Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial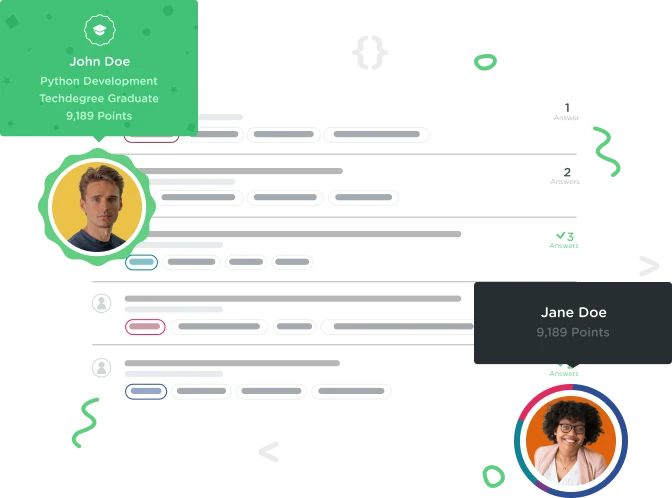

johnny reaser
1,240 Pointserror running code from quiz. Unexpected end of input for the last line
I'm doing the quiz.js and I get an uncaught SyntaxError: Unexpected end of Input on the last line everytime I run it. Below is the code.
/*
1. Store correct answers
- When quiz begins, no answers are correct
*/
let correct = 0;
// 2. Store the rank of a player
let rank;
// 3. Select the <main> HTML element
const main = document.querySelector('main');
/*
4. Ask at least 5 questions
- Store each answer in a variable
- Keep track of the number of correct answers
*/
const answer1 = prompt("question 1");
if ( answer1.toUpperCase() === 'RUBY' ) {
correct += 1;
}
const answer2 = prompt("question 2");
if ( answer2.toUpperCase() === 'PYTHON' ) {
correct += 1;
}
const answer3 = prompt("question 3");
if ( answer3.toUpperCase() === 'CSS' ) {
correct += 1;
}
const answer4 = prompt("question 4");
if ( answer4.toUpperCase() === 'HTML' ) {
correct += 1;
}
const answer5 = prompt("question 5");
if ( answer5.toUpperCase() === 'JAVASCRIPT' ) {
correct += 1;
/*
5. Rank player based on number of correct answers
- 5 correct = Gold
- 3-4 correct = Silver
- 1-2 correct = Bronze
- 0 correct = No crown
*/
if (correct === 5) {
rank = "Gold";
} else if (correct >= 3) {
rank = "Silver";
} else if (correct >= 2) {
rank = "Bronze";
} else {
rank = "none";
}
// 6. Output results to the <main> element
document.querySelector('html').innerHTML = `
<h2>You got ${correct} out of 5 questions correct.</h2>
<p>Crown earned: ${rank}</p>
`;
Mod edit: added markdown for code readability. Check out the "markdown cheatsheet" linked below the comment box for syntax examples :)
3 Answers

johnny reaser
1,240 PointsFound my problem using Visual Studio. I was missing a closing bracket on answer 5. Added the bracket and got it to run. thanks
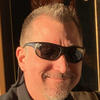
Peter Vann
36,427 PointsAlso, this line is suspect:
document.querySelector('html').innerHTML = <h2>You got ${correct} out of 5 questions correct.</h2> <p>Crown earned: ${rank}</p> ;
You appear to be attempting to use a template literal to make your HTML string and are missing the backticks.
I would do something more like this:
const HTML = `<h2>You got ${correct} out of 5 questions correct.</h2><p>Crown earned: ${rank}</p>`;
document.querySelector('html').innerHTML = HTML;
I don't know if that's all the issues, but it should get you further along.
Again, hope that helps.
Stay safe and happy coding!
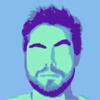
Cameron Childres
11,820 PointsJust a heads up, backticks can disappear in forum posts when the code isn't wrapped in lines of triple backticks -- anything typed like `this` that's not in a code block will format as a span of code like this
. I've edited the post now to display as a code block. Good eye though, thanks for contributing!
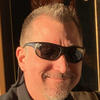
Peter Vann
36,427 PointsHi Johnny!
I see one issue right off the bat.
In this line:
const main = document.querySelector('main');
You are missing the id/class identifier.
Keep in mind getElementById and getElementsByClassName don't reguire them (for obvious reasons), but querySelector and querySelectorAll, do.
if the main element has the class of 'main', your code needs to be:
const main = document.querySelector('.main');
if the main element has the id of 'main', your code needs to be:
const main = document.querySelector('#main');
I hope that helps.
Stay safe and happy coding!
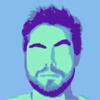
Cameron Childres
11,820 PointsQuick note, you can select elements with the querySelector() method as Johnny's done here -- any valid CSS selector string will work. document.querySelector('main')
will select the first <main> element on the page.