Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial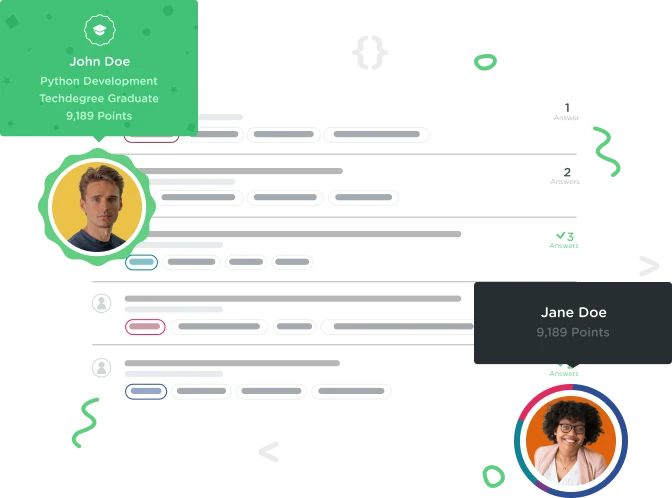

Solomon Asiedu-Ofei Jnr
1,015 PointsError on
I keep getting this error
./com/example/BlogPost.java:20: error: method does not override or implement a method from a supertype @Override ^
What super type are they referring to?
package com.example;
import java.util.Date;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
@Override
public int compareTo(Object obj) {
BlogPost post = (BlogPost) obj;
if(equals(post)) {
return 0;
}
return 1;
}
public String[] getWords() {
return mBody.split("\\s+");
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
3 Answers
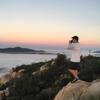
Chase Carnaroli
5,139 PointsActually try this. I think @Trent had the right idea with implementing comparable, but it wasn't equals(post)
that is the issue, comparable just wasn't implemented correctly.
Try this:
public class BlogPost implements Comparable {
// Other code
. . .
@Override public int compareTo(Object obj) {
BlogPost post = (BlogPost) obj;
if(equals(post)) {
return 0;
}
return 1;
}
}
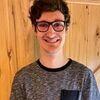
Trent Christofferson
18,846 Pointsyour class does not extend or implement anything, so it can not override anything. You need to implement Comparable.

Solomon Asiedu-Ofei Jnr
1,015 PointsHow do I implement a comparable
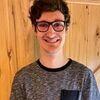
Trent Christofferson
18,846 Pointspublic class BlogPost implements Comparable { // random code in here }

Solomon Asiedu-Ofei Jnr
1,015 Pointshey Trent I did this but its not working.
@Override public class BlogPost { public int compareTo(Object obj) { BlogPost post = (BlogPost) obj; if(equals(post)) { return 0; } return 1; } }
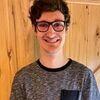
Trent Christofferson
18,846 PointsI think you are probably getting the error in your if statement instead of if(equals(post)) do if (obj.equals(post))
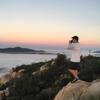
Chase Carnaroli
5,139 PointsI don't think you need @Override
at all in your code.... Try deleting that and see if it works.
Edit: I added what I think the code should look like in order to compile
public int compareTo(Object obj) {
BlogPost post = (BlogPost) obj;
if(equals(post)) {
return 0;
}
return 1;
}
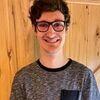
Trent Christofferson
18,846 PointsI think it was because he did equals(post) instead of obj.equals(post)
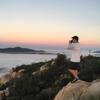
Chase Carnaroli
5,139 PointsNo, equals(post)
should be fine.
He doesn't actually need to put an object before that method because it is inside of a class.
Since it is inside of a class, writing equals(post)
is the same as writing this.equals(post)
both of which are valid code.
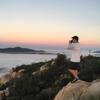
Chase Carnaroli
5,139 PointsActually obj.equals(post)
would not work at all.
obj
and post
are the same thing. The only difference is that post
is cast as a BlogPost