Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial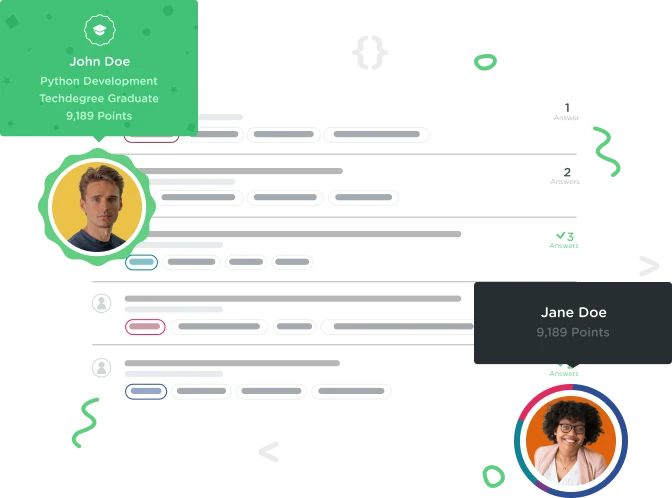
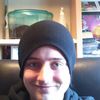
Max Reinsch
5,740 PointsError: Main method
public class PezDispenser {
public static final int MAX_PEZ = 12;
private String mCharacterName;
private int mPezCount;
public PezDispenser(String characterName) {
mCharacterName = characterName;
mPezCount = 0;
}
public boolean isEmpty() {
return mPezCount == 0;
}
public void load() {
mPezCount = MAX_PEZ;
}
public String getCharacterName() {
return mCharacterName;
}
}
When I compile and run it in the console I get the following:
"Error: Main method not found in class PezDispenser, please define the main method as:
public static void main(String[] args)
or a JavaFX application class must extend javafx.application.Application."
3 Answers
Kathryn Ann
10,071 PointsHi Max - When a Java program runs, it runs what's in the main method, so you need to have one in order for the program to work. Just like the error message says, right after you declare the class, begin the main method with the following. Put the rest of your code inside these curly braces.
public static void main (String args[]) {}
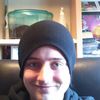
Max Reinsch
5,740 PointsThen I get the following errors:
PezDispenser.java:4: error: illegal start of expression
public static final int MAX_PEZ = 12;
^
PezDispenser.java:4: error: illegal start of expression
public static final int MAX_PEZ = 12;
^
PezDispenser.java:4: error: ';' expected
public static final int MAX_PEZ = 12;
^
PezDispenser.java:5: error: illegal start of expression
private String mCharacterName;
^
PezDispenser.java:6: error: illegal start of expression
private int mPezCount;
^
PezDispenser.java:8: error: illegal start of expression
public PezDispenser(String characterName) {
^
PezDispenser.java:8: error: ')' expected
public PezDispenser(String characterName) {
^
PezDispenser.java:8: error: illegal start of expression
public PezDispenser(String characterName) {
^
PezDispenser.java:8: error: ';' expected
public PezDispenser(String characterName) {
^
PezDispenser.java:25: error: class, interface, or enum expected
}
^
10 errors
Christopher Augg
21,223 PointsHello Max,
Kathryn is correct. I would also like to elaborate a little since you posted the errors after following this post. You have to instantiate your PezDispenser class object within the main method. This is what Kathryn is referring to by "Put the rest of your code in the curly braces." One way of doing this is like so:
public class PezDispenser {
public static final int MAX_PEZ = 12;
private String mCharacterName;
private int mPezCount;
public PezDispenser(String characterName) {
mCharacterName = characterName;
mPezCount = 0;
}
public boolean isEmpty() {
return mPezCount == 0;
}
public void load() {
mPezCount = MAX_PEZ;
}
public String getCharacterName() {
return mCharacterName;
}
public static void main(String[] args) {
PezDispenser pez = new PezDispenser("Smokey");
System.out.println("Is it empty? : " + pez.isEmpty());
System.out.println("Loading the dispenser");
pez.load();
System.out.println("Is it empty? : " + pez.isEmpty());
System.out.println("Character Name : " + pez.getCharacterName());
}
}
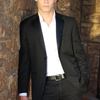
Kalen Loncar
8,743 PointsCorrect me if I'm wrong, but I thought he put that code in the Example.java file and because of that he didn't have to include it in PezDispenser.java, because they're in the same folder. I'm still getting the Error: Main method not found in class message and I don't understand why. Why do I need to add the code that Christopher Augg added to get my code to pass when Craig didn't in the video?
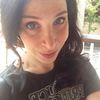
Marni Kostman
7,558 PointsWhat code are you using in your console to execute the program? I had the same issue and I realized the problem was that I was using: clear && javac PezDispenser.java && java PezDispenser Once I ran clear && javac Example.java && java Example it worked
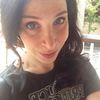
Marni Kostman
7,558 PointsWhat code are you using in your console to execute the program? I had the same issue and I realized the problem was that I was using: clear && javac PezDispenser.java && java PezDispenser Once I ran clear && javac Example.java && java Example it worked
Christopher Augg
21,223 PointsChristopher Augg
21,223 PointsKalen,
Sorry for the confusion. You are absolutely correct. You do not have to include the main method in the PezDispenser class file. I was only providing an example of one way that an object can be instantiated and used within the main method to elaborate what Kathyn meant by "Put the rest of your code inside these curly braces."
Please open your workspace and create a snapshot by clicking on the square icon on the top right. It will give you a link that you can post here. This will allow me to look over your code and help you solve your issue.
Edit: Here is an example of the code that I wrote previously in which I changed by placing the main method in Example.java as you mentioned :
https://w.trhou.se/j4bagtvz4j
Regards,
Chris