Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial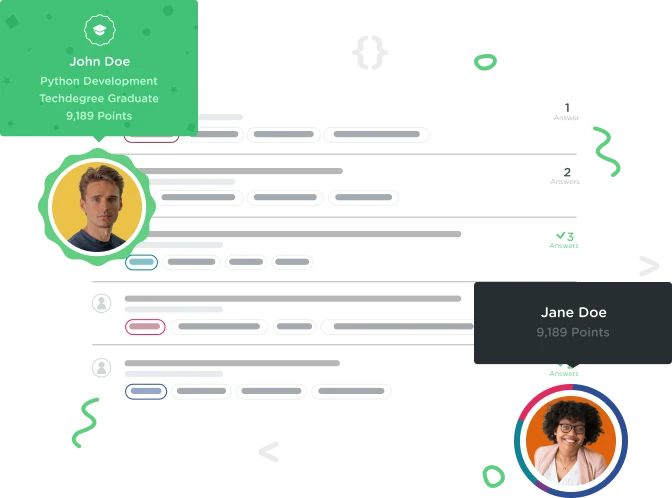
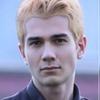
Dinu Comendant
6,049 Pointserror: incompatible types: Object cannot be converted to Song
Getting this error and can't understand where the problem lies, either I'm blind or the problem is in another place in the code:
./com/teamtreehouse/KaraokeMachine.java:122: error: incompatible types: Object cannot
be converted to Song
Song song = mSongQueue.poll();
This is my code for KaraokeMachine.java:
package com.teamtreehouse;
import com.teamtreehouse.model.Song;
import com.teamtreehouse.model.SongBook;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.ArrayDeque;
import java.util.ArrayList;
import java.util.List;
import java.util.HashMap;
import java.util.Map;
import java.util.Queue;
public class KaraokeMachine {
private SongBook mSongBook; //accessing mSongBook from SongBook file
private BufferedReader mReader;
private Map<String, String> mMenu;
private Queue mSongQueue;
public KaraokeMachine (SongBook songBook) {
mSongBook = songBook; //setting mSongBook as a parameter
mReader = new BufferedReader(new InputStreamReader(System.in)); //Inputstream reads from bytes into chars, since it asks for request, its better to wrap it up in BufferedReader
mSongQueue = new ArrayDeque<Song>();
mMenu = new HashMap<String,String> ();
mMenu.put("add", "Add a new song to the song book");
mMenu.put("choose", "Choose a song to sing!");
mMenu.put("play", "Play the next song");
mMenu.put("quit", "Quitting the program");
}
private String promptAction() throws IOException {
System.out.printf("There are %s songs available and %d in the queue. Your options are: %n", mSongBook.getSongCount(), mSongQueue.size());
for (Map.Entry<String, String> option : mMenu.entrySet()) {
System.out.printf("%s - %s %n",
option.getKey(),
option.getValue());
}
System.out.print("What do you want to do: ");
String choice = mReader.readLine();
return choice.trim().toLowerCase();
}
public void run () {
String choice = "";
do {
try {
choice = promptAction();
switch (choice) {
case "add":
Song song = promptNewSong();
mSongBook.addSong(song);
System.out.printf("%s added! %n%n", song);
break;
case "choose":
String artist = promptArtist();
Song artistSong = promptSongForArtist(artist);
mSongQueue.add(artistSong);
System.out.printf("You chose: %s %n", artistSong);
break;
case "play":
playNext();
break;
case "quit":
System.out.println("Thanks for using the program");
break;
default :
System.out.printf("Unknown choice: '%s'. Try again. %n%n%n",
choice);
}
} catch (IOException ioe) {
System.out.println("Problem with input");
ioe.printStackTrace();
}
} while (!choice.equals("quit"));
}
private Song promptNewSong() throws IOException {
System.out.print("Enter artist's name: ");
String artist = mReader.readLine();
System.out.print("Enter the title of the song: ");
String title = mReader.readLine();
System.out.print("Enter the videoURL: ");
String videoUrl = mReader.readLine();
return new Song(artist, title, videoUrl);
}
private String promptArtist() throws IOException {
System.out.println("Available artists: ");
List<String> artists = new ArrayList<>(mSongBook.getArtists());
int index = promptForIndex(artists);
return artists.get(index);
}
private Song promptSongForArtist (String artist) throws IOException {
List<Song> songs = mSongBook.getSongsForArtist(artist);
List<String> songTitles = new ArrayList<>();
for (Song song : songs) {
songTitles.add(song.getTitle());
}
System.out.printf("Available songs for %s: %n", artist);
int index = promptForIndex(songTitles);
return songs.get(index);
}
private int promptForIndex (List<String> options) throws IOException {
int counter = 1;
for (String option : options) {
System.out.printf("%d ) %s %n", counter, option);
counter ++;
}
System.out.println("Your choice: ");
String optionAsString = mReader.readLine();
int choice = Integer.parseInt(optionAsString.trim());
return choice -1;
}
public void playNext() {
Song song = mSongQueue.poll();
if (song == null) {
System.out.println("Sorry, there are no songs in the queue" +
"Use the button CHOOSE to add a song");
} else {
System.out.printf("%n%n%n Open %s to hear %s by %s %n%n%n",
song.getVideoUrl(),
song.getTitle(),
song.getArtist());
}
}
}