Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial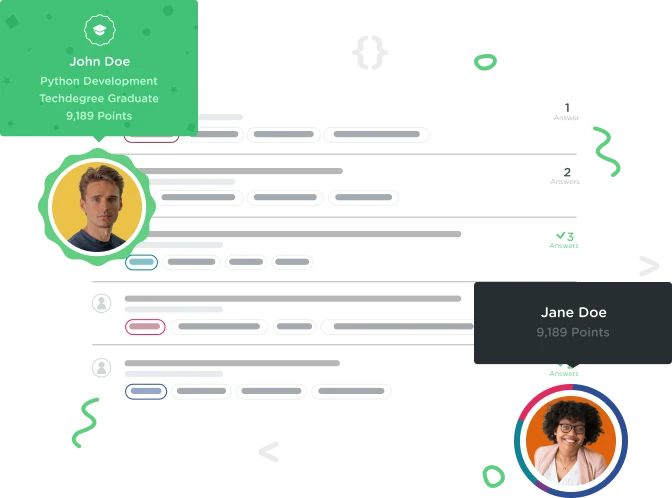

Barry Johnson
1,728 PointsError Message
Hi All -
I keep receiving the drawable cannot be resolved message post my setContentView line. Can someone look at the code and see where I missed something?
package com.example.alltruths;
import android.app.Activity; import android.graphics.drawable.AnimationDrawable; import android.media.MediaPlayer; import android.os.Bundle; import android.view.Menu; import android.view.View; import android.view.animation.AlphaAnimation; import android.widget.Button; import android.widget.ImageView; import android.widget.TextView;
public class MainActivity extends Activity {
private static final String R = null;
private AllTruths mAllTruths = new AllTruths();
private TextView mAnswerLabel;
private Button mGetAnswerButton;
private ImageView mAllTruthsImage;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//Assigning Views from layout
mAnswerLabel = (TextView) findViewById(R.id.textView1);
mGetAnswerButton = (Button) findViewById(R.id. button1);
mAllTruthsImage = (ImageView) findViewById(R.id.imageView1);
mGetAnswerButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View arg0) {
String answer =mAllTruths.getAnswer();
//update the label with the dynamic answer
mAnswerLabel.setText(answer);
animateAllTruths();
animateAnswer();
playSound();
}
});
}
private void animateAllTruths(){
mAllTruthsImage.setImageResource(R.Drawable.ball_animation);
AnimationDrawable ballAnimation = (AnimationDrawable) mAllTruthsImage.getDrawable();
if (ballAnimation.isRunning()) {
ballAnimation.stop();
}
ballAnimation.start();
}
private void animateAnswer(){
AlphaAnimation fadeInAnimation = new AlphaAnimation(0, 1);
fadeInAnimation.setDuration(1500);
fadeInAnimation.setFillAfter(true);
mAnswerLabel.setAnimation(fadeInAnimation);
}
private void playSound(){
MediaPlayer player = MediaPlayer.create(this, R.raw.crystal_ball);
player.start();
player.setOnCompletionListener(new onCompletionListener() {
public void onCompletion(MediaPlayer mp) {
mp.release();
}
}
});
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}
8 Answers
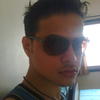
Sanat Tripathi
8,109 PointsYou might have some problem with your XML layout files. This kind of error usually comes when your (Resource) file i.e. R.java is not properly generated due to some errors in your layout file. Try to debug your XML files and if the error still persists then post your layout code too.

Barry Johnson
1,728 PointsHi Sanatkumar -
I tried to debug and I'm getting the same message. There doesn't appear to be a problem with my xml editor.
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center_horizontal" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context=".MainActivity" >
<ImageView
android:id="@+id/imageView1"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:scaleType="fitCenter"
android:src="@drawable/ball" />
<LinearLayout
android:id="@+id/linearLayout1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/imageView1"
android:layout_centerVertical="true"
android:gravity="center_horizontal"
android:orientation="horizontal"
android:weightSum="1" >
<View
android:id="@+id/view1"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_weight="0.2" />
<TextView
android:id="@+id/textView1"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="0.6"
android:gravity="center_horizontal"
android:shadowColor="@android:color/white"
android:shadowRadius="10"
android:textColor="@android:color/white"
android:textSize="32dp" />
<View
android:id="@+id/View01"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_weight="0.2" />
</LinearLayout>
</RelativeLayout>
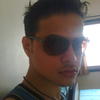
Sanat Tripathi
8,109 PointsI am unsure man, try cleaning your project in eclipse maybe then you might see some errors. Now its time for our teachers to save us :)

Barry Johnson
1,728 PointsHi Sanatkumar - I was able to figure out the problem. One last thing that I seem to be stuck on is there's an error on the last line. I think I possibly missed a { somewhere. Do you see where I may have missed it?
private void animateAnswer(){ AlphaAnimation fadeInAnimation = new AlphaAnimation(0, 1); fadeInAnimation.setDuration(1500); fadeInAnimation.setFillAfter(true);
mAnswerLabel.setAnimation(fadeInAnimation);
}
private void playSound(){
MediaPlayer player = MediaPlayer.create(this, R.raw.crystal_ball);
player.start();
player.setOnCompletionListener(new OnCompletionListener() {
public void onCompletion(MediaPlayer mp) {
mp.release();
}
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
private void handleNeanswer() {
String answer =mAllTruths.getAnswer();
//update the label with the dynamic answer
mAnswerLabel.setText(answer);
animateAllTruths();
animateAnswer();
playSound();
}
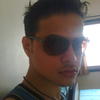
Sanat Tripathi
8,109 PointsYes I think in the playsound function you mess up the curly braces when you define an anonymous class.
private void playSound(){ MediaPlayer player = MediaPlayer.create(this, R.raw.crystal_ball); player.start(); player.setOnCompletionListener(new OnCompletionListener() { public void onCompletion(MediaPlayer mp) { mp.release(); } }); //based on your posted code, u were missing these, i guess

Barry Johnson
1,728 PointsThanks, I look and found that. Strangely, now, I'm getting a yellow hazard, not a red error on some of my fields that says "The method handleNeanswer() from the type MainActivity is never used locally" This came from that particular section, but also says it for a couple more of my "m" classes. private void playSound(){ MediaPlayer player = MediaPlayer.create(this, R.raw.crystal_ball); player.start(); player.setOnCompletionListener(new OnCompletionListener() { public void onCompletion(MediaPlayer mp) { mp.release(); } }); }
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
private void handleNeanswer() {
String answer =mAllTruths.getAnswer();
//update the label with the dynamic answer
mAnswerLabel.setText(answer);
animateAllTruths();
animateAnswer();
playSound();
}};
On my activity main xml page, I have the following error under image view, "The markup in the document following the root element must be well-formed."
<ImageView android:id="@+id/imageView1" android:layout_width="match_parent" android:layout_height="match_parent" android:scaleType="fitCenter" android:src="@drawable/ball" />
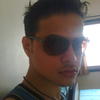
Sanat Tripathi
8,109 PointsWatch for opening and closing tags in the xml file. Those yellow markers indicate warnings and usually those don't matter, you can still run the application with them hanging out there. And try to follow the video tutorials as closely as possible; whenever teachers write code on the screen, you should pause the video and write them in your editor too.

Barry Johnson
1,728 PointsYep, I always do. Problem with this one was, I was writing Python and SQL at the same time and my computer crashed. Once I restored everything, I had errors.
I can't run the app yep because there's an error on the xml file tab as I referenced earlier. I'm receiving an error on image view.
<ImageView
android:id="@+id/imageView1"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:scaleType="fitCenter"
android:src="@drawable/ball" />