Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial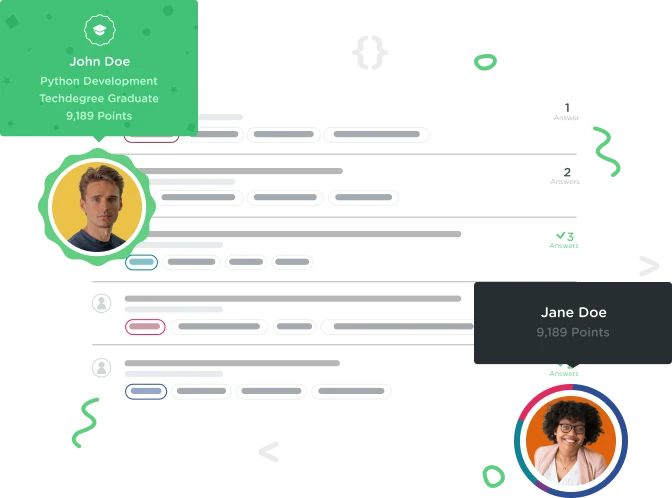

Vinayak Gadag
749 PointsError in ForumPost object in Example.java
Getting error for FOrumPost object in the Example.java
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getDescription(){
return mDescription;
}
public ForumPost(User author,String title, String description){
mAuthor=author;
mTitle=title;
mDescription=description;
}
// TODO: We need to expose the description
}
public class User {
private String mFirstName, mLastName;
public User(String firstName, String lastName) {
// TODO: Set the private fields here
mFirstName=firstName;
mLastName=lastName;
}
public String getFirstName(){
return mFirstName;
}
public String getLastName(){
return mLastName;
}
}
public class Forum {
private String mTopic;
public String getTopic() {
return mTopic;
}
public Forum(String topic){
mTopic = topic;
}
public void addPost(ForumPost post) {
// When all is ready uncomment this...
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
}
}
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
Forum forum = new Forum("Java");
//Take the first two elements passed args
User author = new User("Craig","Dennis");
// Add the author, title and description
ForumPost post = new ForumPost(User.getFirstName,"Java","oops language");
forum.addPost(post);
}
}
2 Answers
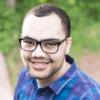
Philip Gales
15,193 PointsHere is your problem:
//Take the first two elements passed args
User author = new User("Craig","Dennis");
// Add the author, title and description
ForumPost post = new ForumPost(User.getFirstName,"Java","oops language");
The first comment tells you to take the first 2 elements passed args. This can be confusing since you haven't worked with the main method, but it is referring to the args parameter in the main method.
public static void main(String[] args) {
They want you to use the String array args to pass in the first and last name, not assign your own. Remember, you do 'arrayName[0]' to get the first value in an array.
The second part of your problem is ForumPost asks for 'User' (aka author) and you passed in a String.
//first parameter is a 'User'
public ForumPost(User author, String title, String description){
Good news for you is previously in your main method you declared a 'User' object.
User author = new User("Craig","Dennis");
I will let you piece this all together, as this is an extremely challenging question and I am surprised you got this far!
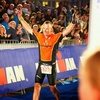
Steve Hunter
57,712 PointsHi there,
The ForumPost
constructor wants you to pass it a User
object, not a name. Try sending in the User
instance you have already created called author
instead of User.firstName
. You will then need to follow the instructions about the args[]
regarding the name of the User
. Get back to me if you need more help.
Steve.