Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial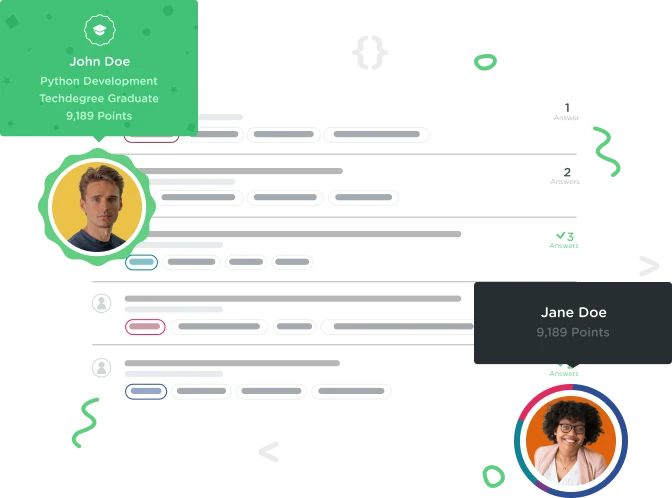
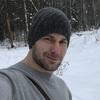
gennady
14,145 PointsError 'Uncaught TypeError: Cannot set property 'color' of undefined' for loop. What is the problem?
Hi, I got the error, could you help me understand why this appears? Here is my code:
const myHeading = document.getElementById('myHeading');
const myInput = document.getElementById('myInput');
const myButton = document.getElementById('myButton');
const myResetButton = document.getElementById('myResetButton');
const myCheckBox = document.getElementById('myCheckBox');
const myList = document.getElementsByTagName('li');
myButton.addEventListener( 'click', () => myHeading.style.color = myInput.value );
myResetButton.addEventListener('click', () => myHeading.style.color = 'black' );
myCheckBox.addEventListener( 'change', () => {
myHeading.style.border = 'lightblue solid 2px';
myHeading.style.borderRadius = '25px';
} );
for (let i in myList) { myList[i].style.color = 'purple'; } //ERROR FOR THIS STRING: 'Uncaught TypeError: Cannot set property 'color' of undefined'.
Meanwhile colours for the list change successfully but later code doesn't work.
Thank you!
1 Answer
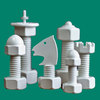
Steven Parker
229,732 PointsGenerally for...in is not a good choice for iterating an array or collection.
In addition to the items with numbered indexes, in will also return items like "length". Trying to access the style property on such an item will return null.
Use a traditional for loop with a numeric index instead.
gennady
14,145 Pointsgennady
14,145 PointsThank you Steven! I read this from MDN:
'Although it may be tempting to use this as a way to iterate over Array elements, the for...in statement will return the name of your user-defined properties in addition to the numeric indexes. Thus it is better to use a traditional for loop with a numeric index when iterating over arrays, because the for...in statement iterates over user-defined properties in addition to the array elements, if you modify the Array object, such as adding custom properties or methods'
Now I understand that in general it's better not to use 'for in loop' for Arrays. BUT for the case I didin't add custom properties or methods. The code is very simple, so what is the problem? Btw Object also has 'length' property, but for objects 'for in' works fine. Really confused about this...
Steven Parker
229,732 PointsSteven Parker
229,732 PointsUnlike a simple array, an HTMLCollection (as returned by getElementsByTagName) will have a "length" property, and "item" and "namedItem" methods by default. And these will be part of a for...in iteration even if you have not added any custom items.