Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial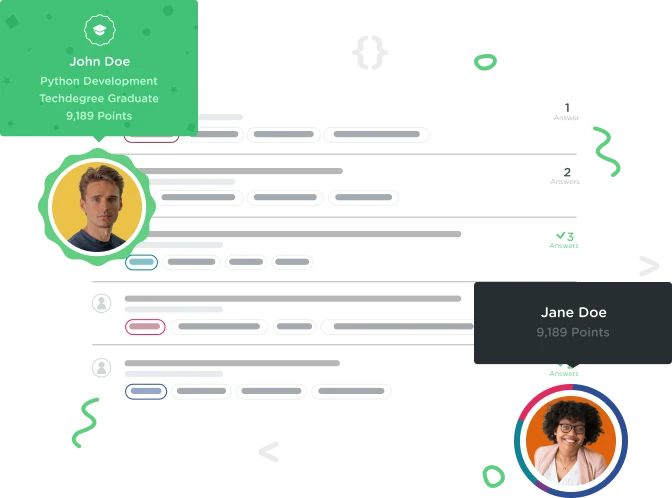
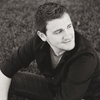
Zachary Zell
4,747 PointsError that closes app
04-09 19:19:18.370 30769-30769/com.example.zachary.ribbitnew E/AndroidRuntime﹕ FATAL EXCEPTION: main Process: com.example.zachary.ribbitnew, PID: 30769 java.lang.RuntimeException: Content has view with id attribute 'android.R.id.list' that is not a ListView class at android.app.ListFragment.ensureList(ListFragment.java:402) at android.app.ListFragment.onViewCreated(ListFragment.java:203) at android.app.FragmentManagerImpl.moveToState(FragmentManager.java:908) at android.app.FragmentManagerImpl.moveToState(FragmentManager.java:1067) at android.app.BackStackRecord.run(BackStackRecord.java:834) at android.app.FragmentManagerImpl.execPendingActions(FragmentManager.java:1452) at android.app.FragmentManagerImpl.executePendingTransactions(FragmentManager.java:483) at android.support.v13.app.FragmentPagerAdapter.finishUpdate(FragmentPagerAdapter.java:145) at android.support.v4.view.ViewPager.populate(ViewPager.java:1073) at android.support.v4.view.ViewPager.populate(ViewPager.java:919) at android.support.v4.view.ViewPager.onMeasure(ViewPager.java:1441) at android.view.View.measure(View.java:17547) at android.view.ViewGroup.measureChildWithMargins(ViewGroup.java:5535) at android.widget.FrameLayout.onMeasure(FrameLayout.java:436) at android.view.View.measure(View.java:17547) at android.view.ViewGroup.measureChildWithMargins(ViewGroup.java:5535) at com.android.internal.widget.ActionBarOverlayLayout.onMeasure(ActionBarOverlayLayout.java:447) at android.view.View.measure(View.java:17547) at android.view.ViewGroup.measureChildWithMargins(ViewGroup.java:5535) at android.widget.FrameLayout.onMeasure(FrameLayout.java:436) at com.android.internal.policy.impl.PhoneWindow$DecorView.onMeasure(PhoneWindow.java:2615) at android.view.View.measure(View.java:17547) at android.view.ViewRootImpl.performMeasure(ViewRootImpl.java:2015) at android.view.ViewRootImpl.measureHierarchy(ViewRootImpl.java:1173) at android.view.ViewRootImpl.performTraversals(ViewRootImpl.java:1379) at android.view.ViewRootImpl.doTraversal(ViewRootImpl.java:1061) at android.view.ViewRootImpl$TraversalRunnable.run(ViewRootImpl.java:5885) at android.view.Choreographer$CallbackRecord.run(Choreographer.java:767) at android.view.Choreographer.doCallbacks(Choreographer.java:580) at android.view.Choreographer.doFrame(Choreographer.java:550) at android.view.Choreographer$FrameDisplayEventReceiver.run(Choreographer.java:753) at android.os.Handler.handleCallback(Handler.java:739) at android.os.Handler.dispatchMessage(Handler.java:95) at android.os.Looper.loop(Looper.java:135) at android.app.ActivityThread.main(ActivityThread.java:5254) at java.lang.reflect.Method.invoke(Native Method) at java.lang.reflect.Method.invoke(Method.java:372) at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:903) at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:698)
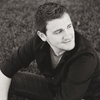
Zachary Zell
4,747 Pointspackage com.example.zachary.ribbitnew;
import android.app.Fragment; import android.app.FragmentManager; import android.content.Context; import android.support.v13.app.FragmentPagerAdapter;
import java.util.Locale;
/**
-
Created by Zachary on 4/9/2015. */ public class SectionsPagerAdapter extends FragmentPagerAdapter {
protected Context mContext;
public SectionsPagerAdapter(Context context, FragmentManager fm) { super(fm); mContext = context; }
@Override public Fragment getItem(int position) { // getItem is called to instantiate the fragment for the given page. // Return a PlaceholderFragment (defined as a static inner class below).
switch (position) { case 0: return new InboxFragment(); case 1: return new FriendsFragment(); } return null;
}
@Override public int getCount() { // Show 2 total pages. return 2; }
@Override public CharSequence getPageTitle(int position) { Locale l = Locale.getDefault(); switch (position) { case 0: return mContext.getString(R.string.title_section1).toUpperCase(l); case 1: return mContext.getString(R.string.title_section2).toUpperCase(l); } return null; } }
2 Answers
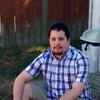
alex gwartney
8,849 Pointsi had the same issue i fixed it by going into the xml file of the list view make sure you have your id as
@android:id/list
see if that works

Miguel Fernandes
1,314 Pointsthanks! solved my problem!
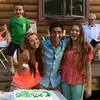
David Axelrod
36,073 PointsMy app closes automatically too :(
Zachary Zell
4,747 PointsZachary Zell
4,747 Pointspackage com.example.zachary.ribbitnew;
import java.util.Locale;
import android.app.Activity; import android.app.ActionBar; import android.app.Fragment; import android.app.FragmentManager; import android.app.FragmentTransaction; import android.content.Intent; import android.nfc.Tag; import android.support.v13.app.FragmentPagerAdapter; import android.os.Bundle; import android.support.v4.app.FragmentActivity; import android.support.v4.view.ViewPager; import android.util.Log; import android.view.Gravity; import android.view.LayoutInflater; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.view.ViewGroup; import android.widget.TextView;
import com.parse.ParseUser;
public class MainActivity extends FragmentActivity implements ActionBar.TabListener {
}