Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial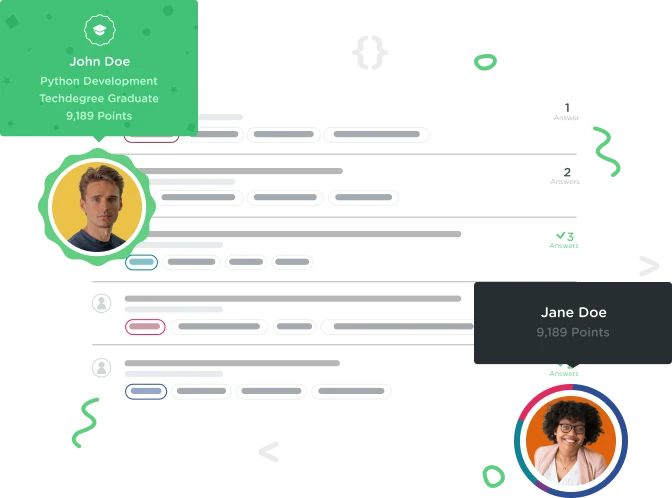

Fabiola Barrientos
9,467 PointsError, operator += not valid
Whenever i try to use the operator "+=", it tells me it isnt valid to use in int or double units. Last time i tried to get the average of something I used "+=", and i have no idea how to do it otherwise.
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
int sum = 0;
foreach( Frog TongueLength in frogs )
{
sum += TongueLength;
}
return sum / frogs.Length;
}
}
}
namespace Treehouse.CodeChallenges
{
public class Frog
{
public int TongueLength { get; }
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
}
}
1 Answer
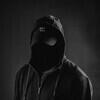
Tim Danner
3,284 PointsThe problem here is that you're trying to assign frog
objects to an int
variable, which causes type error.
Keep in mind that when you're looping through an array of a certain type, all the elements will also be of that type. To access the properties or methods, use the dot notation:
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
int sum = 0;
foreach (Frog frog in frogs)
{
// access the TongueLength property through dot notation
sum += frog.TongueLength;
}
return sum / frogs.Length;
}
}
}