Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial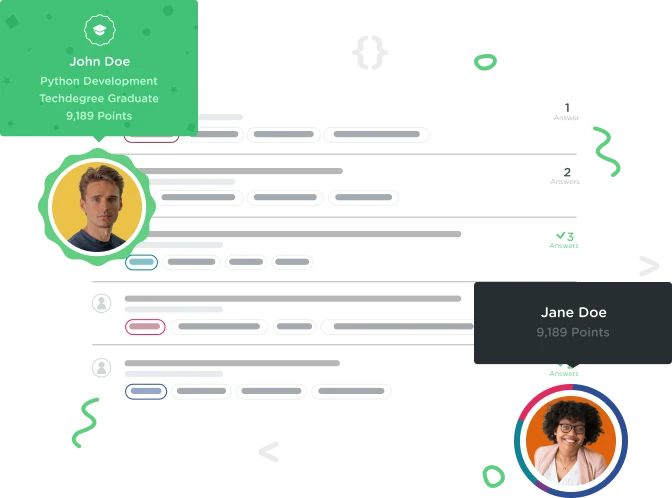

Derek Markman
16,291 PointsERROR: Length must be at least 1 (was 0)
int colorAsInt = Color.parseColor(color);
In the IDE "color" has a red line underneath it and it says: Length must be at least 1 (was 0)
For reference here's my code:
package com.teamtreehouse.funfacts;
import android.graphics.Color;
import java.util.Random;
/**
* Created by Derek on 8/22/2015.
*/
public class ColorWheel {
// Member variable (properties about the object)
public String[] mColors = {
"#39add1", // light blue
"#3079ab", // dark blue
"#c25975", // mauve
"#e15258", // red
"#f9845b", // orange
"#838cc7", // lavender
"#7d669e", // purple
"#53bbb4", // aqua
"#51b46d", // green
"#e0ab18", // mustard
"#637a91", // dark gray
"#f092b0", // pink
"#b7c0c7" // light gray
};
//Method (abilities: things the object can do)
public int getColor() {
String color = "";
// randomly select a fact
Random randomGenerator = new Random(); // construct a new Random number generator
int randomNumber = randomGenerator.nextInt(mColors.length);
color = mColors[randomNumber];
int colorAsInt = Color.parseColor(color);
return colorAsInt;
}
}
Even with this error, my code still works properly and is changing the background color.

malkio kusanagi
12,336 PointsHey man could it be that your color variable is empty at the time you are parsing it?

Derek Markman
16,291 PointsIndeed I have and the error still isn't going away.
Even with this error my code is working properly.

Derek Markman
16,291 PointsWell inside my getColor method
public int getColor() {
String color = "";
// randomly select a fact
Random randomGenerator = new Random(); // construct a new Random number generator
int randomNumber = randomGenerator.nextInt(mColors.length);
color = mColors[randomNumber];
int colorAsInt;
colorAsInt = Color.parseColor(color);
return colorAsInt;
}
Isn't my color variable set to that RNG so I wouldn't know if it's 0, because it could be any number from 0 - 12, right? Since there are 13 colors inside my mColors array.

malkio kusanagi
12,336 PointsYou could narrow down the problem by hardcoding
int colorAsInt = Color.parseColor("#b7c0c7");
// or
int colorAsInt = Color.parseColor( mColors[1] );
if this removes the red line on ide, then the error must be related to the way you are setting the value of String color; or the random generator must be throwing numbers outside the array length

Derek Markman
16,291 PointsWell I don't think I want to hardcode the color, because I still want it to dynamically switch my background colors around, but when I do hardcode the color it gets rid of my error. But either way my code is still working I just wish I knew why I'm getting this weird error.

malkio kusanagi
12,336 PointsHey man does your randomGenerator class work properly? I think it's throwing that error because color has an empty string/null value during the time you are parsing it?
is it a runtime or compile time error? are you able to run the app on emulator?

Derek Markman
16,291 PointsHey thanks for helping me out, I just fixed the error, you were right. I was getting an error because I had initialized my color variable to an empty String. So instead I just simply declared the color variable, and it removed my error and my code is still working properly.
1 Answer

Derek Markman
16,291 PointsIf anyone else is getting the same error on "color" where it says "Length must be at least 1 (was 0)" just change:
String color = "";
To:
String color;

Josiah Wetzel
7,997 PointsThis helped me too, thanks!
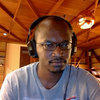
Daniel Muchiri
15,407 PointsThanks Derek Markman, I had the same problem. Declaring String color; instead of String color = ""; worked!!

Marc Wenzel
896 PointsThanks Derek your answer worked for me.
malkio kusanagi
12,336 Pointsmalkio kusanagi
12,336 PointsHave you tried cleaning up the project then rebuilding?