Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial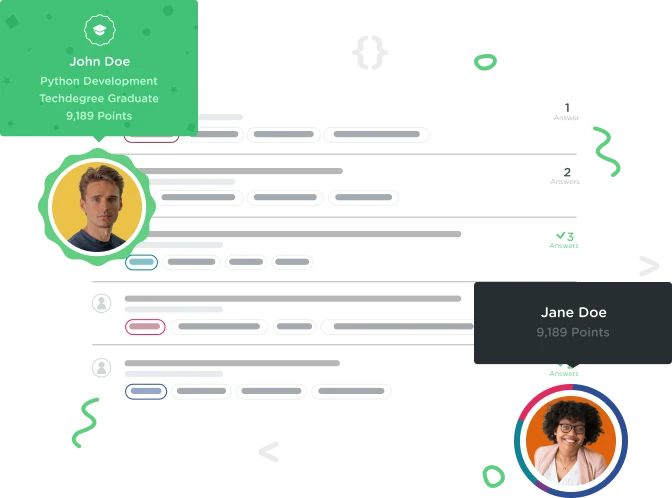
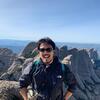
Carlos Chang Cheng
Courses Plus Student 12,030 PointsERROR IN LINE 4
Hi! I'm getting Uncaught Error: ERROR at getRandomNumber (random.js:4) at random.js:12
Here's the code (pretty much the same as the one in the video)
function getRandomNumber( lower, upper ) {
if (isNaN(upper) || isNaN(lower)) {
throw new Error("ERROR");
}
return Math.floor(Math.random() * (upper - lower + 1)) + lower;
}
console.log( getRandomNumber( 'nine', 24 ) ); console.log( getRandomNumber( 1, 100 ) ); console.log( getRandomNumber( 200, 'five hundred' ) ); console.log( getRandomNumber( 1000, 20000 ) ); console.log( getRandomNumber( 50, 100 ) );
3 Answers
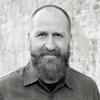
Dave Yankowiak
9,105 PointsI think you're actually doing it correctly, it's just that the browser is giving you more feedback than what is shown in the video (which is using an older browser version). When I run your code, the console also shows me which line of code is having the issue. Based on the sequence of your console.log statements, the browser SHOULD throw an uncaught error on that first call to the function since it uses 'nine'. When I move your console.log statements around so that it runs the function with a few valid numbers first, the console spits out the random numbers and then once it gets to one of the console.log statements with the invalid numbers, it throws the uncaught error.
In other words, looks good to me! :)

Tim Tapping
1,881 PointsHi Dave, I disagree. I move statements around to process a few 'good' ones and still get the uncaught error. WHY doesn't it work the first time and every time?
I'm using Chrome Version 57.0.2987.133 (64-bit) on a Mac.
function getRandomNumber( lower, upper ) { if(isNaN(lower) || isNaN(upper)) { throw new Error('err message'); } return Math.floor(Math.random() * (upper - lower + 1)) + lower; }
console.log( getRandomNumber( 1000, 20000 ) ); console.log( getRandomNumber( 1, 100 ) ); console.log( getRandomNumber( 'nine', 24 ) ); console.log( getRandomNumber( 200, 'five hundred' ) ); console.log( getRandomNumber( 50, 100 ) );

Minh Nguyen
4,574 PointsDave is correct, nothing wrong actually :-) the first call getRandomNumber('nine', 24)
falls into that nice condition, and throw new Error("ERROR")
is invoked, therefore we see that Uncaught Error: ERROR ...
in console. Like this (it actually halts at first console.log
already, the next console.log
won't be invoked as you can see)
function getRandomNumber( lower, upper ) {
if (isNaN(upper) || isNaN(lower)) {
throw new Error("ERROR");
}
return Math.floor(Math.random() * (upper - lower + 1)) + lower;
}
console.log(
getRandomNumber( 'nine', 24 ) // isNaN('nine') === true => throw ERROR in getRandomNumber()
);
console.log(
getRandomNumber( 1, 100 ) // lower (1) and upper (100) are not NaN, no error
);
console.log(
getRandomNumber( 200, 'five hundred' ) // isNaN('five hundred') === true => throw ERROR in getRandomNumber()
);
console.log(
getRandomNumber( 1000, 20000 ) // lower (1000) and upper (20000) are not NaN, no error
);
console.log(
getRandomNumber( 50, 100 ) // lower (50) and upper (100) are not NaN, no error
);
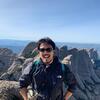
Carlos Chang Cheng
Courses Plus Student 12,030 PointsThank you guys!!! thanks for the feedback... we keep going!
Alec Sandoval
1,687 PointsAlec Sandoval
1,687 Pointswhy is an 'else' not needed in the function?