Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial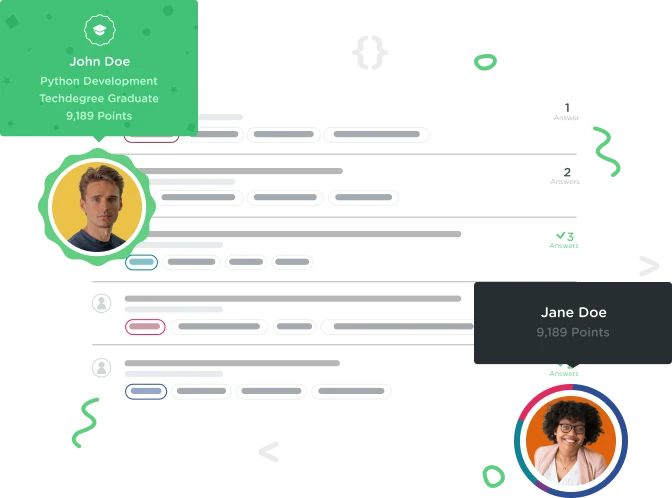

Tijo Thomas
9,737 PointsError: Undefined local variable or method 'index'
I'm getting this error when I run my code which is below. The question I have is why doesn't the code in the video run into this same error as index in the 'remove_item' method of the video is not defined either.
require "./todo_item.rb"
class TodoList
attr_reader :name, :todo_items
def initialize(name)
@name = name
@todo_items = []
end
# creates new item by instantiating ToDoItem class and them added it to the todo_items array.
def add_item(name)
todo_items.push(TodoItem.new(name))
end
def remove_item(name)
if index == find_index(name)
todo_items.delete_at(index)
return true
else
return false
end
end
def mark_complete(name)
end
def find_index(name)
index = 0
found = false
todo_items.each do |todo_item|
if todo_item.name == name
found = true
return index
break
else
index += 1
return nil
end
end
end
end
todo_list = TodoList.new("Groceries")
todo_list.add_item("Milk")
todo_list.add_item("Eggs")
puts todo_list.inspect
if todo_list.remove_item("Eggs")
puts "Eggs were removed from the list."
end
puts todo_list.inspect
2 Answers
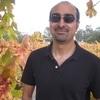
Kourosh Raeen
23,733 PointsIf you use == then you are comparing what the find_index() method returns with the value of index. But there is no local variable index in remove_item() and there is also no method called index that returns anything so it's impossible to make a comparison. However, with an assignment, =, you are assigning the return value of find_index() to index. This return value is either a number, which then ruby interprets it as true even if it is 0, or it is false. So in the end the if statement ends up working as expected:
if true
some code to run
else
some other code to run
end
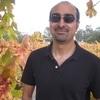
Kourosh Raeen
23,733 PointsIn the remove_item() method the line:
if index == find_index(name)
should be:
if index = find_index(name)
Also, there is a logical problem with your find_index() method. In the else part you add one to index but then you return nil, so the loop will never go past the first item.

Tijo Thomas
9,737 PointsThe only thing I don't understand is how does the if statement work with only 1 equals sign?