Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial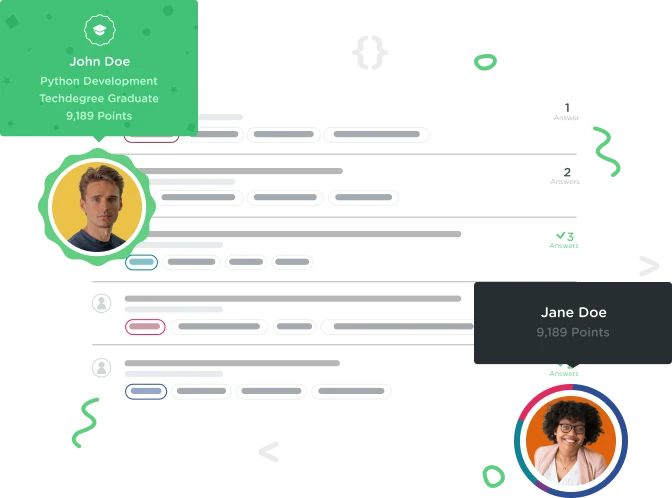
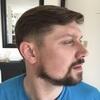
Justin Molyneaux
13,329 PointsERROR - My Arrows Bounce Off of the Targets
For some reason, after I implemented the ITargetable Interface when I shoot my arrows they just bounce off of the targets.
The arrows are supposed to hit the targets and then stay stuck in them.
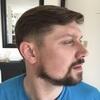
Justin Molyneaux
13,329 PointsHahaha, no IReboundable utilized yet.
Here is my code that I attached as a Component to the Arrow prefab.
using UnityEngine;
using System.Collections;
public class ArrowCollision : MonoBehaviour
{
private void onCollisionEnter(Collision other)
{
if (other.gameObject.GetComponent<ITargetable> () != null)
{
// checks to see if OnCollisionEnter() is being called when arrow hits target
Debug.Log("Arrow hit the target");
// Call the TargetReaction() on the component that implements the ITargetable interface
other.gameObject.GetComponent<ITargetable>().TargetReaction ();
// Stop the arrow from moving.
GetComponent<Rigidbody>().isKinematic = true;
// Parent the arrow to the target so that it's "stuck" to the target.
gameObject.transform.parent = other.transform;
}
}
}
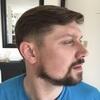
Justin Molyneaux
13,329 PointsHere is my ITargetable Interface
using UnityEngine;
using System.Collections;
public interface ITargetable
{
void TargetReaction ();
}
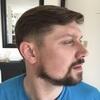
Justin Molyneaux
13,329 PointsHere is where I implemented the interface in the Target.cs
using UnityEngine;
using System.Collections;
public class Target : MonoBehaviour, ITargetable {
[SerializeField]
protected GameObject impactParticles;
protected AudioSource impactSounds;
protected virtual void Start() {
impactSounds = GetComponent<AudioSource>();
}
public virtual void DestroyTarget()
{
// Enable the rigidbody on the target to allow it to fall.
GetComponent<Rigidbody>().isKinematic = false;
// Turn off collisions to prevent double hits.
GetComponent<Collider>().enabled = false;
// Start to destroy this object.
Destroy(gameObject, 1f);
}
public virtual void TargetReaction()
{
// tests to see if this method is being called
Debug.Log("TargetReaction is being called.");
// Play an impact sound.
impactSounds.Play();
// Spawn hit particles.
Instantiate(impactParticles, transform.position, Quaternion.identity);
// Decrement the total number of targets.
TargetSpawner.totalTargets--;
// Add the target to the score.
GameManager.score++;
// Destroy the target.
DestroyTarget ();
}
}
1 Answer
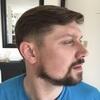
Justin Molyneaux
13,329 PointsI figured it out. It's because I wrote "onCollisionEnter" instead of the correct "OnCollisionEnter" method. Now my game works as expected.
Steven Parker
241,807 PointsSteven Parker
241,807 PointsDid you inherit from IReboundable?
Just kidding.
But it might help to share your code!