Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial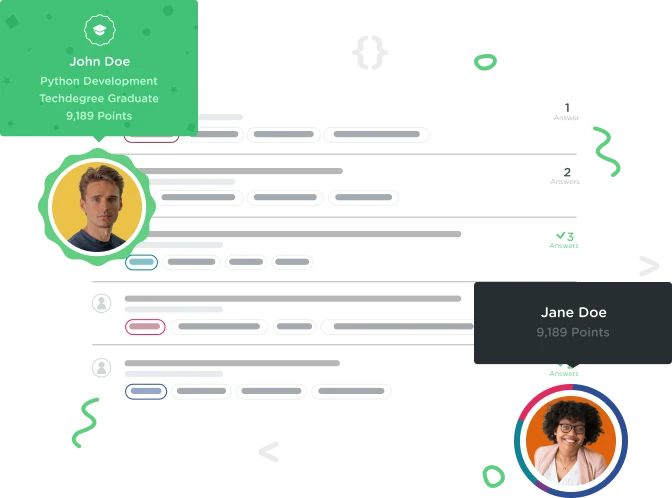

Melissa Tan
1,611 PointsError - non-static method print cannot be referenced
Hi, I have been having a string of errors of this nature and I can't seem to move beyond this:
public static void main(String[] args) { Console console = System.console(); // Welcome to the Introductions program! Your code goes below here Console.printf("Hello, my name is Melissa"); Console.printf("Melissa is learning Java"); } }
CONSOLE
treehouse:~/workspace$ javac Introductions.java
Picked up JAVA_TOOL_OPTIONS: -Xmx128m
Picked up _JAVA_OPTIONS: -Xmx128m
Introductions.java:8: error: non-static method printf(String,Object...) cannot be referenced from a static context
Console.printf("Hello, my name is Melissa");
^
Introductions.java:9: error: non-static method printf(String,Object...) cannot be referenced from a static context
Console.printf("Melissa is learning Java");
May I know how do I solve the problem in this code?
Thank you.
Mike Papamichail
4,883 PointsMike Papamichail
4,883 PointsHey there, i believe the problem lies on the method that you're calling on console.
First of all, i think you should write console with a lower-case c since that's the way you have declared it :
Console console = System.console();
Second of all, the printf() method prints to the console a formatted string-that explains the f at the end of the method- So it's structure looks like this :
console.printf(String , Object);
Explanation: String: A string goes here and you must use the %s symbol to print out another String(eg. this is used for printing out a String that is returned from a method)
Object: Here you place the String*that will be represented by the %s symbol inside your String.
*You can also have numbers instead of String passed in as objects but that's not the point here ;) -just throwing some extra info there-
Example: Let's assume we have a method named returnString() which returns the String "hey" :
We can use the String returned from this method when we call it-during runtime- and print it out to the console like this:
console.printf("%s Melissa" , returnString());
This will return to the console the following String:
hey Melissa
To sum up, if you just want to print out a string that's predefined, use the console.println() method.Otherwise,if you want to use a String that will be returned from a method but you don't know what it will be in the first place(let's say it's a user-inputed String) then you can use:
console.println("hey melissa");
Hope this helps! If you need help with this again, let me know and i will try my best to help you out :D !