Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial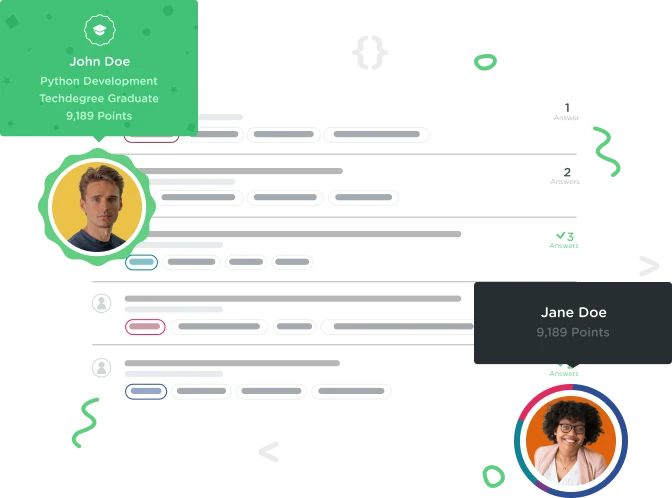

Emmanuel Oduja
7,198 PointsError I'm getting doesn't make sense
I think this should run, instead I'm getting an error every time
public class ConferenceRegistrationAssistant {
/**
* Assists in guiding people to the proper line based on their last name.
*
* @param lastName The person's last name
* @return The line number based on the first letter of lastName
*/
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
char firstLetter = lastName.charAt(0);
if(firstLetter >= 'A' || <= 'M')
{
return 1;
}else if(firstLetter >= 'N' || <= 'Z')
{
return 2;
}
/*
lineNumber should be set based on the first character of the person's last name
Line 1 - A thru M
Line 2 - N thru Z
*/
return lineNumber;
}
}
public class Example {
public static void main(String[] args) {
/*
IMPORTANT: You can compare characters using <, >. <=, >= and == just like numbers
*/
if ('C' < 'D') {
System.out.println("C comes before D");
}
if ('B' > 'A') {
System.out.println("B comes after A");
}
if ('E' >= 'E') {
System.out.println("E is equal to or comes after E");
}
// This code is here for demonstration purposes only...
ConferenceRegistrationAssistant assistant = new ConferenceRegistrationAssistant();
/*
Remember that there are 2 lines.
Line #1 is for A-M
Line #2 is for N-Z
*/
int lineNumber = 0;
/*
This should set lineNumber to 2 because
The last name is Zimmerman which starts with a Z.
Therefore it is between N-Z
*/
lineNumber = assistant.getLineNumberFor("Zimmerman");
/*
This method call should set lineNumber to 1, because 'A' from "Anderson" is between A-M.
*/
lineNumber = assistant.getLineNumberFor("Anderson");
/*
Likewise Charlie Brown's 'B' is between 'A' and 'M', so lineNumber should be set to 1
*/
lineNumber = assistant.getLineNumberFor("Brown");
}
}
1 Answer
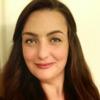
Jennifer Nordell
Treehouse TeacherHi there! You're doing pretty well here, but yes, you do have a syntax error. The reason is this: you can, of course, have many variables in your program. Right now, you're asking if the first letter is great than or equal to 'A' or ... and that's where it has the problem. You have to specify what should be less than or equal to 'M'. It has no way to guess which variable you want to compare.
So where you have:
if(firstLetter >= 'A' || <= 'M')
The correct syntax is:
if(firstLetter >= 'A' || firstLetter <= 'M')
Once you fix this, you will still encounter a problem. The letter shouldn't be greater than A or less than M. Remember, only one of those has to be true for it to execute the code inside the block. This means if the first letter is Z, then they will be assigned to line 1 as Z is greater than or equal to A. Instead, to go in line one the first letter should be greater than or equal to A AND less than or equal to M.
Hope this helps!