Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial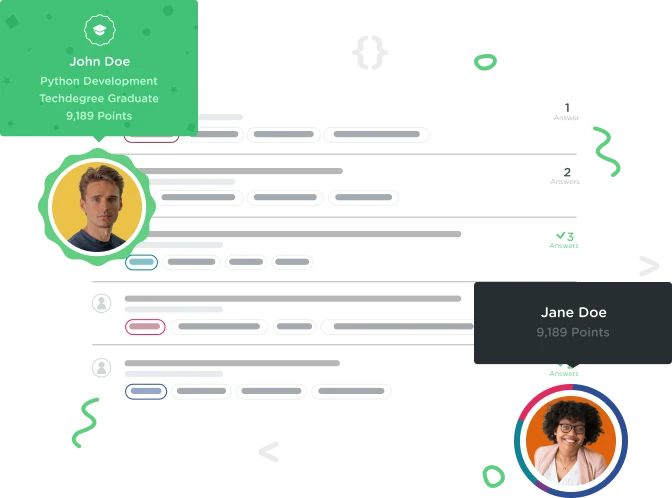
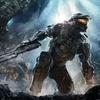
Faraz Malik
20,721 PointsError message: "Expression pattern of type 'Point' cannot match values of type 'Point'"
I am using Xcode. Hello there. I am trying to construct an app where pressing buttons allows you to move a dot across 4 UILabels, which have been labelled as coordinates. Please look at my code below of ViewController.swift (Cocoa touch file) and MovementConstruction.swift.
ViewController.swift:
import UIKit
class ViewController: UIViewController {
@IBOutlet weak var upButton: UIButton!
@IBOutlet weak var rightButton: UIButton!
@IBOutlet weak var downButton: UIButton!
@IBOutlet weak var leftButton: UIButton!
@IBOutlet weak var coordinates0_0: UILabel!
@IBOutlet weak var coordinates0_1: UILabel!
@IBOutlet weak var coordinates1_0: UILabel!
@IBOutlet weak var coordinates1_1: UILabel!
let backgroundColorProvider = BackgroundColorProvider()
var location = Location()
var currentPosition = Point()
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
@IBAction func upMovement() {
view.backgroundColor = backgroundColorProvider.randomColor()
currentPosition = location.moveUp()
}
@IBAction func downMovement() {
view.backgroundColor = backgroundColorProvider.randomColor()
currentPosition = location.moveDown()
}
@IBAction func leftMovement() {
view.backgroundColor = backgroundColorProvider.randomColor()
currentPosition = location.moveLeft()
}
@IBAction func rightMovement() {
view.backgroundColor = backgroundColorProvider.randomColor()
currentPosition = location.moveRight()
}
}
MovementConstruction.swift:
import Foundation
import UIKit
struct Point {
var x: Int = 0
var y: Int = 0
}
class Location {
var currentLocation = Point()
var possibleLocation0_0 = Point(x: 0, y: 0)
var possibleLocation0_1 = Point(x: 0, y: 1)
var possibleLocation1_0 = Point(x: 1, y: 0)
var possibleLocation1_1 = Point(x: 1, y: 1)
func moveUp() -> Point {
currentLocation.y += 1
return currentLocation
}
func moveDown() -> Point {
currentLocation.y -= 1
return currentLocation
}
func moveLeft() -> Point {
currentLocation.x -= 1
return currentLocation
}
func moveRight() -> Point {
currentLocation.x += 1
return currentLocation
}
func returnCorrectVariable(ofCoordinate coordinate: Point, area0_0: UILabel, area0_1: UILabel, area1_0: UILabel, area1_1: UILabel) -> UILabel {
switch coordinate {
case possibleLocation0_0: return area0_0
case possibleLocation0_1: return area0_1
case possibleLocation1_0: return area1_0
case possibleLocation1_1: return area1_1
default:
return area0_0
currentLocation = Point()
}
}
}
NOTE: The error is present in the switch statement right above, with the error "Expression pattern of type 'Point' cannot match values of type 'Point'" being given at each case. The code is still being worked on.
1 Answer
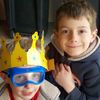
Chris Stromberg
Courses Plus Student 13,389 Pointsfunc returnCorrectVariable(ofCoordinate coordinate: Point, area0_0: UILabel, area0_1: UILabel, area1_0: UILabel, area1_1: UILabel) -> UILabel {
let coordinateValue = (coordinate.x, coordinate.y)
switch coordinateValue {
case (possibleLocation0_0.x, possibleLocation0_0.y) : return area0_0
case (possibleLocation0_1.x, possibleLocation0_1.y) : return area0_0
case (possibleLocation1_0.x, possibleLocation1_0.y) : return area0_0
case (possibleLocation1_1.x, possibleLocation1_1.y) : return area0_0
default:
currentLocation = Point()
return area0_0
}
}
Faraz Malik
20,721 PointsFaraz Malik
20,721 PointsThanks a bunch! I should have realised comparison operators donβt work on custom types.