Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial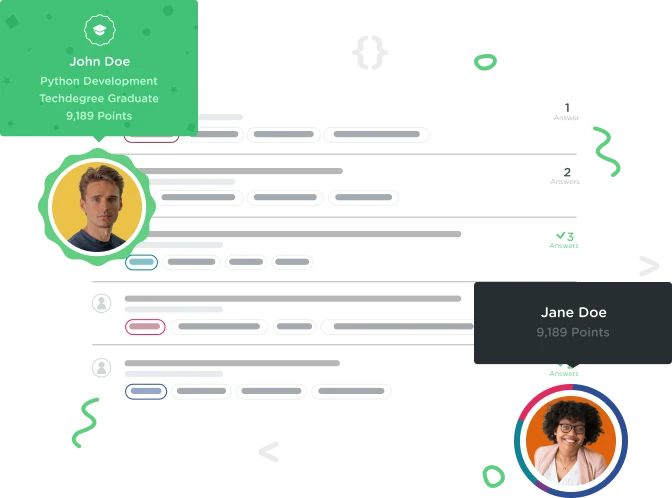
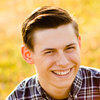
Caleb Kleveter
Treehouse Moderator 37,862 PointsError in code using Swift 2.0 setting current weather to the weatherDictionary in the CurrentWeather struct.
I don't think there is a difference between Pasan's and my code, but I am using Swift 2.0, and I think he is using Swift 1.2 here is my code:
import UIKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
if let plistPath = NSBundle.mainBundle().pathForResource("CurrentWeather", ofType: "plist"),
let weatherDictionary = NSDictionary(contentsOfFile: plistPath),
let currentWeatherDictionary = weatherDictionary["currently"] as? [String: AnyObject] {
/* v======== Error here */
let currentWeather = CurrentWeather(weatherDictionary: currentWeatherDictionary)
}
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
}
The issue says "Initialization of immutable value 'currentWeather' was never used; consider replacing with assignment to '_' or removing it"
4 Answers
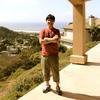
Richard Lu
20,185 PointsWhat you have here is a computed property that is never used
let currentWeatherDictionary = weatherDictionary["currently"] as? [String: AnyObject] {
let currentWeather = CurrentWeather(weatherDictionary: currentWeatherDictionary)
}
just add the if statement to fix it.
if let currentWeatherDictionary = weatherDictionary["currently"] as? [String: AnyObject] {
let currentWeather = CurrentWeather(weatherDictionary: currentWeatherDictionary)
}
This allows the weatherDictionary to ensure the type casting is correct.
I hope I've helped :)
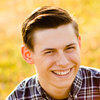
Caleb Kleveter
Treehouse Moderator 37,862 PointsThe issue is gone now, I don't know what happened.

Matthew Gomez
395 PointsI had the same issue, but with your corrections I wind up with two more issues.
let weatherDictionary = NSDictionary(contentsOfFile: plistPath), //error:Boolean condition requires 'where' to serparate it from variable binding
if let currentWeatherDictionary = weatherDictionary["currently"] as? [String: AnyObject] // Use of unresolved identifier 'Weather Dictionary'
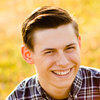
Caleb Kleveter
Treehouse Moderator 37,862 PointsI see what you are doing, but I have the if farther up, look at my code again.
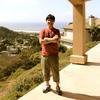
Richard Lu
20,185 PointsYour first if let is unwrapping the NSBundle string value since it may not exist (possibility that plistPath may be nil)
if let plistPath = NSBundle.mainBundle().pathForResource("CurrentWeather", ofType: "plist")
the second if let is used to type cast your dictionary to the specific types your dictionary holds.
if let currentWeatherDictionary = weatherDictionary["currently"] as? [String: AnyObject]
the 'as?' keyword essentially ask weatherDictionary["currently"] if it's a dictionary of type [String: AnyObject]. If weatherDictionary["current"] is another type, then currentWeatherDictionary would evaluate to nil.

Ben Myhre
28,726 PointsI think some review of this project needs to happen at treehouse, as this video was incorrect before I updated to El Capitan and it is incorrect after I update it as well. At least put some good notes in the video.
OK, so before I updated... I was two versions back and did not have Swift 2. Fair enough.... I need to update (even though there is no real mention of versioning issues in this track).
So, I updated. Now, while trying to follow along, the same code errors out... println is no longer a thing.
Not that people shouldn't be able to figure this out, but my hope for using the service is to make things less confusing to get to the meat of what needs to be learned and not add to the confusion. Rabbit hole of confusion is what is happening right up in here.
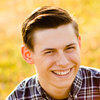
Caleb Kleveter
Treehouse Moderator 37,862 PointsYou might want to do Swift 2.0 basics since you updated to that version of Swift. Also, in Swift 2.0, println
was changed to print

Lawrence Yeo
3,236 PointsHello, I'm using the code that Caleb described above:
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
if let plistPath = NSBundle.mainBundle().pathForResource("CurrentWeather", ofType: "plist"),
let weatherDictionary = NSDictionary(contentsOfFile: plistPath),
let currentWeatherDictionary = weatherDictionary["currently"] as? [String: AnyObject] {
let currentWeather = CurrentWeather(weatherDictionary: currentWeatherDictionary)
}
I am getting the error that was mentioned earlier: "Initialization of immutable value 'currentWeatherDictionary' was never used; consider replacing with assignment to '_' or removing it". How can I resolve this?
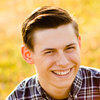
Caleb Kleveter
Treehouse Moderator 37,862 PointsThat happens when the constant is not used, so keep writing the code and you should be fine.
Ben Myhre
28,726 PointsBen Myhre
28,726 PointsI think I am going to quit this course for the time being. After I updated and got to to a certain point in the project... It stopped compiling because the view was created under a different os version. sigh. so annoying and I am sure there was a way to solve it, but aaaargh! :) Not really treehouses fault, just one thing after another with this track.