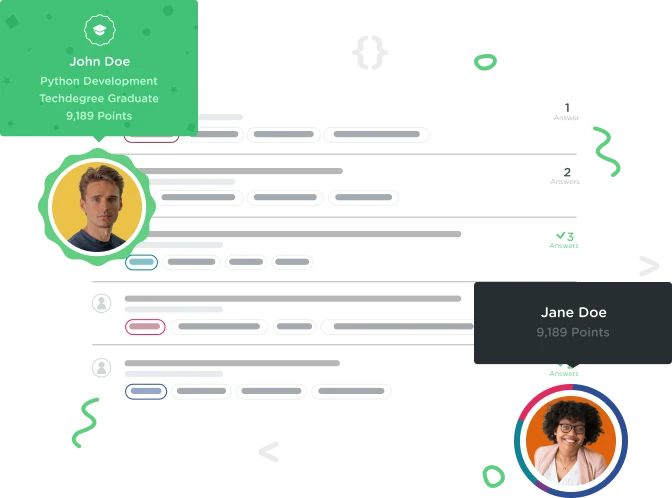
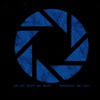
James N
17,864 Pointserror: illegal start of expression
hi, I am getting errors in my code. I don't know what's wrong with it. can someone please help??
public class Hangman {
public static void main(String[] args) {
// Enter amazing code here:
Game game = new Game("Treehouse");
Prompter prompter = new Prompter(game);
boolean isHit = prompter.promptForGuess();
if (isHit) {
System.out.println("We Got A Hit!");
} else {
System.out.println("Oops, that was a miss");
}
game.displayProgress();
}
}
public class Game {
private String mAnswer;
private String mHits;
private String mMisses;
public Game(String answer) {
mAnswer = answer;
mHits = "";
mMisses = "";
}
public boolean applyGuess(char letter) {
boolean isHit = mAnswer.indexOf(letter) >= 0;
if (isHit) {
mHits += letter;
} else {
mMisses += letter;
}
return isHit;
}
public String getCurrentProgress() {
String progress = "";
for (char letter : mAnswer.toCharArray()) {
char display = "_";
if (mHits.indexOf(letter) >= 0) {
display = letter;
}
progress += display;
return progress;
}
public void displayProgress() {
System.out.printf("Try to solve %s\n",mGame.getCurrentProgress());
}
}
}
import java.io.Console;
public class Prompter {
private Game mGame;
public Prompter(Game game) {
mGame = game;
}
public boolean promptForGuess() {
Console console = System.console();
String guessAsString = console.readLine("Enter a letter: ");
char guess = guessAsString.charAt(0);
return mGame.applyGuess(guess);
}
}
./Game.java:32: error: illegal start of expression
public void displayProgress() {
^
./Game.java:32: error: illegal start of expression
public void displayProgress() {
^
./Game.java:32: error: ';' expected
public void displayProgress() {
^
Hangman.java:13: error: cannot find symbol
game.displayProgress();
^
symbol: method displayProgress()
location: variable game of type Game
./Game.java:25: error: incompatible types: String cannot be converted to char
char display = "_";
^
./Game.java:33: error: cannot find symbol
System.out.printf("Try to solve %s\n",mGame.getCurrentProgress());
^
symbol: variable mGame
location: class Game
6 errors
2 Answers

Oron Ben Zvi
14,041 PointsHi, first of all you have six errors, so let's tackle them one by groups.
1,2,3: it appears you've put displayProgress method inside another method (getCurrentProgress). you should get it out of there and put it in the other methods level.
so instead of this:
public String getCurrentProgress() {
String progress = "";
for (char letter : mAnswer.toCharArray()) {
char display = "_";
if (mHits.indexOf(letter) >= 0) {
display = letter;
}
progress += display;
return progress;
}
public void displayProgress() {
System.out.printf("Try to solve %s\n",mGame.getCurrentProgress());
}
}
do something like this (look at where the block brackets are):
public String getCurrentProgress() {
String progress = "";
for (char letter : mAnswer.toCharArray()) {
char display = "_";
if (mHits.indexOf(letter) >= 0) {
display = letter;
}
progress += display;
return progress;
}
}
public void displayProgress() {
System.out.printf("Try to solve %s\n",mGame.getCurrentProgress());
}
4: says that displayProgress method cannot be found because of 1,2 and 3 errors.
5: it seems that you're trying to insert a String type to a Char typed variable:
char Display = "_";
try using single quotes like this:
char Display = '_';
6: it appears that you're trying to use mGame member which doesn't really exist. try just using getCurrentProgress(), it'll use that method on the same object that displayProgress() is used on, It's like this.getCurrentProgress() actually.
so after you moved "displayProgress" to the other methods level.
instead of this:
public void displayProgress() {
System.out.printf("Try to solve %s\n",mGame.getCurrentProgress());
}
do this:
public void displayProgress() {
System.out.printf("Try to solve %s\n", getCurrentProgress());
}
or this (with "this" keyword):
public void displayProgress() {
System.out.printf("Try to solve %s\n", this.getCurrentProgress());
}
Let me know if it helped you, or need more help.
Cheers, O
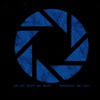
James N
17,864 PointsI am now getting an error that says:
./Game.java:33: error: missing return statement
}
^
1 error
but thanks for the help so far!!!!

Oron Ben Zvi
14,041 PointsIt appears that you're missing a return statement on your getCurrentProgress.
though you do make a
return progress;
inside of your for loop,
you need the make one after and outside the for loop to ensure that a return will always happen anyway.
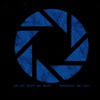
James N
17,864 PointsI will try this, thanks! ill let you know if there's something wrong...
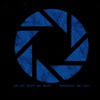
James N
17,864 Pointsthanks for your help! it works!!!

David Spell
899 PointsI am having the same problem. Even when I copied the code from here, I still have errors. What do I do next?
public String getCurrentProgress() { String progress = ""; for (char letter : mAnswer.toCharArray()) { char display = "-"; if (mHits.indexOf(letter) >= 0) { display = letter; } progress += display; return progress; } }
public void displayProgress() {
System.out.printf("Try to solve %s\n",this.getCurrentProgress());
}
}
Nikolay Egov
96 PointsNikolay Egov
96 PointsRegarding the one: char display = "_"; Chars are declared with single quotes so change those to ' I can't figure out the rest yet.