Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial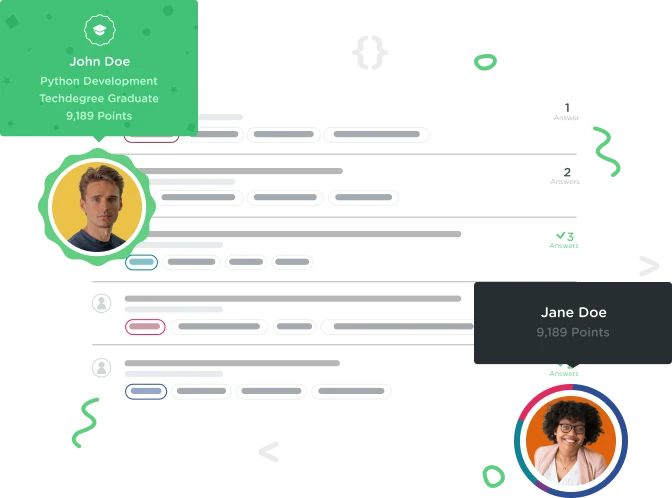
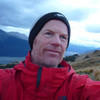
darrell mayson
5,215 PointsError thrown: "in `print_list': undefined method `each' for nil:NilClass (NoMethodError)"
Immediately after I enter the quantity the programme throws this error after printing to the console the "----" under the list name. I've Googled it to death and updated my version of Ruby to ruby 1.9.3p484 (2013-11-22 revision 43786) [i686-linux]. Cannot resolve. Any help appreciated!
Code snippet... def print_list(list) puts "List: #{list['name']}" puts "----"
list["items"].each do |item| puts "Item: " + item['name'] puts "Quantity: " + item['quantity'].to_s puts "---" end end

David O' Rojo
11,051 PointsNote: Moved this as a comment to your question as it is not the answer.
Checking your code, your function works just fine, so maybe your problem is at the definition of the collection:
def print_list(list)
puts "List: #{list['name']}"
puts "----"
list["items"].each do |item|
puts "Item: " + item['name']
puts "Quantity: " + item['quantity'].to_s
puts "---"
end
end
grocery_list = {
'name' => 'groceries',
'items' => [
{ 'name' => 'milk', 'quantity' => 6 },
{ 'name' => 'eggs', 'quantity' => 12 },
]
}
print_list(grocery_list)
# List: groceries
# ----
# Item: milk
# Quantity: 6
# ---
# Item: eggs
# Quantity: 12
# ---
2 Answers

David O' Rojo
11,051 PointsNo offence intended, but you need to learn to present your code in a way that is easy to read, as it will help you and other programmers that read and debug the code. With Markdown is pretty easy to show coloured code in this forums.
Here is what your code looks like:
def create_list
print "What is the list named? "
name = gets.chomp
hash = { "name" => name, "items" => Array.new }
return hash
end
def add_list_item
print "What is the item called? "
item_name = gets.chomp
print "How many of item? "
qty = gets.chomp.to_i
hash = { "name" => item_name, "qty" => qty } # first error
return hash
end
def print_list(list)
puts "List: #{list['name']}"
puts "----"
list["items"].each do |item|
puts "Item: " + item['name']
puts "Quantity: " + item['quantity'].to_s # first error
puts "---"
end
end
def print_list(list)
puts "List: #{list['name']}"
puts "----"
list["items"].each do |item|
puts "Item: " + item['name']
puts "Quantity: " + item['quantity'].to_s
puts "---"
end
end
list = create_list()
puts list.inspect
list['items'].push(add_list_item())
puts list.inspect
print_list('list') # second error
You have two errors there:
- At
add_list_item
you're defining a key with the name of qty while inprint_list
you're referencing the same key as quantity. - When calling
print_list
at the end of the code, you're passing in a String instead of a Hash.
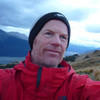
darrell mayson
5,215 PointsThanks for your reply David. Yeah probably should have included full listing. Sorry it's not formatted but TBH learning a new syntax to post code for a language I'm learning may break me ><
def create_list print "What is the list named? " name = gets.chomp #chomp removes trailing white space hash = {"name" => name, "items" => Array.new} #eg., name='Groceries', items = [] return hash end
def add_list_item #returns: key/value hash
# what will the item appear as in your list?
print "What is the item called? "
item_name = gets.chomp
# what quantity of 'item' do you want?
print "How many of item? "
qty = gets.chomp.to_i # convert input from string to integer
hash = {"name" => item_name, "qty" => qty}
return hash
end
def print_list(list) puts "List: #{list['name']}" puts "----" list["items"].each do |item| puts "Item: " + item['name'] puts "Quantity: " + item['quantity'].to_s puts "---" end end
list = create_list() puts list.inspect list['items'].push(add_list_item()) # use 'push' method to insert new value into 'items' array.
puts list.inspect print_list('list')
David O' Rojo
11,051 PointsDavid O' Rojo
11,051 PointsJust to clarify your code:
You can use Markdown to give format to snippets.