Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial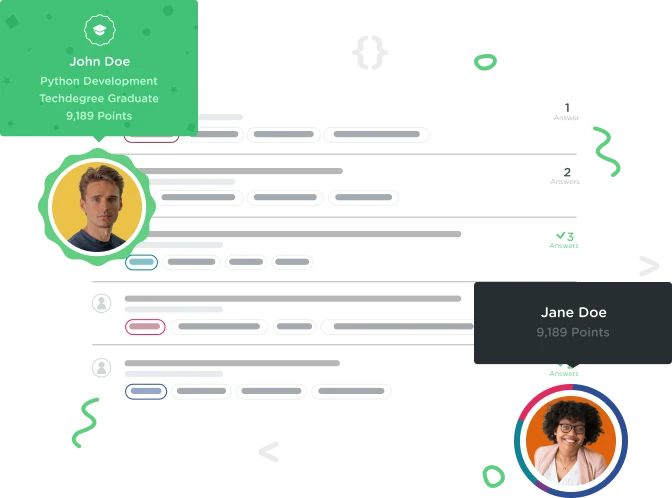
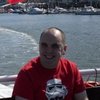
Sean Flanagan
33,236 PointsError in my loop file
Hi.
I got this error in my loop file:
/home/treehouse/workspace/2_loop.js:31
console.log("Item " + createRandomList[i] + " in the array is " + createRandomList[j]);
^
ReferenceError: i is not defined
Here's my code:
/* INSTRUCTIONS
To run this file, click in the Console below and type: node 2_loop.js
If the console isn't visible, click the View menu above and choose Show Console.
You can clear the console by typing `clear` and pressing enter.
If your program is stuck in an infinite loop, you can break out of the program by typing ctrl + C.
*/
/*Note: We've supplied you a basic function for generating a random number from 1 to 100 */
function random100() {
return Math.floor(Math.random() * 100) + 1;
}
/* 1. Create a function named createRandomList that uses a for loop to create an array containing 10 random numbers from 1 to 100 (use the supplied function above to generate the numbers). The function should return that array. */
function createRandomList()
{
for (let i = 1; i <= 100; i++) {
return Math.floor(Math.random() * 100) + 10;
}
}
/* 2. Call the createRandomList() function and store the results in a variable named myRandomList. */
var myRandomList = createRandomList();
/* 3. Use a for loop to access each element in the loop. Each time through the loop log a message to the console that looks something like this:
Item 0 in the array is 48
When you're done you should have output 10 lines to the console -- one for each element.
*/
for (let j = 0; j <= 10; j++) {
console.log("Item " + createRandomList[i] + " in the array is " + createRandomList[j]);
}
// Run your code by typing node 2_loop.js in the console below
3 Answers
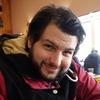
Eric M
11,546 PointsHi Sean,
This is a scope error. Look at the line the error message is pointing to. Is there a variable i
defined in this scope? What should that variable i
be?
It's also worth noting the instructions asked for the use of the random100()
function within your createRandomList()
function. Also, createRandomList()
should return a list of 10 items. Your function returns one.
Be aware that a return statement will early exit from a loop to achieve the return from the function. Your loop is set to run 100 times, but only runs once, but we actually want a list of 10 items.
You might find this code snippet helpful
let random_numbers = [];
for (var i = 0; i <= 10; i++)
{
random_numbers.push(random100());
}
Cheers,
Eric
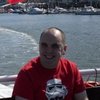
Sean Flanagan
33,236 PointsHi Eric.
I've made some changes to my code. Here it is.
/* INSTRUCTIONS
To run this file, click in the Console below and type: node 2_loop.js
If the console isn't visible, click the View menu above and choose Show Console.
You can clear the console by typing `clear` and pressing enter.
If your program is stuck in an infinite loop, you can break out of the program by typing ctrl + C.
*/
/*Note: We've supplied you a basic function for generating a random number from 1 to 100 */
function random100() {
return Math.floor(Math.random() * 100) + 1;
}
/* 1. Create a function named createRandomList that uses a for loop to create an array containing 10 random numbers from 1 to 100 (use the supplied function above to generate the numbers). The function should return that array. */
function createRandomList()
{
for (let i = 0; i <= 100; i++) {
return Math.floor(Math.random() * 100) + 10;
random100();
}
}
/* 2. Call the createRandomList() function and store the results in a variable named myRandomList. */
var myRandomList = createRandomList();
/* 3. Use a for loop to access each element in the loop. Each time through the loop log a message to the console that looks something like this:
Item 0 in the array is 48
When you're done you should have output 10 lines to the console -- one for each element.
*/
let random_numbers = [];
for (var i = 0; i < 10; i++) {
console.log("Item " + i + " in the array is " + createRandomList(random100()));
}
// Run your code by typing node 2_loop.js in the console below
The output is:
Item 0 in the array is 32
Item 1 in the array is 23
Item 2 in the array is 41
Item 3 in the array is 20
Item 4 in the array is 80
Item 5 in the array is 89
Item 6 in the array is 85
Item 7 in the array is 30
Item 8 in the array is 50
Item 9 in the array is 32
I think I'm making progress. Have I got it right?
Cheers
Sean
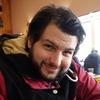
Eric M
11,546 PointsHi Sean,
While your output is good here, you don't actually have an array of numbers. Your code should do what the comments say it does, not something different that looks like it achieves a similar result.
I think you would benefit a lot from running through the JavaScript Loops, Arrays and Objects course, even if you've done it previously. You're misapplying some core concepts.
For instance when you should be looping through the array you instead call createRandomList
and pass it an argument of random100()
, which is another function - so your call looks like:
createRandomList(random100())
createRandomLIst
doesn't take a function as a parameter, in fact it has no parameters at all. In a stricter language this wouldn't even run/compile.
So let's take a look at the createRandomList
function itself. According to our instructions we need to create "a for loop to create an array containing 10 random numbers from 1 to 100 (use the supplied function above to generate the numbers). The function should return that array."
Okay, your function is:
function createRandomList()
{
for (let i = 0; i <= 100; i++)
{
return Math.floor(Math.random() * 100) + 10;
random100();
}
}
Your for loop is only going to run once. It will immediately return a single random number when the for loop executes. The return statement will cause the loop to never run again because we've already exited the function. random100()
is never called, but even if it was it wouldn't matter because nothing is done with the result. Also note that your loop is set to run 101 times if we don't exit early.
Below is this function updated to meet the requirements, I've added some comments - feel free to ask questions about anything you're curious about.
/* takes no arguments, returns an array of 10 random numbers */
function createRandomList()
{
// initialise an empty array
let array_of_random_numbers = [];
// loop 10 times, inserting a random number into the array
for (let i = 0; i < 10; i++)
{
// get a random number from the random100() function
let new_random_number = random100();
// put the random number in the array
array_of_random_numbers.push(new_random_number);
}
// return the array to where ever the function was called from
return array_of_random_numbers;
}
Okay, next bit:
/* 2. Call the createRandomList() function and store the results in a variable named myRandomList. */
var myRandomList = createRandomList();
This part was fine, but note that previously createRandomList() was returning a single value, not a list. With the new function this part of the program now works correctly.
Now we want to loop through myRandomList
to output the numbers.
for (let i = 0; i < 10; i++)
{
console.log("Item " + i + " in the array is " + myRandomList[i]);
}
Here we access an array index each loop, the array index incrementing with the loop (variable i
).
I think if you revist the core concepts of loops, functions, and arrays you will have an easier time overall.
Cheers and good luck,
Eric
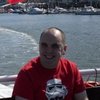
Sean Flanagan
33,236 PointsOk Eric. I've started going over the course you mention again. Thanks for your help.
Sean
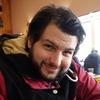
Eric M
11,546 PointsThat's great Sean, good luck and happy coding!
Sean Flanagan
33,236 PointsSean Flanagan
33,236 PointsHi Eric.
I appreciate your attempt to help me.
I've noted the function that we're initially supplied with. But the way the instructions are laid out confuses me. I tend to follow each set of instructions individually.
Eric M
11,546 PointsEric M
11,546 PointsHi Sean,
It's here:
/* 1. Create a function named createRandomList that uses a for loop to create an array containing 10 random numbers from 1 to 100 (use the supplied function above to generate the numbers). The function should return that array. */ function createRandomList()
Specifically the section in the brackets. The
random100()
function is directly above and has the note/*Note: We've supplied you a basic function for generating a random number from 1 to 100 */
The bigger issue is that you're returning one number between 10 and 110 instead of ten numbers between 0 and 100.
Were you able to resolve that issue?
If not feel free to post where you're code is at now and I'll help you fix it up.
Cheers,
Eric